mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
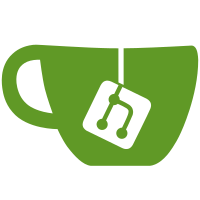
PXA and StrongARM1100 traditionally map their I/O space 1:1 into virtual memory, using a per-bus io_offset that matches the base address of the ioremap mapping. In order for PXA to work in a multiplatform config, this needs to change so I/O space starts at PCI_IOBASE (0xfee00000). Since the pcmcia soc_common support is shared with StrongARM1100, both have to change at the same time. The affected machines are: - Anything with a PCMCIA slot now uses pci_remap_iospace, which is made available to PCMCIA configurations as well, rather than just PCI. The first PCMCIA slot now starts at port number 0x10000. - The Zeus and Viper platforms have PC/104-style ISA buses, which have a static mapping for both I/O and memory space at 0xf1000000, which can no longer work. It does not appear to have any in-tree users, so moving it to port number 0 makes them behave like a traditional PC. - SA1100 does support ISA slots in theory, but all machines that originally enabled this appear to have been removed from the tree ages ago, and the I/O space is never mapped anywhere. - The Nanoengine machine has support for PCI slots, but looks like this never included I/O space, the resources only define the location for memory and config space. With this, the definitions of __io() and IO_SPACE_LIMIT can be simplified, as the only remaining cases are the generic PCI_IOBASE and the custom inb()/outb() macros on RiscPC. S3C24xx still has a custom inb()/outb() in this here, but this is already removed in another branch. Signed-off-by: Arnd Bergmann <arnd@arndb.de>
125 lines
2.8 KiB
C
125 lines
2.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#include <pcmcia/ss.h>
|
|
|
|
struct module;
|
|
struct cpufreq_freqs;
|
|
|
|
struct soc_pcmcia_regulator {
|
|
struct regulator *reg;
|
|
bool on;
|
|
};
|
|
|
|
struct pcmcia_state {
|
|
unsigned detect: 1,
|
|
ready: 1,
|
|
bvd1: 1,
|
|
bvd2: 1,
|
|
wrprot: 1,
|
|
vs_3v: 1,
|
|
vs_Xv: 1;
|
|
};
|
|
|
|
/*
|
|
* This structure encapsulates per-socket state which we might need to
|
|
* use when responding to a Card Services query of some kind.
|
|
*/
|
|
struct soc_pcmcia_socket {
|
|
struct pcmcia_socket socket;
|
|
|
|
/*
|
|
* Info from low level handler
|
|
*/
|
|
unsigned int nr;
|
|
struct clk *clk;
|
|
|
|
/*
|
|
* Core PCMCIA state
|
|
*/
|
|
const struct pcmcia_low_level *ops;
|
|
|
|
unsigned int status;
|
|
socket_state_t cs_state;
|
|
|
|
unsigned short spd_io[MAX_IO_WIN];
|
|
unsigned short spd_mem[MAX_WIN];
|
|
unsigned short spd_attr[MAX_WIN];
|
|
|
|
struct resource res_skt;
|
|
struct resource res_io;
|
|
struct resource res_io_io;
|
|
struct resource res_mem;
|
|
struct resource res_attr;
|
|
|
|
struct {
|
|
int gpio;
|
|
struct gpio_desc *desc;
|
|
unsigned int irq;
|
|
const char *name;
|
|
} stat[6];
|
|
#define SOC_STAT_CD 0 /* Card detect */
|
|
#define SOC_STAT_BVD1 1 /* BATDEAD / IOSTSCHG */
|
|
#define SOC_STAT_BVD2 2 /* BATWARN / IOSPKR */
|
|
#define SOC_STAT_RDY 3 /* Ready / Interrupt */
|
|
#define SOC_STAT_VS1 4 /* Voltage sense 1 */
|
|
#define SOC_STAT_VS2 5 /* Voltage sense 2 */
|
|
|
|
struct gpio_desc *gpio_reset;
|
|
struct gpio_desc *gpio_bus_enable;
|
|
struct soc_pcmcia_regulator vcc;
|
|
struct soc_pcmcia_regulator vpp;
|
|
|
|
unsigned int irq_state;
|
|
|
|
#ifdef CONFIG_CPU_FREQ
|
|
struct notifier_block cpufreq_nb;
|
|
#endif
|
|
struct timer_list poll_timer;
|
|
struct list_head node;
|
|
void *driver_data;
|
|
};
|
|
|
|
|
|
struct pcmcia_low_level {
|
|
struct module *owner;
|
|
|
|
/* first socket in system */
|
|
int first;
|
|
/* nr of sockets */
|
|
int nr;
|
|
|
|
int (*hw_init)(struct soc_pcmcia_socket *);
|
|
void (*hw_shutdown)(struct soc_pcmcia_socket *);
|
|
|
|
void (*socket_state)(struct soc_pcmcia_socket *, struct pcmcia_state *);
|
|
int (*configure_socket)(struct soc_pcmcia_socket *, const socket_state_t *);
|
|
|
|
/*
|
|
* Enable card status IRQs on (re-)initialisation. This can
|
|
* be called at initialisation, power management event, or
|
|
* pcmcia event.
|
|
*/
|
|
void (*socket_init)(struct soc_pcmcia_socket *);
|
|
|
|
/*
|
|
* Disable card status IRQs and PCMCIA bus on suspend.
|
|
*/
|
|
void (*socket_suspend)(struct soc_pcmcia_socket *);
|
|
|
|
/*
|
|
* Hardware specific timing routines.
|
|
* If provided, the get_timing routine overrides the SOC default.
|
|
*/
|
|
unsigned int (*get_timing)(struct soc_pcmcia_socket *, unsigned int, unsigned int);
|
|
int (*set_timing)(struct soc_pcmcia_socket *);
|
|
int (*show_timing)(struct soc_pcmcia_socket *, char *);
|
|
|
|
#ifdef CONFIG_CPU_FREQ
|
|
/*
|
|
* CPUFREQ support.
|
|
*/
|
|
int (*frequency_change)(struct soc_pcmcia_socket *, unsigned long, struct cpufreq_freqs *);
|
|
#endif
|
|
};
|
|
|
|
|
|
|