mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-30 22:26:55 +00:00
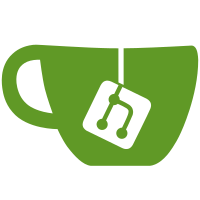
We'd like all architectures to convert to ARCH_ATOMIC, as once all architectures are converted it will be possible to make significant cleanups to the atomics headers, and this will make it much easier to generically enable atomic functionality (e.g. debug logic in the instrumented wrappers). As a step towards that, this patch migrates sh to ARCH_ATOMIC. The arch code provides arch_{atomic,atomic64,xchg,cmpxchg}*(), and common code wraps these with optional instrumentation to provide the regular functions. Signed-off-by: Mark Rutland <mark.rutland@arm.com> Cc: Boqun Feng <boqun.feng@gmail.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Rich Felker <dalias@libc.org> Cc: Will Deacon <will@kernel.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Signed-off-by: Peter Zijlstra (Intel) <peterz@infradead.org> Link: https://lore.kernel.org/r/20210525140232.53872-30-mark.rutland@arm.com
88 lines
2.1 KiB
C
88 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __ASM_SH_ATOMIC_LLSC_H
|
|
#define __ASM_SH_ATOMIC_LLSC_H
|
|
|
|
/*
|
|
* SH-4A note:
|
|
*
|
|
* We basically get atomic_xxx_return() for free compared with
|
|
* atomic_xxx(). movli.l/movco.l require r0 due to the instruction
|
|
* encoding, so the retval is automatically set without having to
|
|
* do any special work.
|
|
*/
|
|
/*
|
|
* To get proper branch prediction for the main line, we must branch
|
|
* forward to code at the end of this object's .text section, then
|
|
* branch back to restart the operation.
|
|
*/
|
|
|
|
#define ATOMIC_OP(op) \
|
|
static inline void arch_atomic_##op(int i, atomic_t *v) \
|
|
{ \
|
|
unsigned long tmp; \
|
|
\
|
|
__asm__ __volatile__ ( \
|
|
"1: movli.l @%2, %0 ! atomic_" #op "\n" \
|
|
" " #op " %1, %0 \n" \
|
|
" movco.l %0, @%2 \n" \
|
|
" bf 1b \n" \
|
|
: "=&z" (tmp) \
|
|
: "r" (i), "r" (&v->counter) \
|
|
: "t"); \
|
|
}
|
|
|
|
#define ATOMIC_OP_RETURN(op) \
|
|
static inline int arch_atomic_##op##_return(int i, atomic_t *v) \
|
|
{ \
|
|
unsigned long temp; \
|
|
\
|
|
__asm__ __volatile__ ( \
|
|
"1: movli.l @%2, %0 ! atomic_" #op "_return \n" \
|
|
" " #op " %1, %0 \n" \
|
|
" movco.l %0, @%2 \n" \
|
|
" bf 1b \n" \
|
|
" synco \n" \
|
|
: "=&z" (temp) \
|
|
: "r" (i), "r" (&v->counter) \
|
|
: "t"); \
|
|
\
|
|
return temp; \
|
|
}
|
|
|
|
#define ATOMIC_FETCH_OP(op) \
|
|
static inline int arch_atomic_fetch_##op(int i, atomic_t *v) \
|
|
{ \
|
|
unsigned long res, temp; \
|
|
\
|
|
__asm__ __volatile__ ( \
|
|
"1: movli.l @%3, %0 ! atomic_fetch_" #op " \n" \
|
|
" mov %0, %1 \n" \
|
|
" " #op " %2, %0 \n" \
|
|
" movco.l %0, @%3 \n" \
|
|
" bf 1b \n" \
|
|
" synco \n" \
|
|
: "=&z" (temp), "=&r" (res) \
|
|
: "r" (i), "r" (&v->counter) \
|
|
: "t"); \
|
|
\
|
|
return res; \
|
|
}
|
|
|
|
#define ATOMIC_OPS(op) ATOMIC_OP(op) ATOMIC_OP_RETURN(op) ATOMIC_FETCH_OP(op)
|
|
|
|
ATOMIC_OPS(add)
|
|
ATOMIC_OPS(sub)
|
|
|
|
#undef ATOMIC_OPS
|
|
#define ATOMIC_OPS(op) ATOMIC_OP(op) ATOMIC_FETCH_OP(op)
|
|
|
|
ATOMIC_OPS(and)
|
|
ATOMIC_OPS(or)
|
|
ATOMIC_OPS(xor)
|
|
|
|
#undef ATOMIC_OPS
|
|
#undef ATOMIC_FETCH_OP
|
|
#undef ATOMIC_OP_RETURN
|
|
#undef ATOMIC_OP
|
|
|
|
#endif /* __ASM_SH_ATOMIC_LLSC_H */
|