mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-27 19:29:37 +00:00
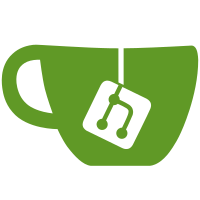
When rngd is run as root then lots of these types of message will appear
in the kernel log if the TPM has been configured to provide random bytes:
[ 7406.275163] tpm tpm0: tpm_transmit: tpm_recv: error -4
The issue is caused by the following call that is interrupted while
waiting for the TPM's response.
sig = wait_event_interruptible(ibmvtpm->wq, !ibmvtpm->tpm_processing_cmd);
Rather than waiting for the response in the low level driver, have it use
the polling loop in tpm_try_transmit() that uses a command's duration to
poll until a result has been returned by the TPM, thus ending when the
timeout has occurred but not responding to signals and ctrl-c anymore. To
stay in this polling loop extend tpm_ibmvtpm_status() to return
'true' for as long as the vTPM is indicated as being busy in
tpm_processing_cmd. Since the loop requires the TPM's timeouts, get them
now using tpm_get_timeouts() after setting the TPM2 version flag on the
chip.
To recreat the resolved issue start rngd like this:
sudo rngd -r /dev/hwrng -t
sudo rngd -r /dev/tpm0 -t
Link: https://bugzilla.redhat.com/show_bug.cgi?id=1981473
Fixes: 6674ff145e
("tpm_ibmvtpm: properly handle interrupted packet receptions")
Cc: Nayna Jain <nayna@linux.ibm.com>
Cc: George Wilson <gcwilson@linux.ibm.com>
Reported-by: Nageswara R Sastry <rnsastry@linux.ibm.com>
Signed-off-by: Stefan Berger <stefanb@linux.ibm.com>
Tested-by: Nageswara R Sastry <rnsastry@linux.ibm.com>
Reviewed-by: Jarkko Sakkinen <jarkko@kernel.org>
Signed-off-by: Jarkko Sakkinen <jarkko@kernel.org>
73 lines
1.8 KiB
C
73 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (C) 2012 IBM Corporation
|
|
*
|
|
* Author: Ashley Lai <ashleydlai@gmail.com>
|
|
*
|
|
* Maintained by: <tpmdd-devel@lists.sourceforge.net>
|
|
*
|
|
* Device driver for TCG/TCPA TPM (trusted platform module).
|
|
* Specifications at www.trustedcomputinggroup.org
|
|
*/
|
|
|
|
#ifndef __TPM_IBMVTPM_H__
|
|
#define __TPM_IBMVTPM_H__
|
|
|
|
/* vTPM Message Format 1 */
|
|
struct ibmvtpm_crq {
|
|
u8 valid;
|
|
u8 msg;
|
|
__be16 len;
|
|
__be32 data;
|
|
__be64 reserved;
|
|
} __attribute__((packed, aligned(8)));
|
|
|
|
struct ibmvtpm_crq_queue {
|
|
struct ibmvtpm_crq *crq_addr;
|
|
u32 index;
|
|
u32 num_entry;
|
|
wait_queue_head_t wq;
|
|
};
|
|
|
|
struct ibmvtpm_dev {
|
|
struct device *dev;
|
|
struct vio_dev *vdev;
|
|
struct ibmvtpm_crq_queue crq_queue;
|
|
dma_addr_t crq_dma_handle;
|
|
u32 rtce_size;
|
|
void __iomem *rtce_buf;
|
|
dma_addr_t rtce_dma_handle;
|
|
spinlock_t rtce_lock;
|
|
wait_queue_head_t wq;
|
|
u16 res_len;
|
|
u32 vtpm_version;
|
|
u8 tpm_processing_cmd;
|
|
};
|
|
|
|
#define CRQ_RES_BUF_SIZE PAGE_SIZE
|
|
|
|
/* Initialize CRQ */
|
|
#define INIT_CRQ_CMD 0xC001000000000000LL /* Init cmd */
|
|
#define INIT_CRQ_COMP_CMD 0xC002000000000000LL /* Init complete cmd */
|
|
#define INIT_CRQ_RES 0x01 /* Init respond */
|
|
#define INIT_CRQ_COMP_RES 0x02 /* Init complete respond */
|
|
#define VALID_INIT_CRQ 0xC0 /* Valid command for init crq */
|
|
|
|
/* vTPM CRQ response is the message type | 0x80 */
|
|
#define VTPM_MSG_RES 0x80
|
|
#define IBMVTPM_VALID_CMD 0x80
|
|
|
|
/* vTPM CRQ message types */
|
|
#define VTPM_GET_VERSION 0x01
|
|
#define VTPM_GET_VERSION_RES (0x01 | VTPM_MSG_RES)
|
|
|
|
#define VTPM_TPM_COMMAND 0x02
|
|
#define VTPM_TPM_COMMAND_RES (0x02 | VTPM_MSG_RES)
|
|
|
|
#define VTPM_GET_RTCE_BUFFER_SIZE 0x03
|
|
#define VTPM_GET_RTCE_BUFFER_SIZE_RES (0x03 | VTPM_MSG_RES)
|
|
|
|
#define VTPM_PREPARE_TO_SUSPEND 0x04
|
|
#define VTPM_PREPARE_TO_SUSPEND_RES (0x04 | VTPM_MSG_RES)
|
|
|
|
#endif
|