mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 21:57:43 +00:00
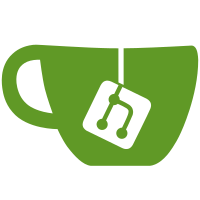
Teach objtool a little more about IRET so that we can avoid using the SAVE/RESTORE annotation. In particular, make the weird corner case in insn->restore go away. The purpose of that corner case is to deal with the fact that UNWIND_HINT_RESTORE lands on the instruction after IRET, but that instruction can end up being outside the basic block, consider: if (cond) sync_core() foo(); Then the hint will land on foo(), and we'll encounter the restore hint without ever having seen the save hint. By teaching objtool about the arch specific exception frame size, and assuming that any IRET in an STT_FUNC symbol is an exception frame sized POP, we can remove the use of save/restore hints for this code. Signed-off-by: Peter Zijlstra (Intel) <peterz@infradead.org> Reviewed-by: Miroslav Benes <mbenes@suse.cz> Reviewed-by: Alexandre Chartre <alexandre.chartre@oracle.com> Acked-by: Josh Poimboeuf <jpoimboe@redhat.com> Link: https://lkml.kernel.org/r/20200416115118.631224674@infradead.org Signed-off-by: Ingo Molnar <mingo@kernel.org>
87 lines
1.5 KiB
C
87 lines
1.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* Copyright (C) 2015 Josh Poimboeuf <jpoimboe@redhat.com>
|
|
*/
|
|
|
|
#ifndef _ARCH_H
|
|
#define _ARCH_H
|
|
|
|
#include <stdbool.h>
|
|
#include <linux/list.h>
|
|
#include "elf.h"
|
|
#include "cfi.h"
|
|
|
|
enum insn_type {
|
|
INSN_JUMP_CONDITIONAL,
|
|
INSN_JUMP_UNCONDITIONAL,
|
|
INSN_JUMP_DYNAMIC,
|
|
INSN_JUMP_DYNAMIC_CONDITIONAL,
|
|
INSN_CALL,
|
|
INSN_CALL_DYNAMIC,
|
|
INSN_RETURN,
|
|
INSN_EXCEPTION_RETURN,
|
|
INSN_CONTEXT_SWITCH,
|
|
INSN_STACK,
|
|
INSN_BUG,
|
|
INSN_NOP,
|
|
INSN_STAC,
|
|
INSN_CLAC,
|
|
INSN_STD,
|
|
INSN_CLD,
|
|
INSN_OTHER,
|
|
};
|
|
|
|
enum op_dest_type {
|
|
OP_DEST_REG,
|
|
OP_DEST_REG_INDIRECT,
|
|
OP_DEST_MEM,
|
|
OP_DEST_PUSH,
|
|
OP_DEST_PUSHF,
|
|
OP_DEST_LEAVE,
|
|
};
|
|
|
|
struct op_dest {
|
|
enum op_dest_type type;
|
|
unsigned char reg;
|
|
int offset;
|
|
};
|
|
|
|
enum op_src_type {
|
|
OP_SRC_REG,
|
|
OP_SRC_REG_INDIRECT,
|
|
OP_SRC_CONST,
|
|
OP_SRC_POP,
|
|
OP_SRC_POPF,
|
|
OP_SRC_ADD,
|
|
OP_SRC_AND,
|
|
};
|
|
|
|
struct op_src {
|
|
enum op_src_type type;
|
|
unsigned char reg;
|
|
int offset;
|
|
};
|
|
|
|
struct stack_op {
|
|
struct op_dest dest;
|
|
struct op_src src;
|
|
struct list_head list;
|
|
};
|
|
|
|
struct instruction;
|
|
|
|
void arch_initial_func_cfi_state(struct cfi_state *state);
|
|
|
|
int arch_decode_instruction(struct elf *elf, struct section *sec,
|
|
unsigned long offset, unsigned int maxlen,
|
|
unsigned int *len, enum insn_type *type,
|
|
unsigned long *immediate,
|
|
struct list_head *ops_list);
|
|
|
|
bool arch_callee_saved_reg(unsigned char reg);
|
|
|
|
unsigned long arch_jump_destination(struct instruction *insn);
|
|
|
|
unsigned long arch_dest_rela_offset(int addend);
|
|
|
|
#endif /* _ARCH_H */
|