mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-04 16:15:11 +00:00
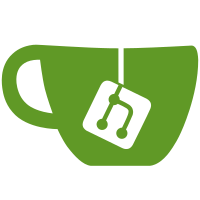
[ Upstream commite33cb5702a
] Benchmark command is used in multiple tests so it should not be mutated by the tests but CMT test alters span argument. Due to the order of tests (CMT test runs last), mutating the span argument in CMT test does not trigger any real problems currently. Mark benchmark_cmd strings as const and setup the benchmark command using pointers. Because the benchmark command becomes const, the input arguments can be used directly. Besides being simpler, using the input arguments directly also removes the internal size restriction. CMT test has to create a copy of the benchmark command before altering the benchmark command. Signed-off-by: Ilpo Järvinen <ilpo.jarvinen@linux.intel.com> Tested-by: Shaopeng Tan <tan.shaopeng@jp.fujitsu.com> Reviewed-by: Shaopeng Tan <tan.shaopeng@jp.fujitsu.com> Reviewed-by: Reinette Chatre <reinette.chatre@intel.com> Reviewed-by: "Wieczor-Retman, Maciej" <maciej.wieczor-retman@intel.com> Signed-off-by: Shuah Khan <skhan@linuxfoundation.org> Stable-dep-of:3aff514644
("selftests/resctrl: Extend signal handler coverage to unmount on receiving signal") Signed-off-by: Sasha Levin <sashal@kernel.org>
169 lines
3.9 KiB
C
169 lines
3.9 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Memory Bandwidth Allocation (MBA) test
|
|
*
|
|
* Copyright (C) 2018 Intel Corporation
|
|
*
|
|
* Authors:
|
|
* Sai Praneeth Prakhya <sai.praneeth.prakhya@intel.com>,
|
|
* Fenghua Yu <fenghua.yu@intel.com>
|
|
*/
|
|
#include "resctrl.h"
|
|
|
|
#define RESULT_FILE_NAME "result_mba"
|
|
#define NUM_OF_RUNS 5
|
|
#define MAX_DIFF_PERCENT 8
|
|
#define ALLOCATION_MAX 100
|
|
#define ALLOCATION_MIN 10
|
|
#define ALLOCATION_STEP 10
|
|
|
|
/*
|
|
* Change schemata percentage from 100 to 10%. Write schemata to specified
|
|
* con_mon grp, mon_grp in resctrl FS.
|
|
* For each allocation, run 5 times in order to get average values.
|
|
*/
|
|
static int mba_setup(struct resctrl_val_param *p)
|
|
{
|
|
static int runs_per_allocation, allocation = 100;
|
|
char allocation_str[64];
|
|
int ret;
|
|
|
|
if (runs_per_allocation >= NUM_OF_RUNS)
|
|
runs_per_allocation = 0;
|
|
|
|
/* Only set up schemata once every NUM_OF_RUNS of allocations */
|
|
if (runs_per_allocation++ != 0)
|
|
return 0;
|
|
|
|
if (allocation < ALLOCATION_MIN || allocation > ALLOCATION_MAX)
|
|
return END_OF_TESTS;
|
|
|
|
sprintf(allocation_str, "%d", allocation);
|
|
|
|
ret = write_schemata(p->ctrlgrp, allocation_str, p->cpu_no,
|
|
p->resctrl_val);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
allocation -= ALLOCATION_STEP;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static bool show_mba_info(unsigned long *bw_imc, unsigned long *bw_resc)
|
|
{
|
|
int allocation, runs;
|
|
bool ret = false;
|
|
|
|
ksft_print_msg("Results are displayed in (MB)\n");
|
|
/* Memory bandwidth from 100% down to 10% */
|
|
for (allocation = 0; allocation < ALLOCATION_MAX / ALLOCATION_STEP;
|
|
allocation++) {
|
|
unsigned long avg_bw_imc, avg_bw_resc;
|
|
unsigned long sum_bw_imc = 0, sum_bw_resc = 0;
|
|
int avg_diff_per;
|
|
float avg_diff;
|
|
|
|
/*
|
|
* The first run is discarded due to inaccurate value from
|
|
* phase transition.
|
|
*/
|
|
for (runs = NUM_OF_RUNS * allocation + 1;
|
|
runs < NUM_OF_RUNS * allocation + NUM_OF_RUNS ; runs++) {
|
|
sum_bw_imc += bw_imc[runs];
|
|
sum_bw_resc += bw_resc[runs];
|
|
}
|
|
|
|
avg_bw_imc = sum_bw_imc / (NUM_OF_RUNS - 1);
|
|
avg_bw_resc = sum_bw_resc / (NUM_OF_RUNS - 1);
|
|
avg_diff = (float)labs(avg_bw_resc - avg_bw_imc) / avg_bw_imc;
|
|
avg_diff_per = (int)(avg_diff * 100);
|
|
|
|
ksft_print_msg("%s Check MBA diff within %d%% for schemata %u\n",
|
|
avg_diff_per > MAX_DIFF_PERCENT ?
|
|
"Fail:" : "Pass:",
|
|
MAX_DIFF_PERCENT,
|
|
ALLOCATION_MAX - ALLOCATION_STEP * allocation);
|
|
|
|
ksft_print_msg("avg_diff_per: %d%%\n", avg_diff_per);
|
|
ksft_print_msg("avg_bw_imc: %lu\n", avg_bw_imc);
|
|
ksft_print_msg("avg_bw_resc: %lu\n", avg_bw_resc);
|
|
if (avg_diff_per > MAX_DIFF_PERCENT)
|
|
ret = true;
|
|
}
|
|
|
|
ksft_print_msg("%s Check schemata change using MBA\n",
|
|
ret ? "Fail:" : "Pass:");
|
|
if (ret)
|
|
ksft_print_msg("At least one test failed\n");
|
|
|
|
return ret;
|
|
}
|
|
|
|
static int check_results(void)
|
|
{
|
|
char *token_array[8], output[] = RESULT_FILE_NAME, temp[512];
|
|
unsigned long bw_imc[1024], bw_resc[1024];
|
|
int runs;
|
|
FILE *fp;
|
|
|
|
fp = fopen(output, "r");
|
|
if (!fp) {
|
|
perror(output);
|
|
|
|
return errno;
|
|
}
|
|
|
|
runs = 0;
|
|
while (fgets(temp, sizeof(temp), fp)) {
|
|
char *token = strtok(temp, ":\t");
|
|
int fields = 0;
|
|
|
|
while (token) {
|
|
token_array[fields++] = token;
|
|
token = strtok(NULL, ":\t");
|
|
}
|
|
|
|
/* Field 3 is perf imc value */
|
|
bw_imc[runs] = strtoul(token_array[3], NULL, 0);
|
|
/* Field 5 is resctrl value */
|
|
bw_resc[runs] = strtoul(token_array[5], NULL, 0);
|
|
runs++;
|
|
}
|
|
|
|
fclose(fp);
|
|
|
|
return show_mba_info(bw_imc, bw_resc);
|
|
}
|
|
|
|
void mba_test_cleanup(void)
|
|
{
|
|
remove(RESULT_FILE_NAME);
|
|
}
|
|
|
|
int mba_schemata_change(int cpu_no, const char * const *benchmark_cmd)
|
|
{
|
|
struct resctrl_val_param param = {
|
|
.resctrl_val = MBA_STR,
|
|
.ctrlgrp = "c1",
|
|
.mongrp = "m1",
|
|
.cpu_no = cpu_no,
|
|
.filename = RESULT_FILE_NAME,
|
|
.bw_report = "reads",
|
|
.setup = mba_setup
|
|
};
|
|
int ret;
|
|
|
|
remove(RESULT_FILE_NAME);
|
|
|
|
ret = resctrl_val(benchmark_cmd, ¶m);
|
|
if (ret)
|
|
goto out;
|
|
|
|
ret = check_results();
|
|
|
|
out:
|
|
mba_test_cleanup();
|
|
|
|
return ret;
|
|
}
|