mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
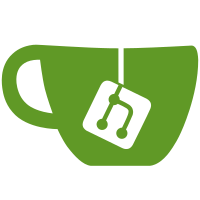
Since commit ee6d3dd4ed
("driver core: make kobj_type constant.") the
driver core allows the usage of const struct kobj_type.
Take advantage of this to constify the structure definitions to prevent
modification at runtime.
Link: https://lkml.kernel.org/r/20230207-kobj_type-damon-v1-1-9d4fea6a465b@weissschuh.net
Signed-off-by: Thomas Weißschuh <linux@weissschuh.net>
Reviewed-by: SeongJae Park <sj@kernel.org>
Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
107 lines
2.3 KiB
C
107 lines
2.3 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Common Primitives for DAMON Sysfs Interface
|
|
*
|
|
* Author: SeongJae Park <sj@kernel.org>
|
|
*/
|
|
|
|
#include <linux/slab.h>
|
|
|
|
#include "sysfs-common.h"
|
|
|
|
DEFINE_MUTEX(damon_sysfs_lock);
|
|
|
|
/*
|
|
* unsigned long range directory
|
|
*/
|
|
|
|
struct damon_sysfs_ul_range *damon_sysfs_ul_range_alloc(
|
|
unsigned long min,
|
|
unsigned long max)
|
|
{
|
|
struct damon_sysfs_ul_range *range = kmalloc(sizeof(*range),
|
|
GFP_KERNEL);
|
|
|
|
if (!range)
|
|
return NULL;
|
|
range->kobj = (struct kobject){};
|
|
range->min = min;
|
|
range->max = max;
|
|
|
|
return range;
|
|
}
|
|
|
|
static ssize_t min_show(struct kobject *kobj, struct kobj_attribute *attr,
|
|
char *buf)
|
|
{
|
|
struct damon_sysfs_ul_range *range = container_of(kobj,
|
|
struct damon_sysfs_ul_range, kobj);
|
|
|
|
return sysfs_emit(buf, "%lu\n", range->min);
|
|
}
|
|
|
|
static ssize_t min_store(struct kobject *kobj, struct kobj_attribute *attr,
|
|
const char *buf, size_t count)
|
|
{
|
|
struct damon_sysfs_ul_range *range = container_of(kobj,
|
|
struct damon_sysfs_ul_range, kobj);
|
|
unsigned long min;
|
|
int err;
|
|
|
|
err = kstrtoul(buf, 0, &min);
|
|
if (err)
|
|
return err;
|
|
|
|
range->min = min;
|
|
return count;
|
|
}
|
|
|
|
static ssize_t max_show(struct kobject *kobj, struct kobj_attribute *attr,
|
|
char *buf)
|
|
{
|
|
struct damon_sysfs_ul_range *range = container_of(kobj,
|
|
struct damon_sysfs_ul_range, kobj);
|
|
|
|
return sysfs_emit(buf, "%lu\n", range->max);
|
|
}
|
|
|
|
static ssize_t max_store(struct kobject *kobj, struct kobj_attribute *attr,
|
|
const char *buf, size_t count)
|
|
{
|
|
struct damon_sysfs_ul_range *range = container_of(kobj,
|
|
struct damon_sysfs_ul_range, kobj);
|
|
unsigned long max;
|
|
int err;
|
|
|
|
err = kstrtoul(buf, 0, &max);
|
|
if (err)
|
|
return err;
|
|
|
|
range->max = max;
|
|
return count;
|
|
}
|
|
|
|
void damon_sysfs_ul_range_release(struct kobject *kobj)
|
|
{
|
|
kfree(container_of(kobj, struct damon_sysfs_ul_range, kobj));
|
|
}
|
|
|
|
static struct kobj_attribute damon_sysfs_ul_range_min_attr =
|
|
__ATTR_RW_MODE(min, 0600);
|
|
|
|
static struct kobj_attribute damon_sysfs_ul_range_max_attr =
|
|
__ATTR_RW_MODE(max, 0600);
|
|
|
|
static struct attribute *damon_sysfs_ul_range_attrs[] = {
|
|
&damon_sysfs_ul_range_min_attr.attr,
|
|
&damon_sysfs_ul_range_max_attr.attr,
|
|
NULL,
|
|
};
|
|
ATTRIBUTE_GROUPS(damon_sysfs_ul_range);
|
|
|
|
const struct kobj_type damon_sysfs_ul_range_ktype = {
|
|
.release = damon_sysfs_ul_range_release,
|
|
.sysfs_ops = &kobj_sysfs_ops,
|
|
.default_groups = damon_sysfs_ul_range_groups,
|
|
};
|
|
|