mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 15:15:47 +00:00
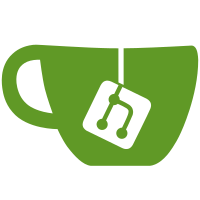
Our PV guest patching code assembles chunks of instructions on the fly when it encounters more complicated instructions to hijack. These instructions need to live in a section that we don't mark as non-executable, as otherwise we fault when jumping there. Right now we put it into the .bss section where it automatically gets marked as non-executable. Add a check to the NX setting function to ensure that we leave these particular pages executable. Signed-off-by: Alexander Graf <agraf@suse.de>
67 lines
1.5 KiB
C
67 lines
1.5 KiB
C
#ifndef _ASM_POWERPC_SECTIONS_H
|
|
#define _ASM_POWERPC_SECTIONS_H
|
|
#ifdef __KERNEL__
|
|
|
|
#include <linux/elf.h>
|
|
#include <linux/uaccess.h>
|
|
#include <asm-generic/sections.h>
|
|
|
|
#ifdef __powerpc64__
|
|
|
|
extern char __start_interrupts[];
|
|
extern char __end_interrupts[];
|
|
|
|
extern char __prom_init_toc_start[];
|
|
extern char __prom_init_toc_end[];
|
|
|
|
static inline int in_kernel_text(unsigned long addr)
|
|
{
|
|
if (addr >= (unsigned long)_stext && addr < (unsigned long)__init_end)
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static inline int overlaps_interrupt_vector_text(unsigned long start,
|
|
unsigned long end)
|
|
{
|
|
unsigned long real_start, real_end;
|
|
real_start = __start_interrupts - _stext;
|
|
real_end = __end_interrupts - _stext;
|
|
|
|
return start < (unsigned long)__va(real_end) &&
|
|
(unsigned long)__va(real_start) < end;
|
|
}
|
|
|
|
static inline int overlaps_kernel_text(unsigned long start, unsigned long end)
|
|
{
|
|
return start < (unsigned long)__init_end &&
|
|
(unsigned long)_stext < end;
|
|
}
|
|
|
|
static inline int overlaps_kvm_tmp(unsigned long start, unsigned long end)
|
|
{
|
|
#ifdef CONFIG_KVM_GUEST
|
|
extern char kvm_tmp[];
|
|
return start < (unsigned long)kvm_tmp &&
|
|
(unsigned long)&kvm_tmp[1024 * 1024] < end;
|
|
#else
|
|
return 0;
|
|
#endif
|
|
}
|
|
|
|
#undef dereference_function_descriptor
|
|
static inline void *dereference_function_descriptor(void *ptr)
|
|
{
|
|
struct ppc64_opd_entry *desc = ptr;
|
|
void *p;
|
|
|
|
if (!probe_kernel_address(&desc->funcaddr, p))
|
|
ptr = p;
|
|
return ptr;
|
|
}
|
|
|
|
#endif
|
|
|
|
#endif /* __KERNEL__ */
|
|
#endif /* _ASM_POWERPC_SECTIONS_H */
|