mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
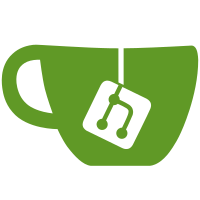
SMT now could be disabled via "/sys/devices/system/cpu/smt/control". Status is shown in "/sys/devices/system/cpu/smt/active" simply as "0" / "1". If this knob isn't here then fallback to checking topology as before. Signed-off-by: Konstantin Khlebnikov <khlebnikov@yandex-team.ru> Cc: Andi Kleen <ak@linux.intel.com> Cc: Dmitry Monakhov <dmtrmonakhov@yandex-team.ru> Cc: Jiri Olsa <jolsa@kernel.org> Cc: Kan Liang <kan.liang@linux.intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Link: http://lore.kernel.org/lkml/158817741394.748034.9273604089138009552.stgit@buzz Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
52 lines
1.1 KiB
C
52 lines
1.1 KiB
C
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <unistd.h>
|
|
#include <linux/bitops.h>
|
|
#include "api/fs/fs.h"
|
|
#include "smt.h"
|
|
|
|
int smt_on(void)
|
|
{
|
|
static bool cached;
|
|
static int cached_result;
|
|
int cpu;
|
|
int ncpu;
|
|
|
|
if (cached)
|
|
return cached_result;
|
|
|
|
if (sysfs__read_int("devices/system/cpu/smt/active", &cached_result) > 0)
|
|
goto done;
|
|
|
|
ncpu = sysconf(_SC_NPROCESSORS_CONF);
|
|
for (cpu = 0; cpu < ncpu; cpu++) {
|
|
unsigned long long siblings;
|
|
char *str;
|
|
size_t strlen;
|
|
char fn[256];
|
|
|
|
snprintf(fn, sizeof fn,
|
|
"devices/system/cpu/cpu%d/topology/core_cpus", cpu);
|
|
if (sysfs__read_str(fn, &str, &strlen) < 0) {
|
|
snprintf(fn, sizeof fn,
|
|
"devices/system/cpu/cpu%d/topology/thread_siblings",
|
|
cpu);
|
|
if (sysfs__read_str(fn, &str, &strlen) < 0)
|
|
continue;
|
|
}
|
|
/* Entry is hex, but does not have 0x, so need custom parser */
|
|
siblings = strtoull(str, NULL, 16);
|
|
free(str);
|
|
if (hweight64(siblings) > 1) {
|
|
cached_result = 1;
|
|
cached = true;
|
|
break;
|
|
}
|
|
}
|
|
if (!cached) {
|
|
cached_result = 0;
|
|
done:
|
|
cached = true;
|
|
}
|
|
return cached_result;
|
|
}
|