mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 23:24:50 +00:00
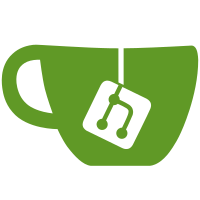
Since BUG() and WARN() may use a trap (e.g. UD2 on x86) to get the address where the BUG() has occurred, kprobes can not do single-step out-of-line that instruction. So prohibit probing on such address. Without this fix, if someone put a kprobe on WARN(), the kernel will crash with invalid opcode error instead of outputing warning message, because kernel can not find correct bug address. Signed-off-by: Masami Hiramatsu <mhiramat@kernel.org> Acked-by: Steven Rostedt (VMware) <rostedt@goodmis.org> Acked-by: Naveen N. Rao <naveen.n.rao@linux.vnet.ibm.com> Cc: Anil S Keshavamurthy <anil.s.keshavamurthy@intel.com> Cc: David S . Miller <davem@davemloft.net> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Naveen N . Rao <naveen.n.rao@linux.ibm.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steven Rostedt <rostedt@goodmis.org> Cc: Thomas Gleixner <tglx@linutronix.de> Link: https://lkml.kernel.org/r/156750890133.19112.3393666300746167111.stgit@devnote2 Signed-off-by: Ingo Molnar <mingo@kernel.org>
84 lines
1.9 KiB
C
84 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_BUG_H
|
|
#define _LINUX_BUG_H
|
|
|
|
#include <asm/bug.h>
|
|
#include <linux/compiler.h>
|
|
#include <linux/build_bug.h>
|
|
|
|
enum bug_trap_type {
|
|
BUG_TRAP_TYPE_NONE = 0,
|
|
BUG_TRAP_TYPE_WARN = 1,
|
|
BUG_TRAP_TYPE_BUG = 2,
|
|
};
|
|
|
|
struct pt_regs;
|
|
|
|
#ifdef __CHECKER__
|
|
#define MAYBE_BUILD_BUG_ON(cond) (0)
|
|
#else /* __CHECKER__ */
|
|
|
|
#define MAYBE_BUILD_BUG_ON(cond) \
|
|
do { \
|
|
if (__builtin_constant_p((cond))) \
|
|
BUILD_BUG_ON(cond); \
|
|
else \
|
|
BUG_ON(cond); \
|
|
} while (0)
|
|
|
|
#endif /* __CHECKER__ */
|
|
|
|
#ifdef CONFIG_GENERIC_BUG
|
|
#include <asm-generic/bug.h>
|
|
|
|
static inline int is_warning_bug(const struct bug_entry *bug)
|
|
{
|
|
return bug->flags & BUGFLAG_WARNING;
|
|
}
|
|
|
|
struct bug_entry *find_bug(unsigned long bugaddr);
|
|
|
|
enum bug_trap_type report_bug(unsigned long bug_addr, struct pt_regs *regs);
|
|
|
|
/* These are defined by the architecture */
|
|
int is_valid_bugaddr(unsigned long addr);
|
|
|
|
void generic_bug_clear_once(void);
|
|
|
|
#else /* !CONFIG_GENERIC_BUG */
|
|
|
|
static inline void *find_bug(unsigned long bugaddr)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static inline enum bug_trap_type report_bug(unsigned long bug_addr,
|
|
struct pt_regs *regs)
|
|
{
|
|
return BUG_TRAP_TYPE_BUG;
|
|
}
|
|
|
|
|
|
static inline void generic_bug_clear_once(void) {}
|
|
|
|
#endif /* CONFIG_GENERIC_BUG */
|
|
|
|
/*
|
|
* Since detected data corruption should stop operation on the affected
|
|
* structures. Return value must be checked and sanely acted on by caller.
|
|
*/
|
|
static inline __must_check bool check_data_corruption(bool v) { return v; }
|
|
#define CHECK_DATA_CORRUPTION(condition, fmt, ...) \
|
|
check_data_corruption(({ \
|
|
bool corruption = unlikely(condition); \
|
|
if (corruption) { \
|
|
if (IS_ENABLED(CONFIG_BUG_ON_DATA_CORRUPTION)) { \
|
|
pr_err(fmt, ##__VA_ARGS__); \
|
|
BUG(); \
|
|
} else \
|
|
WARN(1, fmt, ##__VA_ARGS__); \
|
|
} \
|
|
corruption; \
|
|
}))
|
|
|
|
#endif /* _LINUX_BUG_H */
|