mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
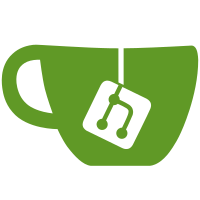
Functions in math-emu are annotated as ENTRY() symbols, but their ends are not annotated at all. But these are standard functions called from C, with proper stack register update etc. Omitting the ends means: * the annotations are not paired and we cannot deal with such functions e.g. in objtool * the symbols are not marked as functions in the object file * there are no sizes of the functions in the object file So fix this by adding ENDPROC() to each such case in math-emu. Signed-off-by: Jiri Slaby <jslaby@suse.cz> Cc: Andy Lutomirski <luto@kernel.org> Cc: Borislav Petkov <bp@alien8.de> Cc: Brian Gerst <brgerst@gmail.com> Cc: Denys Vlasenko <dvlasenk@redhat.com> Cc: H. Peter Anvin <hpa@zytor.com> Cc: Josh Poimboeuf <jpoimboe@redhat.com> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Thomas Gleixner <tglx@linutronix.de> Link: http://lkml.kernel.org/r/20170824080624.7768-1-jslaby@suse.cz Signed-off-by: Ingo Molnar <mingo@kernel.org>
149 lines
3.6 KiB
ArmAsm
149 lines
3.6 KiB
ArmAsm
/*---------------------------------------------------------------------------+
|
|
| reg_norm.S |
|
|
| |
|
|
| Copyright (C) 1992,1993,1994,1995,1997 |
|
|
| W. Metzenthen, 22 Parker St, Ormond, Vic 3163, |
|
|
| Australia. E-mail billm@suburbia.net |
|
|
| |
|
|
| Normalize the value in a FPU_REG. |
|
|
| |
|
|
| Call from C as: |
|
|
| int FPU_normalize(FPU_REG *n) |
|
|
| |
|
|
| int FPU_normalize_nuo(FPU_REG *n) |
|
|
| |
|
|
| Return value is the tag of the answer, or-ed with FPU_Exception if |
|
|
| one was raised, or -1 on internal error. |
|
|
| |
|
|
+---------------------------------------------------------------------------*/
|
|
|
|
#include "fpu_emu.h"
|
|
|
|
|
|
.text
|
|
ENTRY(FPU_normalize)
|
|
pushl %ebp
|
|
movl %esp,%ebp
|
|
pushl %ebx
|
|
|
|
movl PARAM1,%ebx
|
|
|
|
movl SIGH(%ebx),%edx
|
|
movl SIGL(%ebx),%eax
|
|
|
|
orl %edx,%edx /* ms bits */
|
|
js L_done /* Already normalized */
|
|
jnz L_shift_1 /* Shift left 1 - 31 bits */
|
|
|
|
orl %eax,%eax
|
|
jz L_zero /* The contents are zero */
|
|
|
|
movl %eax,%edx
|
|
xorl %eax,%eax
|
|
subw $32,EXP(%ebx) /* This can cause an underflow */
|
|
|
|
/* We need to shift left by 1 - 31 bits */
|
|
L_shift_1:
|
|
bsrl %edx,%ecx /* get the required shift in %ecx */
|
|
subl $31,%ecx
|
|
negl %ecx
|
|
shld %cl,%eax,%edx
|
|
shl %cl,%eax
|
|
subw %cx,EXP(%ebx) /* This can cause an underflow */
|
|
|
|
movl %edx,SIGH(%ebx)
|
|
movl %eax,SIGL(%ebx)
|
|
|
|
L_done:
|
|
cmpw EXP_OVER,EXP(%ebx)
|
|
jge L_overflow
|
|
|
|
cmpw EXP_UNDER,EXP(%ebx)
|
|
jle L_underflow
|
|
|
|
L_exit_valid:
|
|
movl TAG_Valid,%eax
|
|
|
|
/* Convert the exponent to 80x87 form. */
|
|
addw EXTENDED_Ebias,EXP(%ebx)
|
|
andw $0x7fff,EXP(%ebx)
|
|
|
|
L_exit:
|
|
popl %ebx
|
|
leave
|
|
ret
|
|
|
|
|
|
L_zero:
|
|
movw $0,EXP(%ebx)
|
|
movl TAG_Zero,%eax
|
|
jmp L_exit
|
|
|
|
L_underflow:
|
|
/* Convert the exponent to 80x87 form. */
|
|
addw EXTENDED_Ebias,EXP(%ebx)
|
|
push %ebx
|
|
call arith_underflow
|
|
pop %ebx
|
|
jmp L_exit
|
|
|
|
L_overflow:
|
|
/* Convert the exponent to 80x87 form. */
|
|
addw EXTENDED_Ebias,EXP(%ebx)
|
|
push %ebx
|
|
call arith_overflow
|
|
pop %ebx
|
|
jmp L_exit
|
|
ENDPROC(FPU_normalize)
|
|
|
|
|
|
|
|
/* Normalise without reporting underflow or overflow */
|
|
ENTRY(FPU_normalize_nuo)
|
|
pushl %ebp
|
|
movl %esp,%ebp
|
|
pushl %ebx
|
|
|
|
movl PARAM1,%ebx
|
|
|
|
movl SIGH(%ebx),%edx
|
|
movl SIGL(%ebx),%eax
|
|
|
|
orl %edx,%edx /* ms bits */
|
|
js L_exit_nuo_valid /* Already normalized */
|
|
jnz L_nuo_shift_1 /* Shift left 1 - 31 bits */
|
|
|
|
orl %eax,%eax
|
|
jz L_exit_nuo_zero /* The contents are zero */
|
|
|
|
movl %eax,%edx
|
|
xorl %eax,%eax
|
|
subw $32,EXP(%ebx) /* This can cause an underflow */
|
|
|
|
/* We need to shift left by 1 - 31 bits */
|
|
L_nuo_shift_1:
|
|
bsrl %edx,%ecx /* get the required shift in %ecx */
|
|
subl $31,%ecx
|
|
negl %ecx
|
|
shld %cl,%eax,%edx
|
|
shl %cl,%eax
|
|
subw %cx,EXP(%ebx) /* This can cause an underflow */
|
|
|
|
movl %edx,SIGH(%ebx)
|
|
movl %eax,SIGL(%ebx)
|
|
|
|
L_exit_nuo_valid:
|
|
movl TAG_Valid,%eax
|
|
|
|
popl %ebx
|
|
leave
|
|
ret
|
|
|
|
L_exit_nuo_zero:
|
|
movl TAG_Zero,%eax
|
|
movw EXP_UNDER,EXP(%ebx)
|
|
|
|
popl %ebx
|
|
leave
|
|
ret
|
|
ENDPROC(FPU_normalize_nuo)
|