mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
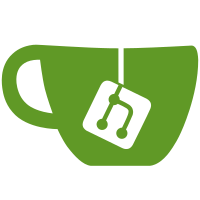
With module.h being implicitly everywhere via device.h, the absence of explicitly including something for EXPORT_SYMBOL went unnoticed. Since we are heading to fix things up and clean module.h from the device.h file, we need to explicitly include these files now. Signed-off-by: Paul Gortmaker <paul.gortmaker@windriver.com>
47 lines
1.2 KiB
C
47 lines
1.2 KiB
C
/*
|
|
* linux/drivers/video/fb_notify.c
|
|
*
|
|
* Copyright (C) 2006 Antonino Daplas <adaplas@pol.net>
|
|
*
|
|
* 2001 - Documented with DocBook
|
|
* - Brad Douglas <brad@neruo.com>
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file COPYING in the main directory of this archive
|
|
* for more details.
|
|
*/
|
|
#include <linux/fb.h>
|
|
#include <linux/notifier.h>
|
|
#include <linux/export.h>
|
|
|
|
static BLOCKING_NOTIFIER_HEAD(fb_notifier_list);
|
|
|
|
/**
|
|
* fb_register_client - register a client notifier
|
|
* @nb: notifier block to callback on events
|
|
*/
|
|
int fb_register_client(struct notifier_block *nb)
|
|
{
|
|
return blocking_notifier_chain_register(&fb_notifier_list, nb);
|
|
}
|
|
EXPORT_SYMBOL(fb_register_client);
|
|
|
|
/**
|
|
* fb_unregister_client - unregister a client notifier
|
|
* @nb: notifier block to callback on events
|
|
*/
|
|
int fb_unregister_client(struct notifier_block *nb)
|
|
{
|
|
return blocking_notifier_chain_unregister(&fb_notifier_list, nb);
|
|
}
|
|
EXPORT_SYMBOL(fb_unregister_client);
|
|
|
|
/**
|
|
* fb_notifier_call_chain - notify clients of fb_events
|
|
*
|
|
*/
|
|
int fb_notifier_call_chain(unsigned long val, void *v)
|
|
{
|
|
return blocking_notifier_call_chain(&fb_notifier_list, val, v);
|
|
}
|
|
EXPORT_SYMBOL_GPL(fb_notifier_call_chain);
|