mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-28 13:22:57 +00:00
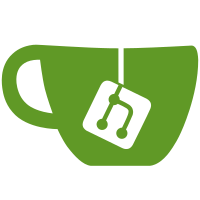
Repair might encounter an inode with a totally garbage i_mode. To fix this problem, we have to figure out if the file was a regular file, a directory, or a special file. One way to figure this out is to check if there are any directories with entries pointing down to the busted file. This patch recovers the file mode by scanning every directory entry on the filesystem to see if there are any that point to the busted file. If the ftype of all such dirents are consistent, the mode is recovered from the ftype. If no dirents are found, the file becomes a regular file. In all cases, ACLs are canceled and the file is made accessible only by root. A previous patch attempted to guess the mode by reading the beginning of the file data. This was rejected by Christoph on the grounds that we cannot trust user-controlled data blocks. Users do not have direct control over the ondisk contents of directory entries, so this method should be much safer. If all the dirents have the same ftype, then we can translate that back into an S_IFMT flag and fix the file. If not, reset the mode to S_IFREG. Signed-off-by: Darrick J. Wong <djwong@kernel.org> Reviewed-by: Christoph Hellwig <hch@lst.de>
84 lines
2.5 KiB
C
84 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* Copyright (c) 2021-2024 Oracle. All Rights Reserved.
|
|
* Author: Darrick J. Wong <djwong@kernel.org>
|
|
*/
|
|
#ifndef __XFS_SCRUB_ISCAN_H__
|
|
#define __XFS_SCRUB_ISCAN_H__
|
|
|
|
struct xchk_iscan {
|
|
struct xfs_scrub *sc;
|
|
|
|
/* Lock to protect the scan cursor. */
|
|
struct mutex lock;
|
|
|
|
/*
|
|
* This is the first inode in the inumber address space that we
|
|
* examined. When the scan wraps around back to here, the scan is
|
|
* finished.
|
|
*/
|
|
xfs_ino_t scan_start_ino;
|
|
|
|
/* This is the inode that will be examined next. */
|
|
xfs_ino_t cursor_ino;
|
|
|
|
/* If nonzero and non-NULL, skip this inode when scanning. */
|
|
xfs_ino_t skip_ino;
|
|
|
|
/*
|
|
* This is the last inode that we've successfully scanned, either
|
|
* because the caller scanned it, or we moved the cursor past an empty
|
|
* part of the inode address space. Scan callers should only use the
|
|
* xchk_iscan_visit function to modify this.
|
|
*/
|
|
xfs_ino_t __visited_ino;
|
|
|
|
/* Operational state of the livescan. */
|
|
unsigned long __opstate;
|
|
|
|
/* Give up on iterating @cursor_ino if we can't iget it by this time. */
|
|
unsigned long __iget_deadline;
|
|
|
|
/* Amount of time (in ms) that we will try to iget an inode. */
|
|
unsigned int iget_timeout;
|
|
|
|
/* Wait this many ms to retry an iget. */
|
|
unsigned int iget_retry_delay;
|
|
|
|
/*
|
|
* The scan grabs batches of inodes and stashes them here before
|
|
* handing them out with _iter. Unallocated inodes are set in the
|
|
* mask so that all updates to that inode are selected for live
|
|
* update propagation.
|
|
*/
|
|
xfs_ino_t __batch_ino;
|
|
xfs_inofree_t __skipped_inomask;
|
|
struct xfs_inode *__inodes[XFS_INODES_PER_CHUNK];
|
|
};
|
|
|
|
/* Set if the scan has been aborted due to some event in the fs. */
|
|
#define XCHK_ISCAN_OPSTATE_ABORTED (1)
|
|
|
|
static inline bool
|
|
xchk_iscan_aborted(const struct xchk_iscan *iscan)
|
|
{
|
|
return test_bit(XCHK_ISCAN_OPSTATE_ABORTED, &iscan->__opstate);
|
|
}
|
|
|
|
static inline void
|
|
xchk_iscan_abort(struct xchk_iscan *iscan)
|
|
{
|
|
set_bit(XCHK_ISCAN_OPSTATE_ABORTED, &iscan->__opstate);
|
|
}
|
|
|
|
void xchk_iscan_start(struct xfs_scrub *sc, unsigned int iget_timeout,
|
|
unsigned int iget_retry_delay, struct xchk_iscan *iscan);
|
|
void xchk_iscan_teardown(struct xchk_iscan *iscan);
|
|
|
|
int xchk_iscan_iter(struct xchk_iscan *iscan, struct xfs_inode **ipp);
|
|
void xchk_iscan_iter_finish(struct xchk_iscan *iscan);
|
|
|
|
void xchk_iscan_mark_visited(struct xchk_iscan *iscan, struct xfs_inode *ip);
|
|
bool xchk_iscan_want_live_update(struct xchk_iscan *iscan, xfs_ino_t ino);
|
|
|
|
#endif /* __XFS_SCRUB_ISCAN_H__ */
|