mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-14 06:35:12 +00:00
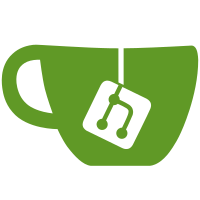
The intended semantics of pfn_valid() is to verify whether there is a struct page for the pfn in question and nothing else. Yet, on arm64 it is used to distinguish memory areas that are mapped in the linear map vs those that require ioremap() to access them. Introduce a dedicated pfn_is_map_memory() wrapper for memblock_is_map_memory() to perform such check and use it where appropriate. Using a wrapper allows to avoid cyclic include dependencies. While here also update style of pfn_valid() so that both pfn_valid() and pfn_is_map_memory() declarations will be consistent. Link: https://lkml.kernel.org/r/20210511100550.28178-4-rppt@kernel.org Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Acked-by: David Hildenbrand <david@redhat.com> Acked-by: Ard Biesheuvel <ardb@kernel.org> Reviewed-by: Kefeng Wang <wangkefeng.wang@huawei.com> Cc: Anshuman Khandual <anshuman.khandual@arm.com> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Marc Zyngier <maz@kernel.org> Cc: Mark Rutland <mark.rutland@arm.com> Cc: Will Deacon <will@kernel.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
101 lines
2.4 KiB
C
101 lines
2.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Based on arch/arm/mm/ioremap.c
|
|
*
|
|
* (C) Copyright 1995 1996 Linus Torvalds
|
|
* Hacked for ARM by Phil Blundell <philb@gnu.org>
|
|
* Hacked to allow all architectures to build, and various cleanups
|
|
* by Russell King
|
|
* Copyright (C) 2012 ARM Ltd.
|
|
*/
|
|
|
|
#include <linux/export.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/vmalloc.h>
|
|
#include <linux/io.h>
|
|
|
|
#include <asm/fixmap.h>
|
|
#include <asm/tlbflush.h>
|
|
|
|
static void __iomem *__ioremap_caller(phys_addr_t phys_addr, size_t size,
|
|
pgprot_t prot, void *caller)
|
|
{
|
|
unsigned long last_addr;
|
|
unsigned long offset = phys_addr & ~PAGE_MASK;
|
|
int err;
|
|
unsigned long addr;
|
|
struct vm_struct *area;
|
|
|
|
/*
|
|
* Page align the mapping address and size, taking account of any
|
|
* offset.
|
|
*/
|
|
phys_addr &= PAGE_MASK;
|
|
size = PAGE_ALIGN(size + offset);
|
|
|
|
/*
|
|
* Don't allow wraparound, zero size or outside PHYS_MASK.
|
|
*/
|
|
last_addr = phys_addr + size - 1;
|
|
if (!size || last_addr < phys_addr || (last_addr & ~PHYS_MASK))
|
|
return NULL;
|
|
|
|
/*
|
|
* Don't allow RAM to be mapped.
|
|
*/
|
|
if (WARN_ON(pfn_is_map_memory(__phys_to_pfn(phys_addr))))
|
|
return NULL;
|
|
|
|
area = get_vm_area_caller(size, VM_IOREMAP, caller);
|
|
if (!area)
|
|
return NULL;
|
|
addr = (unsigned long)area->addr;
|
|
area->phys_addr = phys_addr;
|
|
|
|
err = ioremap_page_range(addr, addr + size, phys_addr, prot);
|
|
if (err) {
|
|
vunmap((void *)addr);
|
|
return NULL;
|
|
}
|
|
|
|
return (void __iomem *)(offset + addr);
|
|
}
|
|
|
|
void __iomem *__ioremap(phys_addr_t phys_addr, size_t size, pgprot_t prot)
|
|
{
|
|
return __ioremap_caller(phys_addr, size, prot,
|
|
__builtin_return_address(0));
|
|
}
|
|
EXPORT_SYMBOL(__ioremap);
|
|
|
|
void iounmap(volatile void __iomem *io_addr)
|
|
{
|
|
unsigned long addr = (unsigned long)io_addr & PAGE_MASK;
|
|
|
|
/*
|
|
* We could get an address outside vmalloc range in case
|
|
* of ioremap_cache() reusing a RAM mapping.
|
|
*/
|
|
if (is_vmalloc_addr((void *)addr))
|
|
vunmap((void *)addr);
|
|
}
|
|
EXPORT_SYMBOL(iounmap);
|
|
|
|
void __iomem *ioremap_cache(phys_addr_t phys_addr, size_t size)
|
|
{
|
|
/* For normal memory we already have a cacheable mapping. */
|
|
if (pfn_is_map_memory(__phys_to_pfn(phys_addr)))
|
|
return (void __iomem *)__phys_to_virt(phys_addr);
|
|
|
|
return __ioremap_caller(phys_addr, size, __pgprot(PROT_NORMAL),
|
|
__builtin_return_address(0));
|
|
}
|
|
EXPORT_SYMBOL(ioremap_cache);
|
|
|
|
/*
|
|
* Must be called after early_fixmap_init
|
|
*/
|
|
void __init early_ioremap_init(void)
|
|
{
|
|
early_ioremap_setup();
|
|
}
|