mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
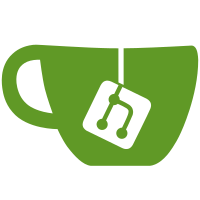
__aio_put_req() plays sick games with file refcount. What it wants is fput() from atomic context; it's almost always done with f_count > 1, so they only have to deal with delayed work in rare cases when their reference happens to be the last one. Current code decrements f_count and if it hasn't hit 0, everything is fine. Otherwise it keeps a pointer to struct file (with zero f_count!) around and has delayed work do __fput() on it. Better way to do it: use atomic_long_add_unless( , -1, 1) instead of !atomic_long_dec_and_test(). IOW, decrement it only if it's not the last reference, leave refcount alone if it was. And use normal fput() in delayed work. I've made that atomic_long_add_unless call a new helper - fput_atomic(). Drops a reference to file if it's safe to do in atomic (i.e. if that's not the last one), tells if it had been able to do that. aio.c converted to it, __fput() use is gone. req->ki_file *always* contributes to refcount now. And __fput() became static. Signed-off-by: Al Viro <viro@zeniv.linux.org.uk>
41 lines
1 KiB
C
41 lines
1 KiB
C
/*
|
|
* Wrapper functions for accessing the file_struct fd array.
|
|
*/
|
|
|
|
#ifndef __LINUX_FILE_H
|
|
#define __LINUX_FILE_H
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/types.h>
|
|
#include <linux/posix_types.h>
|
|
|
|
struct file;
|
|
|
|
extern void fput(struct file *);
|
|
extern void drop_file_write_access(struct file *file);
|
|
|
|
struct file_operations;
|
|
struct vfsmount;
|
|
struct dentry;
|
|
struct path;
|
|
extern struct file *alloc_file(struct path *, fmode_t mode,
|
|
const struct file_operations *fop);
|
|
|
|
static inline void fput_light(struct file *file, int fput_needed)
|
|
{
|
|
if (unlikely(fput_needed))
|
|
fput(file);
|
|
}
|
|
|
|
extern struct file *fget(unsigned int fd);
|
|
extern struct file *fget_light(unsigned int fd, int *fput_needed);
|
|
extern void set_close_on_exec(unsigned int fd, int flag);
|
|
extern void put_filp(struct file *);
|
|
extern int alloc_fd(unsigned start, unsigned flags);
|
|
extern int get_unused_fd(void);
|
|
#define get_unused_fd_flags(flags) alloc_fd(0, (flags))
|
|
extern void put_unused_fd(unsigned int fd);
|
|
|
|
extern void fd_install(unsigned int fd, struct file *file);
|
|
|
|
#endif /* __LINUX_FILE_H */
|