mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-14 06:35:12 +00:00
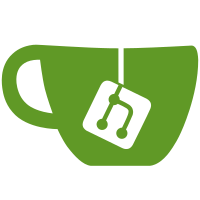
This makes all those 'unsigned char's an explicit 'u8'. This is part of the continuing unification of chars and flags to be consistent u8. This approaches tty_port_default_receive_buf(). Flags to be next. Signed-off-by: "Jiri Slaby (SUSE)" <jirislaby@kernel.org> Cc: William Hubbs <w.d.hubbs@gmail.com> Cc: Chris Brannon <chris@the-brannons.com> Cc: Kirk Reiser <kirk@reisers.ca> Cc: Samuel Thibault <samuel.thibault@ens-lyon.org> Cc: Dmitry Torokhov <dmitry.torokhov@gmail.com> Cc: Arnd Bergmann <arnd@arndb.de> Cc: Max Staudt <max@enpas.org> Cc: Wolfgang Grandegger <wg@grandegger.com> Cc: Marc Kleine-Budde <mkl@pengutronix.de> Cc: "David S. Miller" <davem@davemloft.net> Cc: Eric Dumazet <edumazet@google.com> Cc: Jakub Kicinski <kuba@kernel.org> Cc: Paolo Abeni <pabeni@redhat.com> Cc: Dario Binacchi <dario.binacchi@amarulasolutions.com> Cc: Andreas Koensgen <ajk@comnets.uni-bremen.de> Cc: Jeremy Kerr <jk@codeconstruct.com.au> Cc: Matt Johnston <matt@codeconstruct.com.au> Cc: Liam Girdwood <lgirdwood@gmail.com> Cc: Mark Brown <broonie@kernel.org> Cc: Jaroslav Kysela <perex@perex.cz> Cc: Takashi Iwai <tiwai@suse.com> Cc: Peter Ujfalusi <peter.ujfalusi@gmail.com> Acked-by: Mark Brown <broonie@kernel.org> Link: https://lore.kernel.org/r/20230810091510.13006-17-jirislaby@kernel.org Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
57 lines
1.2 KiB
C
57 lines
1.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_TTY_BUFFER_H
|
|
#define _LINUX_TTY_BUFFER_H
|
|
|
|
#include <linux/atomic.h>
|
|
#include <linux/llist.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/workqueue.h>
|
|
|
|
struct tty_buffer {
|
|
union {
|
|
struct tty_buffer *next;
|
|
struct llist_node free;
|
|
};
|
|
int used;
|
|
int size;
|
|
int commit;
|
|
int lookahead; /* Lazy update on recv, can become less than "read" */
|
|
int read;
|
|
bool flags;
|
|
/* Data points here */
|
|
unsigned long data[];
|
|
};
|
|
|
|
static inline u8 *char_buf_ptr(struct tty_buffer *b, int ofs)
|
|
{
|
|
return ((u8 *)b->data) + ofs;
|
|
}
|
|
|
|
static inline char *flag_buf_ptr(struct tty_buffer *b, int ofs)
|
|
{
|
|
return (char *)char_buf_ptr(b, ofs) + b->size;
|
|
}
|
|
|
|
struct tty_bufhead {
|
|
struct tty_buffer *head; /* Queue head */
|
|
struct work_struct work;
|
|
struct mutex lock;
|
|
atomic_t priority;
|
|
struct tty_buffer sentinel;
|
|
struct llist_head free; /* Free queue head */
|
|
atomic_t mem_used; /* In-use buffers excluding free list */
|
|
int mem_limit;
|
|
struct tty_buffer *tail; /* Active buffer */
|
|
};
|
|
|
|
/*
|
|
* When a break, frame error, or parity error happens, these codes are
|
|
* stuffed into the flags buffer.
|
|
*/
|
|
#define TTY_NORMAL 0
|
|
#define TTY_BREAK 1
|
|
#define TTY_FRAME 2
|
|
#define TTY_PARITY 3
|
|
#define TTY_OVERRUN 4
|
|
|
|
#endif
|