mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 02:39:48 +00:00
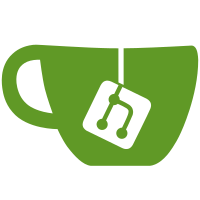
We currently initialize the arch timer IRQ numbers from the reset code, presumably because we once intended to model multiple CPU or SoC types from within the kernel and have hard-coded reset values in the reset code. As we are moving towards userspace being in charge of more fine-grained CPU emulation and stitching together the pieces needed to emulate a particular type of CPU, we should no longer have a tight coupling between resetting a VCPU and setting IRQ numbers. Therefore, move the logic to define and use the default IRQ numbers to the timer code and set the IRQ number immediately when creating the VCPU. Signed-off-by: Christoffer Dall <cdall@linaro.org> Reviewed-by: Marc Zyngier <marc.zyngier@arm.com>
74 lines
2.2 KiB
C
74 lines
2.2 KiB
C
/*
|
|
* Copyright (C) 2012 - Virtual Open Systems and Columbia University
|
|
* Author: Christoffer Dall <c.dall@virtualopensystems.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License, version 2, as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
*/
|
|
#include <linux/compiler.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/kvm_host.h>
|
|
#include <linux/kvm.h>
|
|
|
|
#include <asm/unified.h>
|
|
#include <asm/ptrace.h>
|
|
#include <asm/cputype.h>
|
|
#include <asm/kvm_arm.h>
|
|
#include <asm/kvm_coproc.h>
|
|
|
|
#include <kvm/arm_arch_timer.h>
|
|
|
|
/******************************************************************************
|
|
* Cortex-A15 and Cortex-A7 Reset Values
|
|
*/
|
|
|
|
static struct kvm_regs cortexa_regs_reset = {
|
|
.usr_regs.ARM_cpsr = SVC_MODE | PSR_A_BIT | PSR_I_BIT | PSR_F_BIT,
|
|
};
|
|
|
|
|
|
/*******************************************************************************
|
|
* Exported reset function
|
|
*/
|
|
|
|
/**
|
|
* kvm_reset_vcpu - sets core registers and cp15 registers to reset value
|
|
* @vcpu: The VCPU pointer
|
|
*
|
|
* This function finds the right table above and sets the registers on the
|
|
* virtual CPU struct to their architecturally defined reset values.
|
|
*/
|
|
int kvm_reset_vcpu(struct kvm_vcpu *vcpu)
|
|
{
|
|
struct kvm_regs *reset_regs;
|
|
|
|
switch (vcpu->arch.target) {
|
|
case KVM_ARM_TARGET_CORTEX_A7:
|
|
case KVM_ARM_TARGET_CORTEX_A15:
|
|
reset_regs = &cortexa_regs_reset;
|
|
vcpu->arch.midr = read_cpuid_id();
|
|
break;
|
|
default:
|
|
return -ENODEV;
|
|
}
|
|
|
|
/* Reset core registers */
|
|
memcpy(&vcpu->arch.ctxt.gp_regs, reset_regs, sizeof(vcpu->arch.ctxt.gp_regs));
|
|
|
|
/* Reset CP15 registers */
|
|
kvm_reset_coprocs(vcpu);
|
|
|
|
/* Reset arch_timer context */
|
|
return kvm_timer_vcpu_reset(vcpu);
|
|
}
|