mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
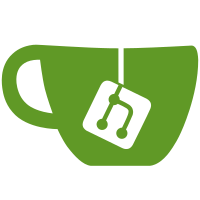
The struct firmware contains a page table pointer that was used only internally in the past. Since the actual page tables are referred from struct fw_priv and should be never from struct firmware, we can drop this unused field gracefully. Signed-off-by: Takashi Iwai <tiwai@suse.de> Acked-by: Luis Chamberlain <mcgrof@kernel.org> Link: https://lore.kernel.org/r/20200415164500.28749-1-tiwai@suse.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
109 lines
2.9 KiB
C
109 lines
2.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_FIRMWARE_H
|
|
#define _LINUX_FIRMWARE_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/compiler.h>
|
|
#include <linux/gfp.h>
|
|
|
|
#define FW_ACTION_NOHOTPLUG 0
|
|
#define FW_ACTION_HOTPLUG 1
|
|
|
|
struct firmware {
|
|
size_t size;
|
|
const u8 *data;
|
|
|
|
/* firmware loader private fields */
|
|
void *priv;
|
|
};
|
|
|
|
struct module;
|
|
struct device;
|
|
|
|
struct builtin_fw {
|
|
char *name;
|
|
void *data;
|
|
unsigned long size;
|
|
};
|
|
|
|
/* We have to play tricks here much like stringify() to get the
|
|
__COUNTER__ macro to be expanded as we want it */
|
|
#define __fw_concat1(x, y) x##y
|
|
#define __fw_concat(x, y) __fw_concat1(x, y)
|
|
|
|
#define DECLARE_BUILTIN_FIRMWARE(name, blob) \
|
|
DECLARE_BUILTIN_FIRMWARE_SIZE(name, &(blob), sizeof(blob))
|
|
|
|
#define DECLARE_BUILTIN_FIRMWARE_SIZE(name, blob, size) \
|
|
static const struct builtin_fw __fw_concat(__builtin_fw,__COUNTER__) \
|
|
__used __section(.builtin_fw) = { name, blob, size }
|
|
|
|
#if defined(CONFIG_FW_LOADER) || (defined(CONFIG_FW_LOADER_MODULE) && defined(MODULE))
|
|
int request_firmware(const struct firmware **fw, const char *name,
|
|
struct device *device);
|
|
int firmware_request_nowarn(const struct firmware **fw, const char *name,
|
|
struct device *device);
|
|
int firmware_request_platform(const struct firmware **fw, const char *name,
|
|
struct device *device);
|
|
int request_firmware_nowait(
|
|
struct module *module, bool uevent,
|
|
const char *name, struct device *device, gfp_t gfp, void *context,
|
|
void (*cont)(const struct firmware *fw, void *context));
|
|
int request_firmware_direct(const struct firmware **fw, const char *name,
|
|
struct device *device);
|
|
int request_firmware_into_buf(const struct firmware **firmware_p,
|
|
const char *name, struct device *device, void *buf, size_t size);
|
|
|
|
void release_firmware(const struct firmware *fw);
|
|
#else
|
|
static inline int request_firmware(const struct firmware **fw,
|
|
const char *name,
|
|
struct device *device)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int firmware_request_nowarn(const struct firmware **fw,
|
|
const char *name,
|
|
struct device *device)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int firmware_request_platform(const struct firmware **fw,
|
|
const char *name,
|
|
struct device *device)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int request_firmware_nowait(
|
|
struct module *module, bool uevent,
|
|
const char *name, struct device *device, gfp_t gfp, void *context,
|
|
void (*cont)(const struct firmware *fw, void *context))
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline void release_firmware(const struct firmware *fw)
|
|
{
|
|
}
|
|
|
|
static inline int request_firmware_direct(const struct firmware **fw,
|
|
const char *name,
|
|
struct device *device)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int request_firmware_into_buf(const struct firmware **firmware_p,
|
|
const char *name, struct device *device, void *buf, size_t size)
|
|
{
|
|
return -EINVAL;
|
|
}
|
|
|
|
#endif
|
|
|
|
int firmware_request_cache(struct device *device, const char *name);
|
|
|
|
#endif
|