mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-27 19:29:37 +00:00
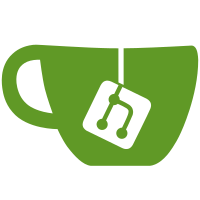
The size of the buffers for storing context's and sessions can vary from
arch to arch as PAGE_SIZE can be anything between 4 kB and 256 kB (the
maximum for PPC64). Define a fixed buffer size set to 16 kB. This should be
enough for most use with three handles (that is how many we allow at the
moment). Parametrize the buffer size while doing this, so that it is easier
to revisit this later on if required.
Cc: stable@vger.kernel.org
Reported-by: Stefan Berger <stefanb@linux.ibm.com>
Fixes: 745b361e98
("tpm: infrastructure for TPM spaces")
Reviewed-by: Jerry Snitselaar <jsnitsel@redhat.com>
Tested-by: Stefan Berger <stefanb@linux.ibm.com>
Signed-off-by: Jarkko Sakkinen <jarkko.sakkinen@linux.intel.com>
55 lines
1.2 KiB
C
55 lines
1.2 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Copyright (C) 2017 James.Bottomley@HansenPartnership.com
|
|
*/
|
|
#include <linux/slab.h>
|
|
#include "tpm-dev.h"
|
|
|
|
struct tpmrm_priv {
|
|
struct file_priv priv;
|
|
struct tpm_space space;
|
|
};
|
|
|
|
static int tpmrm_open(struct inode *inode, struct file *file)
|
|
{
|
|
struct tpm_chip *chip;
|
|
struct tpmrm_priv *priv;
|
|
int rc;
|
|
|
|
chip = container_of(inode->i_cdev, struct tpm_chip, cdevs);
|
|
priv = kzalloc(sizeof(*priv), GFP_KERNEL);
|
|
if (priv == NULL)
|
|
return -ENOMEM;
|
|
|
|
rc = tpm2_init_space(&priv->space, TPM2_SPACE_BUFFER_SIZE);
|
|
if (rc) {
|
|
kfree(priv);
|
|
return -ENOMEM;
|
|
}
|
|
|
|
tpm_common_open(file, chip, &priv->priv, &priv->space);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int tpmrm_release(struct inode *inode, struct file *file)
|
|
{
|
|
struct file_priv *fpriv = file->private_data;
|
|
struct tpmrm_priv *priv = container_of(fpriv, struct tpmrm_priv, priv);
|
|
|
|
tpm_common_release(file, fpriv);
|
|
tpm2_del_space(fpriv->chip, &priv->space);
|
|
kfree(priv);
|
|
|
|
return 0;
|
|
}
|
|
|
|
const struct file_operations tpmrm_fops = {
|
|
.owner = THIS_MODULE,
|
|
.llseek = no_llseek,
|
|
.open = tpmrm_open,
|
|
.read = tpm_common_read,
|
|
.write = tpm_common_write,
|
|
.poll = tpm_common_poll,
|
|
.release = tpmrm_release,
|
|
};
|