mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-13 14:14:37 +00:00
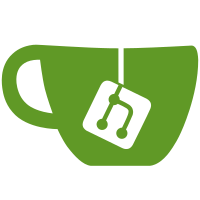
Some people run general-purpose distribution kernels on netbooks with a card that is physically non-removable or logically non-removable (e.g. used for /home) and cannot be cleanly unmounted during suspend. Add a module parameter to set whether cards are assumed removable or non-removable, with the default set by CONFIG_MMC_UNSAFE_RESUME. In general, it is not possible to tell whether a card present in an MMC slot after resume is the same that was there before suspend. So there are two possible behaviours, each of which will cause data loss in some cases: CONFIG_MMC_UNSAFE_RESUME=n (default): Cards are assumed to be removed during suspend. Any filesystem on them must be unmounted before suspend; otherwise, buffered writes will be lost. CONFIG_MMC_UNSAFE_RESUME=y: Cards are assumed to remain present during suspend. They must not be swapped during suspend; otherwise, buffered writes will be flushed to the wrong card. Currently the choice is made at compile time and this allows that to be overridden at module load time. Signed-off-by: Ben Hutchings <ben@decadent.org.uk> Cc: Wouter van Heyst <larstiq@larstiq.dyndns.org> Cc: <linux-mmc@vger.kernel.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
69 lines
2 KiB
C
69 lines
2 KiB
C
/*
|
|
* linux/drivers/mmc/core/core.h
|
|
*
|
|
* Copyright (C) 2003 Russell King, All Rights Reserved.
|
|
* Copyright 2007 Pierre Ossman
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#ifndef _MMC_CORE_CORE_H
|
|
#define _MMC_CORE_CORE_H
|
|
|
|
#include <linux/delay.h>
|
|
|
|
#define MMC_CMD_RETRIES 3
|
|
|
|
struct mmc_bus_ops {
|
|
int (*awake)(struct mmc_host *);
|
|
int (*sleep)(struct mmc_host *);
|
|
void (*remove)(struct mmc_host *);
|
|
void (*detect)(struct mmc_host *);
|
|
int (*suspend)(struct mmc_host *);
|
|
int (*resume)(struct mmc_host *);
|
|
void (*power_save)(struct mmc_host *);
|
|
void (*power_restore)(struct mmc_host *);
|
|
};
|
|
|
|
void mmc_attach_bus(struct mmc_host *host, const struct mmc_bus_ops *ops);
|
|
void mmc_detach_bus(struct mmc_host *host);
|
|
|
|
void mmc_set_chip_select(struct mmc_host *host, int mode);
|
|
void mmc_set_clock(struct mmc_host *host, unsigned int hz);
|
|
void mmc_set_bus_mode(struct mmc_host *host, unsigned int mode);
|
|
void mmc_set_bus_width(struct mmc_host *host, unsigned int width);
|
|
u32 mmc_select_voltage(struct mmc_host *host, u32 ocr);
|
|
void mmc_set_timing(struct mmc_host *host, unsigned int timing);
|
|
|
|
static inline void mmc_delay(unsigned int ms)
|
|
{
|
|
if (ms < 1000 / HZ) {
|
|
cond_resched();
|
|
mdelay(ms);
|
|
} else {
|
|
msleep(ms);
|
|
}
|
|
}
|
|
|
|
void mmc_rescan(struct work_struct *work);
|
|
void mmc_start_host(struct mmc_host *host);
|
|
void mmc_stop_host(struct mmc_host *host);
|
|
|
|
int mmc_attach_mmc(struct mmc_host *host, u32 ocr);
|
|
int mmc_attach_sd(struct mmc_host *host, u32 ocr);
|
|
int mmc_attach_sdio(struct mmc_host *host, u32 ocr);
|
|
|
|
/* Module parameters */
|
|
extern int use_spi_crc;
|
|
extern int mmc_assume_removable;
|
|
|
|
/* Debugfs information for hosts and cards */
|
|
void mmc_add_host_debugfs(struct mmc_host *host);
|
|
void mmc_remove_host_debugfs(struct mmc_host *host);
|
|
|
|
void mmc_add_card_debugfs(struct mmc_card *card);
|
|
void mmc_remove_card_debugfs(struct mmc_card *card);
|
|
|
|
#endif
|
|
|