mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-22 18:41:06 +00:00
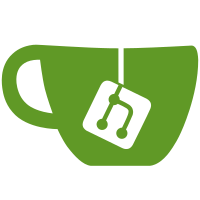
incl_srcpt has the limitation, mentioned in commitb4638af888
("net: dsa: sja1105: always enable the INCL_SRCPT option"), that frames with a MAC DA of 01:80:c2:xx:yy:zz will be received as 01:80:c2:00:00:zz unless PTP RX timestamping is enabled. The incl_srcpt option was initially unconditionally enabled, then that changed with commit42824463d3
("net: dsa: sja1105: Limit use of incl_srcpt to bridge+vlan mode"), then again withb4638af888
("net: dsa: sja1105: always enable the INCL_SRCPT option"). Bottom line is that it now needs to be always enabled, otherwise the driver does not have a reliable source of information regarding source_port and switch_id for link-local traffic (tag_8021q VLANs may be imprecise since now they identify an entire bridging domain when ports are not standalone). If we accept that PTP RX timestamping (and therefore, meta frame generation) is always enabled in hardware, then that limitation could be avoided and packets with any MAC DA can be properly received, because meta frames do contain the original bytes from the MAC DA of their associated link-local packet. This change enables meta frame generation unconditionally, which also has the nice side effects of simplifying the switch control path (a switch reset is no longer required on hwtstamping settings change) and the tagger data path (it no longer needs to be informed whether to expect meta frames or not - it always does). Fixes:227d07a07e
("net: dsa: sja1105: Add support for traffic through standalone ports") Signed-off-by: Vladimir Oltean <vladimir.oltean@nxp.com> Reviewed-by: Simon Horman <simon.horman@corigine.com> Reviewed-by: Florian Fainelli <florian.fainelli@broadcom.com> Signed-off-by: David S. Miller <davem@davemloft.net>
75 lines
2.2 KiB
C
75 lines
2.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0
|
|
* Copyright (c) 2019, Vladimir Oltean <olteanv@gmail.com>
|
|
*/
|
|
|
|
/* Included by drivers/net/dsa/sja1105/sja1105.h and net/dsa/tag_sja1105.c */
|
|
|
|
#ifndef _NET_DSA_SJA1105_H
|
|
#define _NET_DSA_SJA1105_H
|
|
|
|
#include <linux/skbuff.h>
|
|
#include <linux/etherdevice.h>
|
|
#include <linux/dsa/8021q.h>
|
|
#include <net/dsa.h>
|
|
|
|
#define ETH_P_SJA1105 ETH_P_DSA_8021Q
|
|
#define ETH_P_SJA1105_META 0x0008
|
|
#define ETH_P_SJA1110 0xdadc
|
|
|
|
#define SJA1105_DEFAULT_VLAN (VLAN_N_VID - 1)
|
|
|
|
/* IEEE 802.3 Annex 57A: Slow Protocols PDUs (01:80:C2:xx:xx:xx) */
|
|
#define SJA1105_LINKLOCAL_FILTER_A 0x0180C2000000ull
|
|
#define SJA1105_LINKLOCAL_FILTER_A_MASK 0xFFFFFF000000ull
|
|
/* IEEE 1588 Annex F: Transport of PTP over Ethernet (01:1B:19:xx:xx:xx) */
|
|
#define SJA1105_LINKLOCAL_FILTER_B 0x011B19000000ull
|
|
#define SJA1105_LINKLOCAL_FILTER_B_MASK 0xFFFFFF000000ull
|
|
|
|
/* Source and Destination MAC of follow-up meta frames.
|
|
* Whereas the choice of SMAC only affects the unique identification of the
|
|
* switch as sender of meta frames, the DMAC must be an address that is present
|
|
* in the DSA master port's multicast MAC filter.
|
|
* 01-80-C2-00-00-0E is a good choice for this, as all profiles of IEEE 1588
|
|
* over L2 use this address for some purpose already.
|
|
*/
|
|
#define SJA1105_META_SMAC 0x222222222222ull
|
|
#define SJA1105_META_DMAC 0x0180C200000Eull
|
|
|
|
enum sja1110_meta_tstamp {
|
|
SJA1110_META_TSTAMP_TX = 0,
|
|
SJA1110_META_TSTAMP_RX = 1,
|
|
};
|
|
|
|
struct sja1105_deferred_xmit_work {
|
|
struct dsa_port *dp;
|
|
struct sk_buff *skb;
|
|
struct kthread_work work;
|
|
};
|
|
|
|
/* Global tagger data */
|
|
struct sja1105_tagger_data {
|
|
void (*xmit_work_fn)(struct kthread_work *work);
|
|
void (*meta_tstamp_handler)(struct dsa_switch *ds, int port, u8 ts_id,
|
|
enum sja1110_meta_tstamp dir, u64 tstamp);
|
|
};
|
|
|
|
struct sja1105_skb_cb {
|
|
struct sk_buff *clone;
|
|
u64 tstamp;
|
|
/* Only valid for packets cloned for 2-step TX timestamping */
|
|
u8 ts_id;
|
|
};
|
|
|
|
#define SJA1105_SKB_CB(skb) \
|
|
((struct sja1105_skb_cb *)((skb)->cb))
|
|
|
|
static inline struct sja1105_tagger_data *
|
|
sja1105_tagger_data(struct dsa_switch *ds)
|
|
{
|
|
BUG_ON(ds->dst->tag_ops->proto != DSA_TAG_PROTO_SJA1105 &&
|
|
ds->dst->tag_ops->proto != DSA_TAG_PROTO_SJA1110);
|
|
|
|
return ds->tagger_data;
|
|
}
|
|
|
|
#endif /* _NET_DSA_SJA1105_H */
|