mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 15:20:41 +00:00
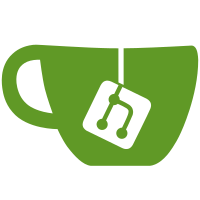
The simple display pipeline is a set of helpers that can be used by DRM drivers to avoid dealing with all the needed components and just define a few functions to operate a simple display device with one full-screen scanout buffer feeding a single output. But it is arguable that this provides the correct level of abstraction for simple drivers, and recently some have been ported from using these simple display helpers to use the regular atomic helpers instead. The rationale for this is that the simple display pipeline helpers don't hide that much of the DRM complexity, while adding an indirection layer that conflates the concepts of CRTCs and planes. This makes the helpers less flexible and harder to be reused among different graphics drivers. Also, for simple drivers, using the full atomic helpers doesn't require a lot of additional code. So adding a simple display pipeline layer may not be worth it. For these reasons, let's follow that trend and make ssd130x a plain DRM driver that creates its own primary plane, CRTC, enconder and connector. Suggested-by: Thomas Zimmermann <tzimmermann@suse.de> Signed-off-by: Javier Martinez Canillas <javierm@redhat.com> Acked-by: Thomas Zimmermann <tzimmermann@suse.de> Link: https://patchwork.freedesktop.org/patch/msgid/20220905222759.2597186-1-javierm@redhat.com
97 lines
2 KiB
C
97 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Header file for:
|
|
* DRM driver for Solomon SSD130x OLED displays
|
|
*
|
|
* Copyright 2022 Red Hat Inc.
|
|
* Author: Javier Martinez Canillas <javierm@redhat.com>
|
|
*
|
|
* Based on drivers/video/fbdev/ssd1307fb.c
|
|
* Copyright 2012 Free Electrons
|
|
*/
|
|
|
|
#ifndef __SSD1307X_H__
|
|
#define __SSD1307X_H__
|
|
|
|
#include <drm/drm_connector.h>
|
|
#include <drm/drm_crtc.h>
|
|
#include <drm/drm_drv.h>
|
|
#include <drm/drm_encoder.h>
|
|
#include <drm/drm_plane_helper.h>
|
|
|
|
#include <linux/regmap.h>
|
|
|
|
#define SSD130X_DATA 0x40
|
|
#define SSD130X_COMMAND 0x80
|
|
|
|
enum ssd130x_variants {
|
|
SH1106_ID,
|
|
SSD1305_ID,
|
|
SSD1306_ID,
|
|
SSD1307_ID,
|
|
SSD1309_ID,
|
|
NR_SSD130X_VARIANTS
|
|
};
|
|
|
|
struct ssd130x_deviceinfo {
|
|
u32 default_vcomh;
|
|
u32 default_dclk_div;
|
|
u32 default_dclk_frq;
|
|
int need_pwm;
|
|
int need_chargepump;
|
|
bool page_mode_only;
|
|
};
|
|
|
|
struct ssd130x_device {
|
|
struct drm_device drm;
|
|
struct device *dev;
|
|
struct drm_display_mode mode;
|
|
struct drm_plane primary_plane;
|
|
struct drm_crtc crtc;
|
|
struct drm_encoder encoder;
|
|
struct drm_connector connector;
|
|
struct i2c_client *client;
|
|
|
|
struct regmap *regmap;
|
|
|
|
const struct ssd130x_deviceinfo *device_info;
|
|
|
|
unsigned page_address_mode : 1;
|
|
unsigned area_color_enable : 1;
|
|
unsigned com_invdir : 1;
|
|
unsigned com_lrremap : 1;
|
|
unsigned com_seq : 1;
|
|
unsigned lookup_table_set : 1;
|
|
unsigned low_power : 1;
|
|
unsigned seg_remap : 1;
|
|
u32 com_offset;
|
|
u32 contrast;
|
|
u32 dclk_div;
|
|
u32 dclk_frq;
|
|
u32 height;
|
|
u8 lookup_table[4];
|
|
u32 page_offset;
|
|
u32 col_offset;
|
|
u32 prechargep1;
|
|
u32 prechargep2;
|
|
|
|
struct backlight_device *bl_dev;
|
|
struct pwm_device *pwm;
|
|
struct gpio_desc *reset;
|
|
struct regulator *vcc_reg;
|
|
u32 vcomh;
|
|
u32 width;
|
|
/* Cached address ranges */
|
|
u8 col_start;
|
|
u8 col_end;
|
|
u8 page_start;
|
|
u8 page_end;
|
|
};
|
|
|
|
extern const struct ssd130x_deviceinfo ssd130x_variants[];
|
|
|
|
struct ssd130x_device *ssd130x_probe(struct device *dev, struct regmap *regmap);
|
|
void ssd130x_remove(struct ssd130x_device *ssd130x);
|
|
void ssd130x_shutdown(struct ssd130x_device *ssd130x);
|
|
|
|
#endif /* __SSD1307X_H__ */
|