mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
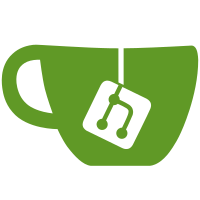
Both pgtable_cache_init() and pgd_cache_init() are used to initialize kmem cache for page table allocations on several architectures that do not use PAGE_SIZE tables for one or more levels of the page table hierarchy. Most architectures do not implement these functions and use __weak default NOP implementation of pgd_cache_init(). Since there is no such default for pgtable_cache_init(), its empty stub is duplicated among most architectures. Rename the definitions of pgd_cache_init() to pgtable_cache_init() and drop empty stubs of pgtable_cache_init(). Link: http://lkml.kernel.org/r/1566457046-22637-1-git-send-email-rppt@linux.ibm.com Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Acked-by: Will Deacon <will@kernel.org> [arm64] Acked-by: Thomas Gleixner <tglx@linutronix.de> [x86] Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Ingo Molnar <mingo@redhat.com> Cc: Borislav Petkov <bp@alien8.de> Cc: Matthew Wilcox <willy@infradead.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
56 lines
1.1 KiB
C
56 lines
1.1 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* PGD allocation/freeing
|
|
*
|
|
* Copyright (C) 2012 ARM Ltd.
|
|
* Author: Catalin Marinas <catalin.marinas@arm.com>
|
|
*/
|
|
|
|
#include <linux/mm.h>
|
|
#include <linux/gfp.h>
|
|
#include <linux/highmem.h>
|
|
#include <linux/slab.h>
|
|
|
|
#include <asm/pgalloc.h>
|
|
#include <asm/page.h>
|
|
#include <asm/tlbflush.h>
|
|
|
|
static struct kmem_cache *pgd_cache __ro_after_init;
|
|
|
|
pgd_t *pgd_alloc(struct mm_struct *mm)
|
|
{
|
|
gfp_t gfp = GFP_PGTABLE_USER;
|
|
|
|
if (PGD_SIZE == PAGE_SIZE)
|
|
return (pgd_t *)__get_free_page(gfp);
|
|
else
|
|
return kmem_cache_alloc(pgd_cache, gfp);
|
|
}
|
|
|
|
void pgd_free(struct mm_struct *mm, pgd_t *pgd)
|
|
{
|
|
if (PGD_SIZE == PAGE_SIZE)
|
|
free_page((unsigned long)pgd);
|
|
else
|
|
kmem_cache_free(pgd_cache, pgd);
|
|
}
|
|
|
|
void __init pgtable_cache_init(void)
|
|
{
|
|
if (PGD_SIZE == PAGE_SIZE)
|
|
return;
|
|
|
|
#ifdef CONFIG_ARM64_PA_BITS_52
|
|
/*
|
|
* With 52-bit physical addresses, the architecture requires the
|
|
* top-level table to be aligned to at least 64 bytes.
|
|
*/
|
|
BUILD_BUG_ON(PGD_SIZE < 64);
|
|
#endif
|
|
|
|
/*
|
|
* Naturally aligned pgds required by the architecture.
|
|
*/
|
|
pgd_cache = kmem_cache_create("pgd_cache", PGD_SIZE, PGD_SIZE,
|
|
SLAB_PANIC, NULL);
|
|
}
|