mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-28 23:24:50 +00:00
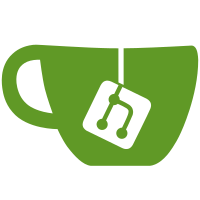
It's problematic to allow signed element_nr's or total's to be passed as part of the flex array API. flex_array_alloc() allows total_nr_elements to be set to a negative quantity, which is obviously erroneous. flex_array_get() and flex_array_put() allows negative array indices in dereferencing an array part, which could address memory mapped before struct flex_array. The fix is to convert all existing element_nr formals to be qualified as unsigned. Existing checks to compare it to total_nr_elements or the max array size based on element_size need not be changed. Signed-off-by: David Rientjes <rientjes@google.com> Cc: Dave Hansen <dave@linux.vnet.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
49 lines
1.2 KiB
C
49 lines
1.2 KiB
C
#ifndef _FLEX_ARRAY_H
|
|
#define _FLEX_ARRAY_H
|
|
|
|
#include <linux/types.h>
|
|
#include <asm/page.h>
|
|
|
|
#define FLEX_ARRAY_PART_SIZE PAGE_SIZE
|
|
#define FLEX_ARRAY_BASE_SIZE PAGE_SIZE
|
|
|
|
struct flex_array_part;
|
|
|
|
/*
|
|
* This is meant to replace cases where an array-like
|
|
* structure has gotten too big to fit into kmalloc()
|
|
* and the developer is getting tempted to use
|
|
* vmalloc().
|
|
*/
|
|
|
|
struct flex_array {
|
|
union {
|
|
struct {
|
|
int element_size;
|
|
int total_nr_elements;
|
|
struct flex_array_part *parts[];
|
|
};
|
|
/*
|
|
* This little trick makes sure that
|
|
* sizeof(flex_array) == PAGE_SIZE
|
|
*/
|
|
char padding[FLEX_ARRAY_BASE_SIZE];
|
|
};
|
|
};
|
|
|
|
#define FLEX_ARRAY_INIT(size, total) { { {\
|
|
.element_size = (size), \
|
|
.total_nr_elements = (total), \
|
|
} } }
|
|
|
|
struct flex_array *flex_array_alloc(int element_size, unsigned int total,
|
|
gfp_t flags);
|
|
int flex_array_prealloc(struct flex_array *fa, unsigned int start,
|
|
unsigned int end, gfp_t flags);
|
|
void flex_array_free(struct flex_array *fa);
|
|
void flex_array_free_parts(struct flex_array *fa);
|
|
int flex_array_put(struct flex_array *fa, unsigned int element_nr, void *src,
|
|
gfp_t flags);
|
|
void *flex_array_get(struct flex_array *fa, unsigned int element_nr);
|
|
|
|
#endif /* _FLEX_ARRAY_H */
|