mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 17:30:02 +00:00
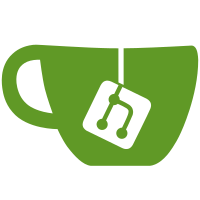
-----BEGIN PGP SIGNATURE----- iQJEBAABCAAuFiEEwPw5LcreJtl1+l5K99NY+ylx4KYFAmHd8DAQHGF4Ym9lQGtl cm5lbC5kawAKCRD301j7KXHgpnhRD/wMAjsNO65PCA+o/bPpVi4ulx9EejAzrJnB 5vHFvREAoOOGKvRpYGe4w3TcKyW+zPb+GtlXFjPfK+wuVzWhrQtW/+vkjKlBt8wK o7rzeMwTKJ9ZGvYaaQpp1yC0WURBB3qnCRQhb8dOQzhJgEXinhIOznZsut4mniLv fTqcDmKAb/+G6K6CQCCqnH0I/+OJZyUeSFo1kk2i4ZqCBepQpBkOL6H2rBOtGxUg bt1jiGHbbhCRYEE3u2kV0HP10qAChNaMQC705jV4Qpf4+3EntSxs+6nSb74dvMkX 3+Wmp8Ctq6lpPnDL1nrAFGz3jZnB0Y+GdgOclQn3ViQd1FCXZzuYWQ3fTaBfURCZ /RE5nc047SqpwCFLOynM++OkaeQZ1zSxeyoFTtzDaPF4tLuaX3JHswvTzNGPw8SN BnexseNnNBCjJliZSEE7fOkjJDcev2dvRxPtI8/wkF4lHUgETc5IW563C53xo/Tx 32yFjZwCVIpNWk21su/0H3iEq80wZ7PnriiN/E3JA6XbnevlRPu0NPMb0D258GCm yCcdPVDNZsQCB8hluqZcu0g6LSgZRo90Yg1oqKqEpAllJJMBaEAPPPuUIJh998mo iKGxZzgr7d9jrbGJTInp0F8b3B3/oV/hxgzy0Hu/mHP3AsnaAk9o/oEQZ7rX4Khr 6biloqkIMA== =RWnJ -----END PGP SIGNATURE----- Merge tag 'for-5.17/block-2022-01-11' of git://git.kernel.dk/linux-block Pull block updates from Jens Axboe: - Unify where the struct request handling code is located in the blk-mq code (Christoph) - Header cleanups (Christoph) - Clean up the io_context handling code (Christoph, me) - Get rid of ->rq_disk in struct request (Christoph) - Error handling fix for add_disk() (Christoph) - request allocation cleanusp (Christoph) - Documentation updates (Eric, Matthew) - Remove trivial crypto unregister helper (Eric) - Reduce shared tag overhead (John) - Reduce poll_stats memory overhead (me) - Known indirect function call for dio (me) - Use atomic references for struct request (me) - Support request list issue for block and NVMe (me) - Improve queue dispatch pinning (Ming) - Improve the direct list issue code (Keith) - BFQ improvements (Jan) - Direct completion helper and use it in mmc block (Sebastian) - Use raw spinlock for the blktrace code (Wander) - fsync error handling fix (Ye) - Various fixes and cleanups (Lukas, Randy, Yang, Tetsuo, Ming, me) * tag 'for-5.17/block-2022-01-11' of git://git.kernel.dk/linux-block: (132 commits) MAINTAINERS: add entries for block layer documentation docs: block: remove queue-sysfs.rst docs: sysfs-block: document virt_boundary_mask docs: sysfs-block: document stable_writes docs: sysfs-block: fill in missing documentation from queue-sysfs.rst docs: sysfs-block: add contact for nomerges docs: sysfs-block: sort alphabetically docs: sysfs-block: move to stable directory block: don't protect submit_bio_checks by q_usage_counter block: fix old-style declaration nvme-pci: fix queue_rqs list splitting block: introduce rq_list_move block: introduce rq_list_for_each_safe macro block: move rq_list macros to blk-mq.h block: drop needless assignment in set_task_ioprio() block: remove unnecessary trailing '\' bio.h: fix kernel-doc warnings block: check minor range in device_add_disk() block: use "unsigned long" for blk_validate_block_size(). block: fix error unwinding in device_add_disk ...
236 lines
4.8 KiB
C
236 lines
4.8 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* fs/ioprio.c
|
|
*
|
|
* Copyright (C) 2004 Jens Axboe <axboe@kernel.dk>
|
|
*
|
|
* Helper functions for setting/querying io priorities of processes. The
|
|
* system calls closely mimmick getpriority/setpriority, see the man page for
|
|
* those. The prio argument is a composite of prio class and prio data, where
|
|
* the data argument has meaning within that class. The standard scheduling
|
|
* classes have 8 distinct prio levels, with 0 being the highest prio and 7
|
|
* being the lowest.
|
|
*
|
|
* IOW, setting BE scheduling class with prio 2 is done ala:
|
|
*
|
|
* unsigned int prio = (IOPRIO_CLASS_BE << IOPRIO_CLASS_SHIFT) | 2;
|
|
*
|
|
* ioprio_set(PRIO_PROCESS, pid, prio);
|
|
*
|
|
* See also Documentation/block/ioprio.rst
|
|
*
|
|
*/
|
|
#include <linux/gfp.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/ioprio.h>
|
|
#include <linux/cred.h>
|
|
#include <linux/blkdev.h>
|
|
#include <linux/capability.h>
|
|
#include <linux/syscalls.h>
|
|
#include <linux/security.h>
|
|
#include <linux/pid_namespace.h>
|
|
|
|
int ioprio_check_cap(int ioprio)
|
|
{
|
|
int class = IOPRIO_PRIO_CLASS(ioprio);
|
|
int data = IOPRIO_PRIO_DATA(ioprio);
|
|
|
|
switch (class) {
|
|
case IOPRIO_CLASS_RT:
|
|
/*
|
|
* Originally this only checked for CAP_SYS_ADMIN,
|
|
* which was implicitly allowed for pid 0 by security
|
|
* modules such as SELinux. Make sure we check
|
|
* CAP_SYS_ADMIN first to avoid a denial/avc for
|
|
* possibly missing CAP_SYS_NICE permission.
|
|
*/
|
|
if (!capable(CAP_SYS_ADMIN) && !capable(CAP_SYS_NICE))
|
|
return -EPERM;
|
|
fallthrough;
|
|
/* rt has prio field too */
|
|
case IOPRIO_CLASS_BE:
|
|
if (data >= IOPRIO_NR_LEVELS || data < 0)
|
|
return -EINVAL;
|
|
break;
|
|
case IOPRIO_CLASS_IDLE:
|
|
break;
|
|
case IOPRIO_CLASS_NONE:
|
|
if (data)
|
|
return -EINVAL;
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
SYSCALL_DEFINE3(ioprio_set, int, which, int, who, int, ioprio)
|
|
{
|
|
struct task_struct *p, *g;
|
|
struct user_struct *user;
|
|
struct pid *pgrp;
|
|
kuid_t uid;
|
|
int ret;
|
|
|
|
ret = ioprio_check_cap(ioprio);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = -ESRCH;
|
|
rcu_read_lock();
|
|
switch (which) {
|
|
case IOPRIO_WHO_PROCESS:
|
|
if (!who)
|
|
p = current;
|
|
else
|
|
p = find_task_by_vpid(who);
|
|
if (p)
|
|
ret = set_task_ioprio(p, ioprio);
|
|
break;
|
|
case IOPRIO_WHO_PGRP:
|
|
if (!who)
|
|
pgrp = task_pgrp(current);
|
|
else
|
|
pgrp = find_vpid(who);
|
|
|
|
read_lock(&tasklist_lock);
|
|
do_each_pid_thread(pgrp, PIDTYPE_PGID, p) {
|
|
ret = set_task_ioprio(p, ioprio);
|
|
if (ret) {
|
|
read_unlock(&tasklist_lock);
|
|
goto out;
|
|
}
|
|
} while_each_pid_thread(pgrp, PIDTYPE_PGID, p);
|
|
read_unlock(&tasklist_lock);
|
|
|
|
break;
|
|
case IOPRIO_WHO_USER:
|
|
uid = make_kuid(current_user_ns(), who);
|
|
if (!uid_valid(uid))
|
|
break;
|
|
if (!who)
|
|
user = current_user();
|
|
else
|
|
user = find_user(uid);
|
|
|
|
if (!user)
|
|
break;
|
|
|
|
for_each_process_thread(g, p) {
|
|
if (!uid_eq(task_uid(p), uid) ||
|
|
!task_pid_vnr(p))
|
|
continue;
|
|
ret = set_task_ioprio(p, ioprio);
|
|
if (ret)
|
|
goto free_uid;
|
|
}
|
|
free_uid:
|
|
if (who)
|
|
free_uid(user);
|
|
break;
|
|
default:
|
|
ret = -EINVAL;
|
|
}
|
|
|
|
out:
|
|
rcu_read_unlock();
|
|
return ret;
|
|
}
|
|
|
|
static int get_task_ioprio(struct task_struct *p)
|
|
{
|
|
int ret;
|
|
|
|
ret = security_task_getioprio(p);
|
|
if (ret)
|
|
goto out;
|
|
ret = IOPRIO_DEFAULT;
|
|
task_lock(p);
|
|
if (p->io_context)
|
|
ret = p->io_context->ioprio;
|
|
task_unlock(p);
|
|
out:
|
|
return ret;
|
|
}
|
|
|
|
int ioprio_best(unsigned short aprio, unsigned short bprio)
|
|
{
|
|
if (!ioprio_valid(aprio))
|
|
aprio = IOPRIO_DEFAULT;
|
|
if (!ioprio_valid(bprio))
|
|
bprio = IOPRIO_DEFAULT;
|
|
|
|
return min(aprio, bprio);
|
|
}
|
|
|
|
SYSCALL_DEFINE2(ioprio_get, int, which, int, who)
|
|
{
|
|
struct task_struct *g, *p;
|
|
struct user_struct *user;
|
|
struct pid *pgrp;
|
|
kuid_t uid;
|
|
int ret = -ESRCH;
|
|
int tmpio;
|
|
|
|
rcu_read_lock();
|
|
switch (which) {
|
|
case IOPRIO_WHO_PROCESS:
|
|
if (!who)
|
|
p = current;
|
|
else
|
|
p = find_task_by_vpid(who);
|
|
if (p)
|
|
ret = get_task_ioprio(p);
|
|
break;
|
|
case IOPRIO_WHO_PGRP:
|
|
if (!who)
|
|
pgrp = task_pgrp(current);
|
|
else
|
|
pgrp = find_vpid(who);
|
|
read_lock(&tasklist_lock);
|
|
do_each_pid_thread(pgrp, PIDTYPE_PGID, p) {
|
|
tmpio = get_task_ioprio(p);
|
|
if (tmpio < 0)
|
|
continue;
|
|
if (ret == -ESRCH)
|
|
ret = tmpio;
|
|
else
|
|
ret = ioprio_best(ret, tmpio);
|
|
} while_each_pid_thread(pgrp, PIDTYPE_PGID, p);
|
|
read_unlock(&tasklist_lock);
|
|
|
|
break;
|
|
case IOPRIO_WHO_USER:
|
|
uid = make_kuid(current_user_ns(), who);
|
|
if (!who)
|
|
user = current_user();
|
|
else
|
|
user = find_user(uid);
|
|
|
|
if (!user)
|
|
break;
|
|
|
|
for_each_process_thread(g, p) {
|
|
if (!uid_eq(task_uid(p), user->uid) ||
|
|
!task_pid_vnr(p))
|
|
continue;
|
|
tmpio = get_task_ioprio(p);
|
|
if (tmpio < 0)
|
|
continue;
|
|
if (ret == -ESRCH)
|
|
ret = tmpio;
|
|
else
|
|
ret = ioprio_best(ret, tmpio);
|
|
}
|
|
|
|
if (who)
|
|
free_uid(user);
|
|
break;
|
|
default:
|
|
ret = -EINVAL;
|
|
}
|
|
|
|
rcu_read_unlock();
|
|
return ret;
|
|
}
|