mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 16:29:59 +00:00
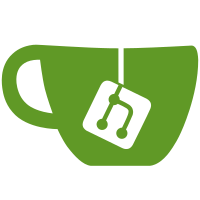
Some systems allow devices to handle I/O Page Faults in the core mm. For
example systems implementing the PCIe PRI extension or Arm SMMU stall
model. Infrastructure for reporting these recoverable page faults was
added to the IOMMU core by commit 0c830e6b32
("iommu: Introduce device
fault report API"). Add a page fault handler for host SVA.
IOMMU driver can now instantiate several fault workqueues and link them
to IOPF-capable devices. Drivers can choose between a single global
workqueue, one per IOMMU device, one per low-level fault queue, one per
domain, etc.
When it receives a fault event, most commonly in an IRQ handler, the
IOMMU driver reports the fault using iommu_report_device_fault(), which
calls the registered handler. The page fault handler then calls the mm
fault handler, and reports either success or failure with
iommu_page_response(). After the handler succeeds, the hardware retries
the access.
The iopf_param pointer could be embedded into iommu_fault_param. But
putting iopf_param into the iommu_param structure allows us not to care
about ordering between calls to iopf_queue_add_device() and
iommu_register_device_fault_handler().
Tested-by: Lu Baolu <baolu.lu@linux.intel.com>
Reviewed-by: Eric Auger <eric.auger@redhat.com>
Reviewed-by: Jacob Pan <jacob.jun.pan@linux.intel.com>
Reviewed-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
Reviewed-by: Lu Baolu <baolu.lu@linux.intel.com>
Signed-off-by: Jean-Philippe Brucker <jean-philippe@linaro.org>
Link: https://lore.kernel.org/r/20210401154718.307519-7-jean-philippe@linaro.org
Signed-off-by: Joerg Roedel <jroedel@suse.de>
68 lines
1.6 KiB
C
68 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* SVA library for IOMMU drivers
|
|
*/
|
|
#ifndef _IOMMU_SVA_LIB_H
|
|
#define _IOMMU_SVA_LIB_H
|
|
|
|
#include <linux/ioasid.h>
|
|
#include <linux/mm_types.h>
|
|
|
|
int iommu_sva_alloc_pasid(struct mm_struct *mm, ioasid_t min, ioasid_t max);
|
|
void iommu_sva_free_pasid(struct mm_struct *mm);
|
|
struct mm_struct *iommu_sva_find(ioasid_t pasid);
|
|
|
|
/* I/O Page fault */
|
|
struct device;
|
|
struct iommu_fault;
|
|
struct iopf_queue;
|
|
|
|
#ifdef CONFIG_IOMMU_SVA_LIB
|
|
int iommu_queue_iopf(struct iommu_fault *fault, void *cookie);
|
|
|
|
int iopf_queue_add_device(struct iopf_queue *queue, struct device *dev);
|
|
int iopf_queue_remove_device(struct iopf_queue *queue,
|
|
struct device *dev);
|
|
int iopf_queue_flush_dev(struct device *dev);
|
|
struct iopf_queue *iopf_queue_alloc(const char *name);
|
|
void iopf_queue_free(struct iopf_queue *queue);
|
|
int iopf_queue_discard_partial(struct iopf_queue *queue);
|
|
|
|
#else /* CONFIG_IOMMU_SVA_LIB */
|
|
static inline int iommu_queue_iopf(struct iommu_fault *fault, void *cookie)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int iopf_queue_add_device(struct iopf_queue *queue,
|
|
struct device *dev)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int iopf_queue_remove_device(struct iopf_queue *queue,
|
|
struct device *dev)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int iopf_queue_flush_dev(struct device *dev)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline struct iopf_queue *iopf_queue_alloc(const char *name)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static inline void iopf_queue_free(struct iopf_queue *queue)
|
|
{
|
|
}
|
|
|
|
static inline int iopf_queue_discard_partial(struct iopf_queue *queue)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
#endif /* CONFIG_IOMMU_SVA_LIB */
|
|
#endif /* _IOMMU_SVA_LIB_H */
|