mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 16:29:59 +00:00
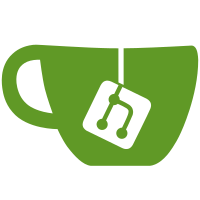
Bigger work around ARM Primecell PL35x SMC memory controller driver by Miquel Raynal built on previous series from Naga Sureshkumar Relli. This includes bindings cleanup and correction, converting these to dtschema and several cleanyps in pl353-smc driver. -----BEGIN PGP SIGNATURE----- iQJEBAABCgAuFiEE3dJiKD0RGyM7briowTdm5oaLg9cFAmDDbN8QHGtyemtAa2Vy bmVsLm9yZwAKCRDBN2bmhouD1y6sD/0asCOer/fNfM4qDyr4TtDsBFO8ckKoH7h+ /VEDZGyIw5pc3RYyxp62i4qGzjAh2Q65SaGZ+AwCt1Hhcb3e5PutmYgqvNd6hnJR eWtUi8/kyzmvQhH+QG1umiOFAngv8hs1dmEfNHb51IYJMXp2f7l4byoCmZilqMaj em94DsLAE+AZaz00zucJpX8HG4UsqOvL+eZWn81AQKlMLM0OyiALCZwxNoXgYaaJ 6UZh7t3YMkQeK7V3Z+B98QP4hfAfCDhaO1K8femu1zfVq517ceab9oICXcKQ/TvO ntrKSBLXywWVwlBZIXqLM4k5XhZyVBUXEEUl+F8FQkEkxiewYA2WRFN7oOyvE3uZ l7NejRPGxUaQZ46Irtl6yhpqhgZAQ/dA+gk5RdaT6LdT8MF1GDkwS6Rh7rDOx6jg GBZ1l1A3b4/BX90LVTRIUnOnUkHLNRS4Fz4GQfpl0sBdSO+9ELyruJezhcZCza9M sIlyqNoKFDTvhby5xwDLJf78Dn6Ocft58BKMPl2IAaBvGem6pRdWmc8P6qSE7edv CixfGmSloPhZtFtN9gI6ME1SxKPCu/z8Yji5nRtNFbbXkVId8PDawO9mawf/GYeU KehtekzlDsr21by4bgXSqq6TLiXVgEzHy4aQp2t8fWJct/K9seFLLiPE8Ajrl6ce h9Zj3T3Frw== =iNR6 -----END PGP SIGNATURE----- Merge tag 'memory-controller-drv-pl353-5.14' of https://git.kernel.org/pub/scm/linux/kernel/git/krzk/linux-mem-ctrl into arm/drivers Memory controller drivers for v5.14 - PL353 Bigger work around ARM Primecell PL35x SMC memory controller driver by Miquel Raynal built on previous series from Naga Sureshkumar Relli. This includes bindings cleanup and correction, converting these to dtschema and several cleanyps in pl353-smc driver. * tag 'memory-controller-drv-pl353-5.14' of https://git.kernel.org/pub/scm/linux/kernel/git/krzk/linux-mem-ctrl: dt-binding: memory: pl353-smc: Convert to yaml MAINTAINERS: Add PL353 SMC entry memory: pl353-smc: Declare variables following a reverse christmas tree order memory: pl353-smc: Avoid useless acronyms in descriptions memory: pl353-smc: Let lower level controller drivers handle inits memory: pl353-smc: Rename goto labels memory: pl353-smc: Fix style dt-binding: memory: pl353-smc: Fix the NAND controller node in the example dt-binding: memory: pl353-smc: Drop unsupported nodes from the example dt-binding: memory: pl353-smc: Fix the example syntax and style dt-binding: memory: pl353-smc: Describe the child reg property dt-binding: memory: pl353-smc: Drop the partitioning section dt-binding: memory: pl353-smc: Document the range property dt-binding: memory: pl353-smc: Rephrase the binding Link: https://lore.kernel.org/r/20210611140659.61980-2-krzysztof.kozlowski@canonical.com Signed-off-by: Olof Johansson <olof@lixom.net>
168 lines
3.7 KiB
C
168 lines
3.7 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* ARM PL353 SMC driver
|
|
*
|
|
* Copyright (C) 2012 - 2018 Xilinx, Inc
|
|
* Author: Punnaiah Choudary Kalluri <punnaiah@xilinx.com>
|
|
* Author: Naga Sureshkumar Relli <nagasure@xilinx.com>
|
|
*/
|
|
|
|
#include <linux/clk.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of_platform.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/amba/bus.h>
|
|
|
|
/**
|
|
* struct pl353_smc_data - Private smc driver structure
|
|
* @memclk: Pointer to the peripheral clock
|
|
* @aclk: Pointer to the AXI peripheral clock
|
|
*/
|
|
struct pl353_smc_data {
|
|
struct clk *memclk;
|
|
struct clk *aclk;
|
|
};
|
|
|
|
static int __maybe_unused pl353_smc_suspend(struct device *dev)
|
|
{
|
|
struct pl353_smc_data *pl353_smc = dev_get_drvdata(dev);
|
|
|
|
clk_disable(pl353_smc->memclk);
|
|
clk_disable(pl353_smc->aclk);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int __maybe_unused pl353_smc_resume(struct device *dev)
|
|
{
|
|
struct pl353_smc_data *pl353_smc = dev_get_drvdata(dev);
|
|
int ret;
|
|
|
|
ret = clk_enable(pl353_smc->aclk);
|
|
if (ret) {
|
|
dev_err(dev, "Cannot enable axi domain clock.\n");
|
|
return ret;
|
|
}
|
|
|
|
ret = clk_enable(pl353_smc->memclk);
|
|
if (ret) {
|
|
dev_err(dev, "Cannot enable memory clock.\n");
|
|
clk_disable(pl353_smc->aclk);
|
|
return ret;
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
static SIMPLE_DEV_PM_OPS(pl353_smc_dev_pm_ops, pl353_smc_suspend,
|
|
pl353_smc_resume);
|
|
|
|
static const struct of_device_id pl353_smc_supported_children[] = {
|
|
{
|
|
.compatible = "cfi-flash"
|
|
},
|
|
{
|
|
.compatible = "arm,pl353-nand-r2p1",
|
|
},
|
|
{}
|
|
};
|
|
|
|
static int pl353_smc_probe(struct amba_device *adev, const struct amba_id *id)
|
|
{
|
|
struct device_node *of_node = adev->dev.of_node;
|
|
const struct of_device_id *match = NULL;
|
|
struct pl353_smc_data *pl353_smc;
|
|
struct device_node *child;
|
|
int err;
|
|
|
|
pl353_smc = devm_kzalloc(&adev->dev, sizeof(*pl353_smc), GFP_KERNEL);
|
|
if (!pl353_smc)
|
|
return -ENOMEM;
|
|
|
|
pl353_smc->aclk = devm_clk_get(&adev->dev, "apb_pclk");
|
|
if (IS_ERR(pl353_smc->aclk)) {
|
|
dev_err(&adev->dev, "aclk clock not found.\n");
|
|
return PTR_ERR(pl353_smc->aclk);
|
|
}
|
|
|
|
pl353_smc->memclk = devm_clk_get(&adev->dev, "memclk");
|
|
if (IS_ERR(pl353_smc->memclk)) {
|
|
dev_err(&adev->dev, "memclk clock not found.\n");
|
|
return PTR_ERR(pl353_smc->memclk);
|
|
}
|
|
|
|
err = clk_prepare_enable(pl353_smc->aclk);
|
|
if (err) {
|
|
dev_err(&adev->dev, "Unable to enable AXI clock.\n");
|
|
return err;
|
|
}
|
|
|
|
err = clk_prepare_enable(pl353_smc->memclk);
|
|
if (err) {
|
|
dev_err(&adev->dev, "Unable to enable memory clock.\n");
|
|
goto disable_axi_clk;
|
|
}
|
|
|
|
amba_set_drvdata(adev, pl353_smc);
|
|
|
|
/* Find compatible children. Only a single child is supported */
|
|
for_each_available_child_of_node(of_node, child) {
|
|
match = of_match_node(pl353_smc_supported_children, child);
|
|
if (!match) {
|
|
dev_warn(&adev->dev, "unsupported child node\n");
|
|
continue;
|
|
}
|
|
break;
|
|
}
|
|
if (!match) {
|
|
err = -ENODEV;
|
|
dev_err(&adev->dev, "no matching children\n");
|
|
goto disable_mem_clk;
|
|
}
|
|
|
|
of_platform_device_create(child, NULL, &adev->dev);
|
|
|
|
return 0;
|
|
|
|
disable_mem_clk:
|
|
clk_disable_unprepare(pl353_smc->memclk);
|
|
disable_axi_clk:
|
|
clk_disable_unprepare(pl353_smc->aclk);
|
|
|
|
return err;
|
|
}
|
|
|
|
static void pl353_smc_remove(struct amba_device *adev)
|
|
{
|
|
struct pl353_smc_data *pl353_smc = amba_get_drvdata(adev);
|
|
|
|
clk_disable_unprepare(pl353_smc->memclk);
|
|
clk_disable_unprepare(pl353_smc->aclk);
|
|
}
|
|
|
|
static const struct amba_id pl353_ids[] = {
|
|
{
|
|
.id = 0x00041353,
|
|
.mask = 0x000fffff,
|
|
},
|
|
{ 0, 0 },
|
|
};
|
|
MODULE_DEVICE_TABLE(amba, pl353_ids);
|
|
|
|
static struct amba_driver pl353_smc_driver = {
|
|
.drv = {
|
|
.owner = THIS_MODULE,
|
|
.name = "pl353-smc",
|
|
.pm = &pl353_smc_dev_pm_ops,
|
|
},
|
|
.id_table = pl353_ids,
|
|
.probe = pl353_smc_probe,
|
|
.remove = pl353_smc_remove,
|
|
};
|
|
|
|
module_amba_driver(pl353_smc_driver);
|
|
|
|
MODULE_AUTHOR("Xilinx, Inc.");
|
|
MODULE_DESCRIPTION("ARM PL353 SMC Driver");
|
|
MODULE_LICENSE("GPL");
|