mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-14 06:35:12 +00:00
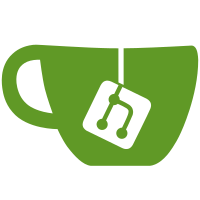
Currently when restoring the SVE state we supply the SVE vector length as an argument to sve_load_state() and the underlying macros. This becomes inconvenient with the addition of SME since we may need to restore any combination of SVE and SME vector lengths, and we already separately restore the vector length in the KVM code. We don't need to know the vector length during the actual register load since the SME load instructions can index into the data array for us. Refactor the interface so we explicitly set the vector length separately to restoring the SVE registers in preparation for adding SME support, no functional change should be involved. Signed-off-by: Mark Brown <broonie@kernel.org> Link: https://lore.kernel.org/r/20211019172247.3045838-9-broonie@kernel.org Signed-off-by: Will Deacon <will@kernel.org>
88 lines
1.6 KiB
ArmAsm
88 lines
1.6 KiB
ArmAsm
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* FP/SIMD state saving and restoring
|
|
*
|
|
* Copyright (C) 2012 ARM Ltd.
|
|
* Author: Catalin Marinas <catalin.marinas@arm.com>
|
|
*/
|
|
|
|
#include <linux/linkage.h>
|
|
|
|
#include <asm/assembler.h>
|
|
#include <asm/fpsimdmacros.h>
|
|
|
|
/*
|
|
* Save the FP registers.
|
|
*
|
|
* x0 - pointer to struct fpsimd_state
|
|
*/
|
|
SYM_FUNC_START(fpsimd_save_state)
|
|
fpsimd_save x0, 8
|
|
ret
|
|
SYM_FUNC_END(fpsimd_save_state)
|
|
|
|
/*
|
|
* Load the FP registers.
|
|
*
|
|
* x0 - pointer to struct fpsimd_state
|
|
*/
|
|
SYM_FUNC_START(fpsimd_load_state)
|
|
fpsimd_restore x0, 8
|
|
ret
|
|
SYM_FUNC_END(fpsimd_load_state)
|
|
|
|
#ifdef CONFIG_ARM64_SVE
|
|
|
|
/*
|
|
* Save the SVE state
|
|
*
|
|
* x0 - pointer to buffer for state
|
|
* x1 - pointer to storage for FPSR
|
|
* x2 - Save FFR if non-zero
|
|
*/
|
|
SYM_FUNC_START(sve_save_state)
|
|
sve_save 0, x1, x2, 3
|
|
ret
|
|
SYM_FUNC_END(sve_save_state)
|
|
|
|
/*
|
|
* Load the SVE state
|
|
*
|
|
* x0 - pointer to buffer for state
|
|
* x1 - pointer to storage for FPSR
|
|
* x2 - Restore FFR if non-zero
|
|
*/
|
|
SYM_FUNC_START(sve_load_state)
|
|
sve_load 0, x1, x2, 4
|
|
ret
|
|
SYM_FUNC_END(sve_load_state)
|
|
|
|
SYM_FUNC_START(sve_get_vl)
|
|
_sve_rdvl 0, 1
|
|
ret
|
|
SYM_FUNC_END(sve_get_vl)
|
|
|
|
SYM_FUNC_START(sve_set_vq)
|
|
sve_load_vq x0, x1, x2
|
|
ret
|
|
SYM_FUNC_END(sve_set_vq)
|
|
|
|
/*
|
|
* Zero all SVE registers but the first 128-bits of each vector
|
|
*
|
|
* VQ must already be configured by caller, any further updates of VQ
|
|
* will need to ensure that the register state remains valid.
|
|
*
|
|
* x0 = include FFR?
|
|
* x1 = VQ - 1
|
|
*/
|
|
SYM_FUNC_START(sve_flush_live)
|
|
cbz x1, 1f // A VQ-1 of 0 is 128 bits so no extra Z state
|
|
sve_flush_z
|
|
1: sve_flush_p
|
|
tbz x0, #0, 2f
|
|
sve_flush_ffr
|
|
2: ret
|
|
SYM_FUNC_END(sve_flush_live)
|
|
|
|
#endif /* CONFIG_ARM64_SVE */
|