mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 17:59:32 +00:00
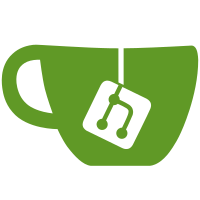
The soft-dirty is a bit on a PTE which helps to track which pages a task writes to. In order to do this tracking one should 1. Clear soft-dirty bits from PTEs ("echo 4 > /proc/PID/clear_refs) 2. Wait some time. 3. Read soft-dirty bits (55'th in /proc/PID/pagemap2 entries) To do this tracking, the writable bit is cleared from PTEs when the soft-dirty bit is. Thus, after this, when the task tries to modify a page at some virtual address the #PF occurs and the kernel sets the soft-dirty bit on the respective PTE. Note, that although all the task's address space is marked as r/o after the soft-dirty bits clear, the #PF-s that occur after that are processed fast. This is so, since the pages are still mapped to physical memory, and thus all the kernel does is finds this fact out and puts back writable, dirty and soft-dirty bits on the PTE. Another thing to note, is that when mremap moves PTEs they are marked with soft-dirty as well, since from the user perspective mremap modifies the virtual memory at mremap's new address. Signed-off-by: Pavel Emelyanov <xemul@parallels.com> Cc: Matt Mackall <mpm@selenic.com> Cc: Xiao Guangrong <xiaoguangrong@linux.vnet.ibm.com> Cc: Glauber Costa <glommer@parallels.com> Cc: Marcelo Tosatti <mtosatti@redhat.com> Cc: KOSAKI Motohiro <kosaki.motohiro@gmail.com> Cc: Stephen Rothwell <sfr@canb.auug.org.au> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
370 lines
12 KiB
C
370 lines
12 KiB
C
#ifndef _ASM_X86_PGTABLE_DEFS_H
|
|
#define _ASM_X86_PGTABLE_DEFS_H
|
|
|
|
#include <linux/const.h>
|
|
#include <asm/page_types.h>
|
|
|
|
#define FIRST_USER_ADDRESS 0
|
|
|
|
#define _PAGE_BIT_PRESENT 0 /* is present */
|
|
#define _PAGE_BIT_RW 1 /* writeable */
|
|
#define _PAGE_BIT_USER 2 /* userspace addressable */
|
|
#define _PAGE_BIT_PWT 3 /* page write through */
|
|
#define _PAGE_BIT_PCD 4 /* page cache disabled */
|
|
#define _PAGE_BIT_ACCESSED 5 /* was accessed (raised by CPU) */
|
|
#define _PAGE_BIT_DIRTY 6 /* was written to (raised by CPU) */
|
|
#define _PAGE_BIT_PSE 7 /* 4 MB (or 2MB) page */
|
|
#define _PAGE_BIT_PAT 7 /* on 4KB pages */
|
|
#define _PAGE_BIT_GLOBAL 8 /* Global TLB entry PPro+ */
|
|
#define _PAGE_BIT_UNUSED1 9 /* available for programmer */
|
|
#define _PAGE_BIT_IOMAP 10 /* flag used to indicate IO mapping */
|
|
#define _PAGE_BIT_HIDDEN 11 /* hidden by kmemcheck */
|
|
#define _PAGE_BIT_PAT_LARGE 12 /* On 2MB or 1GB pages */
|
|
#define _PAGE_BIT_SPECIAL _PAGE_BIT_UNUSED1
|
|
#define _PAGE_BIT_CPA_TEST _PAGE_BIT_UNUSED1
|
|
#define _PAGE_BIT_SPLITTING _PAGE_BIT_UNUSED1 /* only valid on a PSE pmd */
|
|
#define _PAGE_BIT_NX 63 /* No execute: only valid after cpuid check */
|
|
|
|
/* If _PAGE_BIT_PRESENT is clear, we use these: */
|
|
/* - if the user mapped it with PROT_NONE; pte_present gives true */
|
|
#define _PAGE_BIT_PROTNONE _PAGE_BIT_GLOBAL
|
|
/* - set: nonlinear file mapping, saved PTE; unset:swap */
|
|
#define _PAGE_BIT_FILE _PAGE_BIT_DIRTY
|
|
|
|
#define _PAGE_PRESENT (_AT(pteval_t, 1) << _PAGE_BIT_PRESENT)
|
|
#define _PAGE_RW (_AT(pteval_t, 1) << _PAGE_BIT_RW)
|
|
#define _PAGE_USER (_AT(pteval_t, 1) << _PAGE_BIT_USER)
|
|
#define _PAGE_PWT (_AT(pteval_t, 1) << _PAGE_BIT_PWT)
|
|
#define _PAGE_PCD (_AT(pteval_t, 1) << _PAGE_BIT_PCD)
|
|
#define _PAGE_ACCESSED (_AT(pteval_t, 1) << _PAGE_BIT_ACCESSED)
|
|
#define _PAGE_DIRTY (_AT(pteval_t, 1) << _PAGE_BIT_DIRTY)
|
|
#define _PAGE_PSE (_AT(pteval_t, 1) << _PAGE_BIT_PSE)
|
|
#define _PAGE_GLOBAL (_AT(pteval_t, 1) << _PAGE_BIT_GLOBAL)
|
|
#define _PAGE_UNUSED1 (_AT(pteval_t, 1) << _PAGE_BIT_UNUSED1)
|
|
#define _PAGE_IOMAP (_AT(pteval_t, 1) << _PAGE_BIT_IOMAP)
|
|
#define _PAGE_PAT (_AT(pteval_t, 1) << _PAGE_BIT_PAT)
|
|
#define _PAGE_PAT_LARGE (_AT(pteval_t, 1) << _PAGE_BIT_PAT_LARGE)
|
|
#define _PAGE_SPECIAL (_AT(pteval_t, 1) << _PAGE_BIT_SPECIAL)
|
|
#define _PAGE_CPA_TEST (_AT(pteval_t, 1) << _PAGE_BIT_CPA_TEST)
|
|
#define _PAGE_SPLITTING (_AT(pteval_t, 1) << _PAGE_BIT_SPLITTING)
|
|
#define __HAVE_ARCH_PTE_SPECIAL
|
|
|
|
#ifdef CONFIG_KMEMCHECK
|
|
#define _PAGE_HIDDEN (_AT(pteval_t, 1) << _PAGE_BIT_HIDDEN)
|
|
#else
|
|
#define _PAGE_HIDDEN (_AT(pteval_t, 0))
|
|
#endif
|
|
|
|
/*
|
|
* The same hidden bit is used by kmemcheck, but since kmemcheck
|
|
* works on kernel pages while soft-dirty engine on user space,
|
|
* they do not conflict with each other.
|
|
*/
|
|
|
|
#ifdef CONFIG_MEM_SOFT_DIRTY
|
|
#define _PAGE_SOFT_DIRTY (_AT(pteval_t, 1) << _PAGE_BIT_HIDDEN)
|
|
#else
|
|
#define _PAGE_SOFT_DIRTY (_AT(pteval_t, 0))
|
|
#endif
|
|
|
|
#if defined(CONFIG_X86_64) || defined(CONFIG_X86_PAE)
|
|
#define _PAGE_NX (_AT(pteval_t, 1) << _PAGE_BIT_NX)
|
|
#else
|
|
#define _PAGE_NX (_AT(pteval_t, 0))
|
|
#endif
|
|
|
|
#define _PAGE_FILE (_AT(pteval_t, 1) << _PAGE_BIT_FILE)
|
|
#define _PAGE_PROTNONE (_AT(pteval_t, 1) << _PAGE_BIT_PROTNONE)
|
|
|
|
/*
|
|
* _PAGE_NUMA indicates that this page will trigger a numa hinting
|
|
* minor page fault to gather numa placement statistics (see
|
|
* pte_numa()). The bit picked (8) is within the range between
|
|
* _PAGE_FILE (6) and _PAGE_PROTNONE (8) bits. Therefore, it doesn't
|
|
* require changes to the swp entry format because that bit is always
|
|
* zero when the pte is not present.
|
|
*
|
|
* The bit picked must be always zero when the pmd is present and not
|
|
* present, so that we don't lose information when we set it while
|
|
* atomically clearing the present bit.
|
|
*
|
|
* Because we shared the same bit (8) with _PAGE_PROTNONE this can be
|
|
* interpreted as _PAGE_NUMA only in places that _PAGE_PROTNONE
|
|
* couldn't reach, like handle_mm_fault() (see access_error in
|
|
* arch/x86/mm/fault.c, the vma protection must not be PROT_NONE for
|
|
* handle_mm_fault() to be invoked).
|
|
*/
|
|
#define _PAGE_NUMA _PAGE_PROTNONE
|
|
|
|
#define _PAGE_TABLE (_PAGE_PRESENT | _PAGE_RW | _PAGE_USER | \
|
|
_PAGE_ACCESSED | _PAGE_DIRTY)
|
|
#define _KERNPG_TABLE (_PAGE_PRESENT | _PAGE_RW | _PAGE_ACCESSED | \
|
|
_PAGE_DIRTY)
|
|
|
|
/* Set of bits not changed in pte_modify */
|
|
#define _PAGE_CHG_MASK (PTE_PFN_MASK | _PAGE_PCD | _PAGE_PWT | \
|
|
_PAGE_SPECIAL | _PAGE_ACCESSED | _PAGE_DIRTY)
|
|
#define _HPAGE_CHG_MASK (_PAGE_CHG_MASK | _PAGE_PSE)
|
|
|
|
#define _PAGE_CACHE_MASK (_PAGE_PCD | _PAGE_PWT)
|
|
#define _PAGE_CACHE_WB (0)
|
|
#define _PAGE_CACHE_WC (_PAGE_PWT)
|
|
#define _PAGE_CACHE_UC_MINUS (_PAGE_PCD)
|
|
#define _PAGE_CACHE_UC (_PAGE_PCD | _PAGE_PWT)
|
|
|
|
#define PAGE_NONE __pgprot(_PAGE_PROTNONE | _PAGE_ACCESSED)
|
|
#define PAGE_SHARED __pgprot(_PAGE_PRESENT | _PAGE_RW | _PAGE_USER | \
|
|
_PAGE_ACCESSED | _PAGE_NX)
|
|
|
|
#define PAGE_SHARED_EXEC __pgprot(_PAGE_PRESENT | _PAGE_RW | \
|
|
_PAGE_USER | _PAGE_ACCESSED)
|
|
#define PAGE_COPY_NOEXEC __pgprot(_PAGE_PRESENT | _PAGE_USER | \
|
|
_PAGE_ACCESSED | _PAGE_NX)
|
|
#define PAGE_COPY_EXEC __pgprot(_PAGE_PRESENT | _PAGE_USER | \
|
|
_PAGE_ACCESSED)
|
|
#define PAGE_COPY PAGE_COPY_NOEXEC
|
|
#define PAGE_READONLY __pgprot(_PAGE_PRESENT | _PAGE_USER | \
|
|
_PAGE_ACCESSED | _PAGE_NX)
|
|
#define PAGE_READONLY_EXEC __pgprot(_PAGE_PRESENT | _PAGE_USER | \
|
|
_PAGE_ACCESSED)
|
|
|
|
#define __PAGE_KERNEL_EXEC \
|
|
(_PAGE_PRESENT | _PAGE_RW | _PAGE_DIRTY | _PAGE_ACCESSED | _PAGE_GLOBAL)
|
|
#define __PAGE_KERNEL (__PAGE_KERNEL_EXEC | _PAGE_NX)
|
|
|
|
#define __PAGE_KERNEL_RO (__PAGE_KERNEL & ~_PAGE_RW)
|
|
#define __PAGE_KERNEL_RX (__PAGE_KERNEL_EXEC & ~_PAGE_RW)
|
|
#define __PAGE_KERNEL_EXEC_NOCACHE (__PAGE_KERNEL_EXEC | _PAGE_PCD | _PAGE_PWT)
|
|
#define __PAGE_KERNEL_WC (__PAGE_KERNEL | _PAGE_CACHE_WC)
|
|
#define __PAGE_KERNEL_NOCACHE (__PAGE_KERNEL | _PAGE_PCD | _PAGE_PWT)
|
|
#define __PAGE_KERNEL_UC_MINUS (__PAGE_KERNEL | _PAGE_PCD)
|
|
#define __PAGE_KERNEL_VSYSCALL (__PAGE_KERNEL_RX | _PAGE_USER)
|
|
#define __PAGE_KERNEL_VVAR (__PAGE_KERNEL_RO | _PAGE_USER)
|
|
#define __PAGE_KERNEL_VVAR_NOCACHE (__PAGE_KERNEL_VVAR | _PAGE_PCD | _PAGE_PWT)
|
|
#define __PAGE_KERNEL_LARGE (__PAGE_KERNEL | _PAGE_PSE)
|
|
#define __PAGE_KERNEL_LARGE_NOCACHE (__PAGE_KERNEL | _PAGE_CACHE_UC | _PAGE_PSE)
|
|
#define __PAGE_KERNEL_LARGE_EXEC (__PAGE_KERNEL_EXEC | _PAGE_PSE)
|
|
|
|
#define __PAGE_KERNEL_IO (__PAGE_KERNEL | _PAGE_IOMAP)
|
|
#define __PAGE_KERNEL_IO_NOCACHE (__PAGE_KERNEL_NOCACHE | _PAGE_IOMAP)
|
|
#define __PAGE_KERNEL_IO_UC_MINUS (__PAGE_KERNEL_UC_MINUS | _PAGE_IOMAP)
|
|
#define __PAGE_KERNEL_IO_WC (__PAGE_KERNEL_WC | _PAGE_IOMAP)
|
|
|
|
#define PAGE_KERNEL __pgprot(__PAGE_KERNEL)
|
|
#define PAGE_KERNEL_RO __pgprot(__PAGE_KERNEL_RO)
|
|
#define PAGE_KERNEL_EXEC __pgprot(__PAGE_KERNEL_EXEC)
|
|
#define PAGE_KERNEL_RX __pgprot(__PAGE_KERNEL_RX)
|
|
#define PAGE_KERNEL_WC __pgprot(__PAGE_KERNEL_WC)
|
|
#define PAGE_KERNEL_NOCACHE __pgprot(__PAGE_KERNEL_NOCACHE)
|
|
#define PAGE_KERNEL_UC_MINUS __pgprot(__PAGE_KERNEL_UC_MINUS)
|
|
#define PAGE_KERNEL_EXEC_NOCACHE __pgprot(__PAGE_KERNEL_EXEC_NOCACHE)
|
|
#define PAGE_KERNEL_LARGE __pgprot(__PAGE_KERNEL_LARGE)
|
|
#define PAGE_KERNEL_LARGE_NOCACHE __pgprot(__PAGE_KERNEL_LARGE_NOCACHE)
|
|
#define PAGE_KERNEL_LARGE_EXEC __pgprot(__PAGE_KERNEL_LARGE_EXEC)
|
|
#define PAGE_KERNEL_VSYSCALL __pgprot(__PAGE_KERNEL_VSYSCALL)
|
|
#define PAGE_KERNEL_VVAR __pgprot(__PAGE_KERNEL_VVAR)
|
|
#define PAGE_KERNEL_VVAR_NOCACHE __pgprot(__PAGE_KERNEL_VVAR_NOCACHE)
|
|
|
|
#define PAGE_KERNEL_IO __pgprot(__PAGE_KERNEL_IO)
|
|
#define PAGE_KERNEL_IO_NOCACHE __pgprot(__PAGE_KERNEL_IO_NOCACHE)
|
|
#define PAGE_KERNEL_IO_UC_MINUS __pgprot(__PAGE_KERNEL_IO_UC_MINUS)
|
|
#define PAGE_KERNEL_IO_WC __pgprot(__PAGE_KERNEL_IO_WC)
|
|
|
|
/* xwr */
|
|
#define __P000 PAGE_NONE
|
|
#define __P001 PAGE_READONLY
|
|
#define __P010 PAGE_COPY
|
|
#define __P011 PAGE_COPY
|
|
#define __P100 PAGE_READONLY_EXEC
|
|
#define __P101 PAGE_READONLY_EXEC
|
|
#define __P110 PAGE_COPY_EXEC
|
|
#define __P111 PAGE_COPY_EXEC
|
|
|
|
#define __S000 PAGE_NONE
|
|
#define __S001 PAGE_READONLY
|
|
#define __S010 PAGE_SHARED
|
|
#define __S011 PAGE_SHARED
|
|
#define __S100 PAGE_READONLY_EXEC
|
|
#define __S101 PAGE_READONLY_EXEC
|
|
#define __S110 PAGE_SHARED_EXEC
|
|
#define __S111 PAGE_SHARED_EXEC
|
|
|
|
/*
|
|
* early identity mapping pte attrib macros.
|
|
*/
|
|
#ifdef CONFIG_X86_64
|
|
#define __PAGE_KERNEL_IDENT_LARGE_EXEC __PAGE_KERNEL_LARGE_EXEC
|
|
#else
|
|
/*
|
|
* For PDE_IDENT_ATTR include USER bit. As the PDE and PTE protection
|
|
* bits are combined, this will alow user to access the high address mapped
|
|
* VDSO in the presence of CONFIG_COMPAT_VDSO
|
|
*/
|
|
#define PTE_IDENT_ATTR 0x003 /* PRESENT+RW */
|
|
#define PDE_IDENT_ATTR 0x067 /* PRESENT+RW+USER+DIRTY+ACCESSED */
|
|
#define PGD_IDENT_ATTR 0x001 /* PRESENT (no other attributes) */
|
|
#endif
|
|
|
|
#ifdef CONFIG_X86_32
|
|
# include <asm/pgtable_32_types.h>
|
|
#else
|
|
# include <asm/pgtable_64_types.h>
|
|
#endif
|
|
|
|
#ifndef __ASSEMBLY__
|
|
|
|
#include <linux/types.h>
|
|
|
|
/* PTE_PFN_MASK extracts the PFN from a (pte|pmd|pud|pgd)val_t */
|
|
#define PTE_PFN_MASK ((pteval_t)PHYSICAL_PAGE_MASK)
|
|
|
|
/* PTE_FLAGS_MASK extracts the flags from a (pte|pmd|pud|pgd)val_t */
|
|
#define PTE_FLAGS_MASK (~PTE_PFN_MASK)
|
|
|
|
typedef struct pgprot { pgprotval_t pgprot; } pgprot_t;
|
|
|
|
typedef struct { pgdval_t pgd; } pgd_t;
|
|
|
|
static inline pgd_t native_make_pgd(pgdval_t val)
|
|
{
|
|
return (pgd_t) { val };
|
|
}
|
|
|
|
static inline pgdval_t native_pgd_val(pgd_t pgd)
|
|
{
|
|
return pgd.pgd;
|
|
}
|
|
|
|
static inline pgdval_t pgd_flags(pgd_t pgd)
|
|
{
|
|
return native_pgd_val(pgd) & PTE_FLAGS_MASK;
|
|
}
|
|
|
|
#if PAGETABLE_LEVELS > 3
|
|
typedef struct { pudval_t pud; } pud_t;
|
|
|
|
static inline pud_t native_make_pud(pmdval_t val)
|
|
{
|
|
return (pud_t) { val };
|
|
}
|
|
|
|
static inline pudval_t native_pud_val(pud_t pud)
|
|
{
|
|
return pud.pud;
|
|
}
|
|
#else
|
|
#include <asm-generic/pgtable-nopud.h>
|
|
|
|
static inline pudval_t native_pud_val(pud_t pud)
|
|
{
|
|
return native_pgd_val(pud.pgd);
|
|
}
|
|
#endif
|
|
|
|
#if PAGETABLE_LEVELS > 2
|
|
typedef struct { pmdval_t pmd; } pmd_t;
|
|
|
|
static inline pmd_t native_make_pmd(pmdval_t val)
|
|
{
|
|
return (pmd_t) { val };
|
|
}
|
|
|
|
static inline pmdval_t native_pmd_val(pmd_t pmd)
|
|
{
|
|
return pmd.pmd;
|
|
}
|
|
#else
|
|
#include <asm-generic/pgtable-nopmd.h>
|
|
|
|
static inline pmdval_t native_pmd_val(pmd_t pmd)
|
|
{
|
|
return native_pgd_val(pmd.pud.pgd);
|
|
}
|
|
#endif
|
|
|
|
static inline pudval_t pud_flags(pud_t pud)
|
|
{
|
|
return native_pud_val(pud) & PTE_FLAGS_MASK;
|
|
}
|
|
|
|
static inline pmdval_t pmd_flags(pmd_t pmd)
|
|
{
|
|
return native_pmd_val(pmd) & PTE_FLAGS_MASK;
|
|
}
|
|
|
|
static inline pte_t native_make_pte(pteval_t val)
|
|
{
|
|
return (pte_t) { .pte = val };
|
|
}
|
|
|
|
static inline pteval_t native_pte_val(pte_t pte)
|
|
{
|
|
return pte.pte;
|
|
}
|
|
|
|
static inline pteval_t pte_flags(pte_t pte)
|
|
{
|
|
return native_pte_val(pte) & PTE_FLAGS_MASK;
|
|
}
|
|
|
|
#define pgprot_val(x) ((x).pgprot)
|
|
#define __pgprot(x) ((pgprot_t) { (x) } )
|
|
|
|
|
|
typedef struct page *pgtable_t;
|
|
|
|
extern pteval_t __supported_pte_mask;
|
|
extern void set_nx(void);
|
|
extern int nx_enabled;
|
|
|
|
#define pgprot_writecombine pgprot_writecombine
|
|
extern pgprot_t pgprot_writecombine(pgprot_t prot);
|
|
|
|
/* Indicate that x86 has its own track and untrack pfn vma functions */
|
|
#define __HAVE_PFNMAP_TRACKING
|
|
|
|
#define __HAVE_PHYS_MEM_ACCESS_PROT
|
|
struct file;
|
|
pgprot_t phys_mem_access_prot(struct file *file, unsigned long pfn,
|
|
unsigned long size, pgprot_t vma_prot);
|
|
int phys_mem_access_prot_allowed(struct file *file, unsigned long pfn,
|
|
unsigned long size, pgprot_t *vma_prot);
|
|
|
|
/* Install a pte for a particular vaddr in kernel space. */
|
|
void set_pte_vaddr(unsigned long vaddr, pte_t pte);
|
|
|
|
#ifdef CONFIG_X86_32
|
|
extern void native_pagetable_init(void);
|
|
#else
|
|
#define native_pagetable_init paging_init
|
|
#endif
|
|
|
|
struct seq_file;
|
|
extern void arch_report_meminfo(struct seq_file *m);
|
|
|
|
enum pg_level {
|
|
PG_LEVEL_NONE,
|
|
PG_LEVEL_4K,
|
|
PG_LEVEL_2M,
|
|
PG_LEVEL_1G,
|
|
PG_LEVEL_NUM
|
|
};
|
|
|
|
#ifdef CONFIG_PROC_FS
|
|
extern void update_page_count(int level, unsigned long pages);
|
|
#else
|
|
static inline void update_page_count(int level, unsigned long pages) { }
|
|
#endif
|
|
|
|
/*
|
|
* Helper function that returns the kernel pagetable entry controlling
|
|
* the virtual address 'address'. NULL means no pagetable entry present.
|
|
* NOTE: the return type is pte_t but if the pmd is PSE then we return it
|
|
* as a pte too.
|
|
*/
|
|
extern pte_t *lookup_address(unsigned long address, unsigned int *level);
|
|
extern phys_addr_t slow_virt_to_phys(void *__address);
|
|
|
|
#endif /* !__ASSEMBLY__ */
|
|
|
|
#endif /* _ASM_X86_PGTABLE_DEFS_H */
|