mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 23:24:50 +00:00
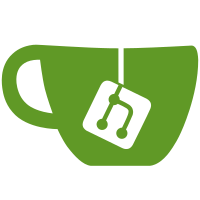
Merge yet more updates from Andrew Morton: - More MM work. 100ish more to go. Mike Rapoport's "mm: remove __ARCH_HAS_5LEVEL_HACK" series should fix the current ppc issue - Various other little subsystems * emailed patches from Andrew Morton <akpm@linux-foundation.org>: (127 commits) lib/ubsan.c: fix gcc-10 warnings tools/testing/selftests/vm: remove duplicate headers selftests: vm: pkeys: fix multilib builds for x86 selftests: vm: pkeys: use the correct page size on powerpc selftests/vm/pkeys: override access right definitions on powerpc selftests/vm/pkeys: test correct behaviour of pkey-0 selftests/vm/pkeys: introduce a sub-page allocator selftests/vm/pkeys: detect write violation on a mapped access-denied-key page selftests/vm/pkeys: associate key on a mapped page and detect write violation selftests/vm/pkeys: associate key on a mapped page and detect access violation selftests/vm/pkeys: improve checks to determine pkey support selftests/vm/pkeys: fix assertion in test_pkey_alloc_exhaust() selftests/vm/pkeys: fix number of reserved powerpc pkeys selftests/vm/pkeys: introduce powerpc support selftests/vm/pkeys: introduce generic pkey abstractions selftests: vm: pkeys: use the correct huge page size selftests/vm/pkeys: fix alloc_random_pkey() to make it really random selftests/vm/pkeys: fix assertion in pkey_disable_set/clear() selftests/vm/pkeys: fix pkey_disable_clear() selftests: vm: pkeys: add helpers for pkey bits ...
110 lines
2.7 KiB
C
110 lines
2.7 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* linux/fs/binfmt_em86.c
|
|
*
|
|
* Based on linux/fs/binfmt_script.c
|
|
* Copyright (C) 1996 Martin von Löwis
|
|
* original #!-checking implemented by tytso.
|
|
*
|
|
* em86 changes Copyright (C) 1997 Jim Paradis
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/string.h>
|
|
#include <linux/stat.h>
|
|
#include <linux/binfmts.h>
|
|
#include <linux/elf.h>
|
|
#include <linux/init.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/file.h>
|
|
#include <linux/errno.h>
|
|
|
|
|
|
#define EM86_INTERP "/usr/bin/em86"
|
|
#define EM86_I_NAME "em86"
|
|
|
|
static int load_em86(struct linux_binprm *bprm)
|
|
{
|
|
const char *i_name, *i_arg;
|
|
char *interp;
|
|
struct file * file;
|
|
int retval;
|
|
struct elfhdr elf_ex;
|
|
|
|
/* Make sure this is a Linux/Intel ELF executable... */
|
|
elf_ex = *((struct elfhdr *)bprm->buf);
|
|
|
|
if (memcmp(elf_ex.e_ident, ELFMAG, SELFMAG) != 0)
|
|
return -ENOEXEC;
|
|
|
|
/* First of all, some simple consistency checks */
|
|
if ((elf_ex.e_type != ET_EXEC && elf_ex.e_type != ET_DYN) ||
|
|
(!((elf_ex.e_machine == EM_386) || (elf_ex.e_machine == EM_486))) ||
|
|
!bprm->file->f_op->mmap) {
|
|
return -ENOEXEC;
|
|
}
|
|
|
|
/* Need to be able to load the file after exec */
|
|
if (bprm->interp_flags & BINPRM_FLAGS_PATH_INACCESSIBLE)
|
|
return -ENOENT;
|
|
|
|
/* Unlike in the script case, we don't have to do any hairy
|
|
* parsing to find our interpreter... it's hardcoded!
|
|
*/
|
|
interp = EM86_INTERP;
|
|
i_name = EM86_I_NAME;
|
|
i_arg = NULL; /* We reserve the right to add an arg later */
|
|
|
|
/*
|
|
* Splice in (1) the interpreter's name for argv[0]
|
|
* (2) (optional) argument to interpreter
|
|
* (3) filename of emulated file (replace argv[0])
|
|
*
|
|
* This is done in reverse order, because of how the
|
|
* user environment and arguments are stored.
|
|
*/
|
|
remove_arg_zero(bprm);
|
|
retval = copy_string_kernel(bprm->filename, bprm);
|
|
if (retval < 0) return retval;
|
|
bprm->argc++;
|
|
if (i_arg) {
|
|
retval = copy_string_kernel(i_arg, bprm);
|
|
if (retval < 0) return retval;
|
|
bprm->argc++;
|
|
}
|
|
retval = copy_string_kernel(i_name, bprm);
|
|
if (retval < 0) return retval;
|
|
bprm->argc++;
|
|
|
|
/*
|
|
* OK, now restart the process with the interpreter's inode.
|
|
* Note that we use open_exec() as the name is now in kernel
|
|
* space, and we don't need to copy it.
|
|
*/
|
|
file = open_exec(interp);
|
|
if (IS_ERR(file))
|
|
return PTR_ERR(file);
|
|
|
|
bprm->interpreter = file;
|
|
return 0;
|
|
}
|
|
|
|
static struct linux_binfmt em86_format = {
|
|
.module = THIS_MODULE,
|
|
.load_binary = load_em86,
|
|
};
|
|
|
|
static int __init init_em86_binfmt(void)
|
|
{
|
|
register_binfmt(&em86_format);
|
|
return 0;
|
|
}
|
|
|
|
static void __exit exit_em86_binfmt(void)
|
|
{
|
|
unregister_binfmt(&em86_format);
|
|
}
|
|
|
|
core_initcall(init_em86_binfmt);
|
|
module_exit(exit_em86_binfmt);
|
|
MODULE_LICENSE("GPL");
|