mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 07:04:44 +00:00
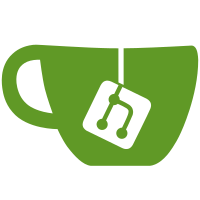
Add fprobe events for tracing function entry and exit instead of kprobe events. With this change, we can continue to trace function entry/exit even if the CONFIG_KPROBES_ON_FTRACE is not available. Since CONFIG_KPROBES_ON_FTRACE requires the CONFIG_DYNAMIC_FTRACE_WITH_REGS, it is not available if the architecture only supports CONFIG_DYNAMIC_FTRACE_WITH_ARGS. And that means kprobe events can not probe function entry/exit effectively on such architecture. But this can be solved if the dynamic events supports fprobe events. The fprobe event is a new dynamic events which is only for the function (symbol) entry and exit. This event accepts non register fetch arguments so that user can trace the function arguments and return values. The fprobe events syntax is here; f[:[GRP/][EVENT]] FUNCTION [FETCHARGS] f[MAXACTIVE][:[GRP/][EVENT]] FUNCTION%return [FETCHARGS] E.g. # echo 'f vfs_read $arg1' >> dynamic_events # echo 'f vfs_read%return $retval' >> dynamic_events # cat dynamic_events f:fprobes/vfs_read__entry vfs_read arg1=$arg1 f:fprobes/vfs_read__exit vfs_read%return arg1=$retval # echo 1 > events/fprobes/enable # head -n 20 trace | tail # TASK-PID CPU# ||||| TIMESTAMP FUNCTION # | | | ||||| | | sh-142 [005] ...1. 448.386420: vfs_read__entry: (vfs_read+0x4/0x340) arg1=0xffff888007f7c540 sh-142 [005] ..... 448.386436: vfs_read__exit: (ksys_read+0x75/0x100 <- vfs_read) arg1=0x1 sh-142 [005] ...1. 448.386451: vfs_read__entry: (vfs_read+0x4/0x340) arg1=0xffff888007f7c540 sh-142 [005] ..... 448.386458: vfs_read__exit: (ksys_read+0x75/0x100 <- vfs_read) arg1=0x1 sh-142 [005] ...1. 448.386469: vfs_read__entry: (vfs_read+0x4/0x340) arg1=0xffff888007f7c540 sh-142 [005] ..... 448.386476: vfs_read__exit: (ksys_read+0x75/0x100 <- vfs_read) arg1=0x1 sh-142 [005] ...1. 448.602073: vfs_read__entry: (vfs_read+0x4/0x340) arg1=0xffff888007f7c540 sh-142 [005] ..... 448.602089: vfs_read__exit: (ksys_read+0x75/0x100 <- vfs_read) arg1=0x1 Link: https://lore.kernel.org/all/168507469754.913472.6112857614708350210.stgit@mhiramat.roam.corp.google.com/ Reported-by: kernel test robot <lkp@intel.com> Link: https://lore.kernel.org/all/202302011530.7vm4O8Ro-lkp@intel.com/ Signed-off-by: Masami Hiramatsu (Google) <mhiramat@kernel.org>
118 lines
3.1 KiB
C
118 lines
3.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/* Simple ftrace probe wrapper */
|
|
#ifndef _LINUX_FPROBE_H
|
|
#define _LINUX_FPROBE_H
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/ftrace.h>
|
|
#include <linux/rethook.h>
|
|
|
|
/**
|
|
* struct fprobe - ftrace based probe.
|
|
* @ops: The ftrace_ops.
|
|
* @nmissed: The counter for missing events.
|
|
* @flags: The status flag.
|
|
* @rethook: The rethook data structure. (internal data)
|
|
* @entry_data_size: The private data storage size.
|
|
* @nr_maxactive: The max number of active functions.
|
|
* @entry_handler: The callback function for function entry.
|
|
* @exit_handler: The callback function for function exit.
|
|
*/
|
|
struct fprobe {
|
|
#ifdef CONFIG_FUNCTION_TRACER
|
|
/*
|
|
* If CONFIG_FUNCTION_TRACER is not set, CONFIG_FPROBE is disabled too.
|
|
* But user of fprobe may keep embedding the struct fprobe on their own
|
|
* code. To avoid build error, this will keep the fprobe data structure
|
|
* defined here, but remove ftrace_ops data structure.
|
|
*/
|
|
struct ftrace_ops ops;
|
|
#endif
|
|
unsigned long nmissed;
|
|
unsigned int flags;
|
|
struct rethook *rethook;
|
|
size_t entry_data_size;
|
|
int nr_maxactive;
|
|
|
|
int (*entry_handler)(struct fprobe *fp, unsigned long entry_ip,
|
|
unsigned long ret_ip, struct pt_regs *regs,
|
|
void *entry_data);
|
|
void (*exit_handler)(struct fprobe *fp, unsigned long entry_ip,
|
|
unsigned long ret_ip, struct pt_regs *regs,
|
|
void *entry_data);
|
|
};
|
|
|
|
/* This fprobe is soft-disabled. */
|
|
#define FPROBE_FL_DISABLED 1
|
|
|
|
/*
|
|
* This fprobe handler will be shared with kprobes.
|
|
* This flag must be set before registering.
|
|
*/
|
|
#define FPROBE_FL_KPROBE_SHARED 2
|
|
|
|
static inline bool fprobe_disabled(struct fprobe *fp)
|
|
{
|
|
return (fp) ? fp->flags & FPROBE_FL_DISABLED : false;
|
|
}
|
|
|
|
static inline bool fprobe_shared_with_kprobes(struct fprobe *fp)
|
|
{
|
|
return (fp) ? fp->flags & FPROBE_FL_KPROBE_SHARED : false;
|
|
}
|
|
|
|
#ifdef CONFIG_FPROBE
|
|
int register_fprobe(struct fprobe *fp, const char *filter, const char *notfilter);
|
|
int register_fprobe_ips(struct fprobe *fp, unsigned long *addrs, int num);
|
|
int register_fprobe_syms(struct fprobe *fp, const char **syms, int num);
|
|
int unregister_fprobe(struct fprobe *fp);
|
|
bool fprobe_is_registered(struct fprobe *fp);
|
|
#else
|
|
static inline int register_fprobe(struct fprobe *fp, const char *filter, const char *notfilter)
|
|
{
|
|
return -EOPNOTSUPP;
|
|
}
|
|
static inline int register_fprobe_ips(struct fprobe *fp, unsigned long *addrs, int num)
|
|
{
|
|
return -EOPNOTSUPP;
|
|
}
|
|
static inline int register_fprobe_syms(struct fprobe *fp, const char **syms, int num)
|
|
{
|
|
return -EOPNOTSUPP;
|
|
}
|
|
static inline int unregister_fprobe(struct fprobe *fp)
|
|
{
|
|
return -EOPNOTSUPP;
|
|
}
|
|
static inline bool fprobe_is_registered(struct fprobe *fp)
|
|
{
|
|
return false;
|
|
}
|
|
#endif
|
|
|
|
/**
|
|
* disable_fprobe() - Disable fprobe
|
|
* @fp: The fprobe to be disabled.
|
|
*
|
|
* This will soft-disable @fp. Note that this doesn't remove the ftrace
|
|
* hooks from the function entry.
|
|
*/
|
|
static inline void disable_fprobe(struct fprobe *fp)
|
|
{
|
|
if (fp)
|
|
fp->flags |= FPROBE_FL_DISABLED;
|
|
}
|
|
|
|
/**
|
|
* enable_fprobe() - Enable fprobe
|
|
* @fp: The fprobe to be enabled.
|
|
*
|
|
* This will soft-enable @fp.
|
|
*/
|
|
static inline void enable_fprobe(struct fprobe *fp)
|
|
{
|
|
if (fp)
|
|
fp->flags &= ~FPROBE_FL_DISABLED;
|
|
}
|
|
|
|
#endif
|