mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-04 09:59:32 +00:00
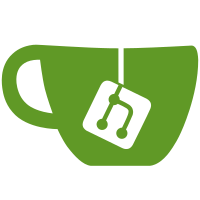
This patch includes some fixes and cleanup for idle-page writeback. 1. writeback_limit interface Now writeback_limit interface is rather conusing. For example, once writeback limit budget is exausted, admin can see 0 from /sys/block/zramX/writeback_limit which is same semantic with disable writeback_limit at this moment. IOW, admin cannot tell that zero came from disable writeback limit or exausted writeback limit. To make the interface clear, let's sepatate enable of writeback limit to another knob - /sys/block/zram0/writeback_limit_enable * before: while true : # to re-enable writeback limit once previous one is used up echo 0 > /sys/block/zram0/writeback_limit echo $((200<<20)) > /sys/block/zram0/writeback_limit .. .. # used up the writeback limit budget * new # To enable writeback limit, from the beginning, admin should # enable it. echo $((200<<20)) > /sys/block/zram0/writeback_limit echo 1 > /sys/block/zram/0/writeback_limit_enable while true : echo $((200<<20)) > /sys/block/zram0/writeback_limit .. .. # used up the writeback limit budget It's much strightforward. 2. fix condition check idle/huge writeback mode check The mode in writeback_store is not bit opeartion any more so no need to use bit operations. Furthermore, current condition check is broken in that it does writeback every pages regardless of huge/idle. 3. clean up idle_store No need to use goto. [minchan@kernel.org: missed spin_lock_init] Link: http://lkml.kernel.org/r/20190103001601.GA255139@google.com Link: http://lkml.kernel.org/r/20181224033529.19450-1-minchan@kernel.org Signed-off-by: Minchan Kim <minchan@kernel.org> Suggested-by: John Dias <joaodias@google.com> Cc: Sergey Senozhatsky <sergey.senozhatsky.work@gmail.com> Cc: John Dias <joaodias@google.com> Cc: Srinivas Paladugu <srnvs@google.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
129 lines
3.7 KiB
C
129 lines
3.7 KiB
C
/*
|
|
* Compressed RAM block device
|
|
*
|
|
* Copyright (C) 2008, 2009, 2010 Nitin Gupta
|
|
* 2012, 2013 Minchan Kim
|
|
*
|
|
* This code is released using a dual license strategy: BSD/GPL
|
|
* You can choose the licence that better fits your requirements.
|
|
*
|
|
* Released under the terms of 3-clause BSD License
|
|
* Released under the terms of GNU General Public License Version 2.0
|
|
*
|
|
*/
|
|
|
|
#ifndef _ZRAM_DRV_H_
|
|
#define _ZRAM_DRV_H_
|
|
|
|
#include <linux/rwsem.h>
|
|
#include <linux/zsmalloc.h>
|
|
#include <linux/crypto.h>
|
|
|
|
#include "zcomp.h"
|
|
|
|
#define SECTORS_PER_PAGE_SHIFT (PAGE_SHIFT - SECTOR_SHIFT)
|
|
#define SECTORS_PER_PAGE (1 << SECTORS_PER_PAGE_SHIFT)
|
|
#define ZRAM_LOGICAL_BLOCK_SHIFT 12
|
|
#define ZRAM_LOGICAL_BLOCK_SIZE (1 << ZRAM_LOGICAL_BLOCK_SHIFT)
|
|
#define ZRAM_SECTOR_PER_LOGICAL_BLOCK \
|
|
(1 << (ZRAM_LOGICAL_BLOCK_SHIFT - SECTOR_SHIFT))
|
|
|
|
|
|
/*
|
|
* The lower ZRAM_FLAG_SHIFT bits of table.flags is for
|
|
* object size (excluding header), the higher bits is for
|
|
* zram_pageflags.
|
|
*
|
|
* zram is mainly used for memory efficiency so we want to keep memory
|
|
* footprint small so we can squeeze size and flags into a field.
|
|
* The lower ZRAM_FLAG_SHIFT bits is for object size (excluding header),
|
|
* the higher bits is for zram_pageflags.
|
|
*/
|
|
#define ZRAM_FLAG_SHIFT 24
|
|
|
|
/* Flags for zram pages (table[page_no].flags) */
|
|
enum zram_pageflags {
|
|
/* zram slot is locked */
|
|
ZRAM_LOCK = ZRAM_FLAG_SHIFT,
|
|
ZRAM_SAME, /* Page consists the same element */
|
|
ZRAM_WB, /* page is stored on backing_device */
|
|
ZRAM_UNDER_WB, /* page is under writeback */
|
|
ZRAM_HUGE, /* Incompressible page */
|
|
ZRAM_IDLE, /* not accessed page since last idle marking */
|
|
|
|
__NR_ZRAM_PAGEFLAGS,
|
|
};
|
|
|
|
/*-- Data structures */
|
|
|
|
/* Allocated for each disk page */
|
|
struct zram_table_entry {
|
|
union {
|
|
unsigned long handle;
|
|
unsigned long element;
|
|
};
|
|
unsigned long flags;
|
|
#ifdef CONFIG_ZRAM_MEMORY_TRACKING
|
|
ktime_t ac_time;
|
|
#endif
|
|
};
|
|
|
|
struct zram_stats {
|
|
atomic64_t compr_data_size; /* compressed size of pages stored */
|
|
atomic64_t num_reads; /* failed + successful */
|
|
atomic64_t num_writes; /* --do-- */
|
|
atomic64_t failed_reads; /* can happen when memory is too low */
|
|
atomic64_t failed_writes; /* can happen when memory is too low */
|
|
atomic64_t invalid_io; /* non-page-aligned I/O requests */
|
|
atomic64_t notify_free; /* no. of swap slot free notifications */
|
|
atomic64_t same_pages; /* no. of same element filled pages */
|
|
atomic64_t huge_pages; /* no. of huge pages */
|
|
atomic64_t pages_stored; /* no. of pages currently stored */
|
|
atomic_long_t max_used_pages; /* no. of maximum pages stored */
|
|
atomic64_t writestall; /* no. of write slow paths */
|
|
atomic64_t miss_free; /* no. of missed free */
|
|
#ifdef CONFIG_ZRAM_WRITEBACK
|
|
atomic64_t bd_count; /* no. of pages in backing device */
|
|
atomic64_t bd_reads; /* no. of reads from backing device */
|
|
atomic64_t bd_writes; /* no. of writes from backing device */
|
|
#endif
|
|
};
|
|
|
|
struct zram {
|
|
struct zram_table_entry *table;
|
|
struct zs_pool *mem_pool;
|
|
struct zcomp *comp;
|
|
struct gendisk *disk;
|
|
/* Prevent concurrent execution of device init */
|
|
struct rw_semaphore init_lock;
|
|
/*
|
|
* the number of pages zram can consume for storing compressed data
|
|
*/
|
|
unsigned long limit_pages;
|
|
|
|
struct zram_stats stats;
|
|
/*
|
|
* This is the limit on amount of *uncompressed* worth of data
|
|
* we can store in a disk.
|
|
*/
|
|
u64 disksize; /* bytes */
|
|
char compressor[CRYPTO_MAX_ALG_NAME];
|
|
/*
|
|
* zram is claimed so open request will be failed
|
|
*/
|
|
bool claim; /* Protected by bdev->bd_mutex */
|
|
struct file *backing_dev;
|
|
#ifdef CONFIG_ZRAM_WRITEBACK
|
|
spinlock_t wb_limit_lock;
|
|
bool wb_limit_enable;
|
|
u64 bd_wb_limit;
|
|
struct block_device *bdev;
|
|
unsigned int old_block_size;
|
|
unsigned long *bitmap;
|
|
unsigned long nr_pages;
|
|
#endif
|
|
#ifdef CONFIG_ZRAM_MEMORY_TRACKING
|
|
struct dentry *debugfs_dir;
|
|
#endif
|
|
};
|
|
#endif
|