mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 08:19:28 +00:00
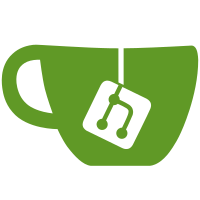
There are place holder functions in the IPA code that do nothing. For the most part these are inverse functions, for example, once the routing or filter tables are set up there is no need to perform any matching teardown activity at shutdown, or in the case of an error. These can be safely removed, resulting in some code simplification. Add comments in these spots making it explicit that there is no inverse. Signed-off-by: Alex Elder <elder@linaro.org> Signed-off-by: Jakub Kicinski <kuba@kernel.org>
102 lines
2.3 KiB
C
102 lines
2.3 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
|
|
/* Copyright (c) 2012-2018, The Linux Foundation. All rights reserved.
|
|
* Copyright (C) 2019-2021 Linaro Ltd.
|
|
*/
|
|
#ifndef _IPA_TABLE_H_
|
|
#define _IPA_TABLE_H_
|
|
|
|
#include <linux/types.h>
|
|
|
|
struct ipa;
|
|
|
|
/* The maximum number of filter table entries (IPv4, IPv6; hashed or not) */
|
|
#define IPA_FILTER_COUNT_MAX 14
|
|
|
|
/* The maximum number of route table entries (IPv4, IPv6; hashed or not) */
|
|
#define IPA_ROUTE_COUNT_MAX 15
|
|
|
|
#ifdef IPA_VALIDATE
|
|
|
|
/**
|
|
* ipa_table_valid() - Validate route and filter table memory regions
|
|
* @ipa: IPA pointer
|
|
*
|
|
* Return: true if all regions are valid, false otherwise
|
|
*/
|
|
bool ipa_table_valid(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_filter_map_valid() - Validate a filter table endpoint bitmap
|
|
* @ipa: IPA pointer
|
|
* @filter_mask: Filter table endpoint bitmap to check
|
|
*
|
|
* Return: true if all regions are valid, false otherwise
|
|
*/
|
|
bool ipa_filter_map_valid(struct ipa *ipa, u32 filter_mask);
|
|
|
|
#else /* !IPA_VALIDATE */
|
|
|
|
static inline bool ipa_table_valid(struct ipa *ipa)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
static inline bool ipa_filter_map_valid(struct ipa *ipa, u32 filter_mask)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
#endif /* !IPA_VALIDATE */
|
|
|
|
/**
|
|
* ipa_table_hash_support() - Return true if hashed tables are supported
|
|
* @ipa: IPA pointer
|
|
*/
|
|
static inline bool ipa_table_hash_support(struct ipa *ipa)
|
|
{
|
|
return ipa->version != IPA_VERSION_4_2;
|
|
}
|
|
|
|
/**
|
|
* ipa_table_reset() - Reset filter and route tables entries to "none"
|
|
* @ipa: IPA pointer
|
|
* @modem: Whether to reset modem or AP entries
|
|
*/
|
|
void ipa_table_reset(struct ipa *ipa, bool modem);
|
|
|
|
/**
|
|
* ipa_table_hash_flush() - Synchronize hashed filter and route updates
|
|
* @ipa: IPA pointer
|
|
*/
|
|
int ipa_table_hash_flush(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_setup() - Set up filter and route tables
|
|
* @ipa: IPA pointer
|
|
*
|
|
* There is no need for a matching ipa_table_teardown() function.
|
|
*/
|
|
int ipa_table_setup(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_config() - Configure filter and route tables
|
|
* @ipa: IPA pointer
|
|
*
|
|
* There is no need for a matching ipa_table_deconfig() function.
|
|
*/
|
|
void ipa_table_config(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_init() - Do early initialization of filter and route tables
|
|
* @ipa: IPA pointer
|
|
*/
|
|
int ipa_table_init(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_exit() - Inverse of ipa_table_init()
|
|
* @ipa: IPA pointer
|
|
*/
|
|
void ipa_table_exit(struct ipa *ipa);
|
|
|
|
#endif /* _IPA_TABLE_H_ */
|