mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 23:25:07 +00:00
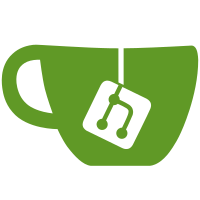
There is no evidence of officially registered ACPI IDs for these devices. Thus, revert ACPI support from the driver. All authors of the respective changes are being informed here:d5c94568cc
("iio: add bmp280 pressure and temperature driver")6dba72eca7
("iio: pressure: bmp280: add support for BMP180")14beaa8f5a
("iio: pressure: bmp280: add humidity support") Above seems a cargo cult without paying attention to how ACPI IDs are being allocated. Cc: Vlad Dogaru <ddvlad@gmail.com> Cc: Akinobu Mita <akinobu.mita@gmail.com> Cc: Matt Ranostay <mranostay@gmail.com> Signed-off-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com> Signed-off-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
75 lines
1.9 KiB
C
75 lines
1.9 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
#include <linux/module.h>
|
|
#include <linux/i2c.h>
|
|
#include <linux/of.h>
|
|
#include <linux/regmap.h>
|
|
|
|
#include "bmp280.h"
|
|
|
|
static int bmp280_i2c_probe(struct i2c_client *client,
|
|
const struct i2c_device_id *id)
|
|
{
|
|
struct regmap *regmap;
|
|
const struct regmap_config *regmap_config;
|
|
|
|
switch (id->driver_data) {
|
|
case BMP180_CHIP_ID:
|
|
regmap_config = &bmp180_regmap_config;
|
|
break;
|
|
case BMP280_CHIP_ID:
|
|
case BME280_CHIP_ID:
|
|
regmap_config = &bmp280_regmap_config;
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
regmap = devm_regmap_init_i2c(client, regmap_config);
|
|
if (IS_ERR(regmap)) {
|
|
dev_err(&client->dev, "failed to allocate register map\n");
|
|
return PTR_ERR(regmap);
|
|
}
|
|
|
|
return bmp280_common_probe(&client->dev,
|
|
regmap,
|
|
id->driver_data,
|
|
id->name,
|
|
client->irq);
|
|
}
|
|
|
|
#ifdef CONFIG_OF
|
|
static const struct of_device_id bmp280_of_i2c_match[] = {
|
|
{ .compatible = "bosch,bme280", .data = (void *)BME280_CHIP_ID },
|
|
{ .compatible = "bosch,bmp280", .data = (void *)BMP280_CHIP_ID },
|
|
{ .compatible = "bosch,bmp180", .data = (void *)BMP180_CHIP_ID },
|
|
{ .compatible = "bosch,bmp085", .data = (void *)BMP180_CHIP_ID },
|
|
{ },
|
|
};
|
|
MODULE_DEVICE_TABLE(of, bmp280_of_i2c_match);
|
|
#else
|
|
#define bmp280_of_i2c_match NULL
|
|
#endif
|
|
|
|
static const struct i2c_device_id bmp280_i2c_id[] = {
|
|
{"bmp280", BMP280_CHIP_ID },
|
|
{"bmp180", BMP180_CHIP_ID },
|
|
{"bmp085", BMP180_CHIP_ID },
|
|
{"bme280", BME280_CHIP_ID },
|
|
{ },
|
|
};
|
|
MODULE_DEVICE_TABLE(i2c, bmp280_i2c_id);
|
|
|
|
static struct i2c_driver bmp280_i2c_driver = {
|
|
.driver = {
|
|
.name = "bmp280",
|
|
.of_match_table = of_match_ptr(bmp280_of_i2c_match),
|
|
.pm = &bmp280_dev_pm_ops,
|
|
},
|
|
.probe = bmp280_i2c_probe,
|
|
.id_table = bmp280_i2c_id,
|
|
};
|
|
module_i2c_driver(bmp280_i2c_driver);
|
|
|
|
MODULE_AUTHOR("Vlad Dogaru <vlad.dogaru@intel.com>");
|
|
MODULE_DESCRIPTION("Driver for Bosch Sensortec BMP180/BMP280 pressure and temperature sensor");
|
|
MODULE_LICENSE("GPL v2");
|