mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 09:19:51 +00:00
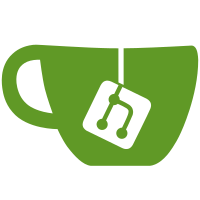
On TGL/RKL the BIOS likes to use some kind of bogus DBUF layout that doesn't match what the spec recommends. With a single active pipe that is not going to be a problem, but with multiple pipes active skl_commit_modeset_enables() goes into an infinite loop since it can't figure out any order in which it can commit the pipes without causing DBUF overlaps between the planes. We'd need some kind of extra DBUF defrag stage in between to make the transition possible. But that is clearly way too complex a solution, so in the name of simplicity let's just sanitize the DBUF state by simply turning off all planes when we detect a pipe encroaching on its neighbours' DBUF slices. We only have to disable the primary planes as all other planes should have already been disabled (if they somehow were enabled) by earlier sanitization steps. And for good measure let's also sanitize in case the DBUF allocations of the pipes already seem to overlap each other. Cc: <stable@vger.kernel.org> # v5.14+ Closes: https://gitlab.freedesktop.org/drm/intel/-/issues/4762 Signed-off-by: Ville Syrjälä <ville.syrjala@linux.intel.com> Link: https://patchwork.freedesktop.org/patch/msgid/20220204141818.1900-3-ville.syrjala@linux.intel.com Reviewed-by: Stanislav Lisovskiy <stanislav.lisovskiy@intel.com>
98 lines
3.8 KiB
C
98 lines
3.8 KiB
C
/* SPDX-License-Identifier: MIT */
|
|
/*
|
|
* Copyright © 2019 Intel Corporation
|
|
*/
|
|
|
|
#ifndef __INTEL_PM_H__
|
|
#define __INTEL_PM_H__
|
|
|
|
#include <linux/types.h>
|
|
|
|
#include "display/intel_display.h"
|
|
#include "display/intel_global_state.h"
|
|
|
|
#include "i915_drv.h"
|
|
|
|
struct drm_device;
|
|
struct drm_i915_private;
|
|
struct i915_request;
|
|
struct intel_atomic_state;
|
|
struct intel_bw_state;
|
|
struct intel_crtc;
|
|
struct intel_crtc_state;
|
|
struct intel_plane;
|
|
struct skl_ddb_entry;
|
|
struct skl_pipe_wm;
|
|
struct skl_wm_level;
|
|
|
|
void intel_init_clock_gating(struct drm_i915_private *dev_priv);
|
|
void intel_suspend_hw(struct drm_i915_private *dev_priv);
|
|
int ilk_wm_max_level(const struct drm_i915_private *dev_priv);
|
|
void intel_init_pm(struct drm_i915_private *dev_priv);
|
|
void intel_init_clock_gating_hooks(struct drm_i915_private *dev_priv);
|
|
void intel_pm_setup(struct drm_i915_private *dev_priv);
|
|
void g4x_wm_get_hw_state(struct drm_i915_private *dev_priv);
|
|
void vlv_wm_get_hw_state(struct drm_i915_private *dev_priv);
|
|
void ilk_wm_get_hw_state(struct drm_i915_private *dev_priv);
|
|
void skl_wm_get_hw_state(struct drm_i915_private *dev_priv);
|
|
u8 intel_enabled_dbuf_slices_mask(struct drm_i915_private *dev_priv);
|
|
void skl_pipe_ddb_get_hw_state(struct intel_crtc *crtc,
|
|
struct skl_ddb_entry *ddb_y,
|
|
struct skl_ddb_entry *ddb_uv);
|
|
void skl_ddb_get_hw_state(struct drm_i915_private *dev_priv);
|
|
u32 skl_ddb_dbuf_slice_mask(struct drm_i915_private *dev_priv,
|
|
const struct skl_ddb_entry *entry);
|
|
void skl_pipe_wm_get_hw_state(struct intel_crtc *crtc,
|
|
struct skl_pipe_wm *out);
|
|
void g4x_wm_sanitize(struct drm_i915_private *dev_priv);
|
|
void vlv_wm_sanitize(struct drm_i915_private *dev_priv);
|
|
void skl_wm_sanitize(struct drm_i915_private *dev_priv);
|
|
bool intel_can_enable_sagv(struct drm_i915_private *dev_priv,
|
|
const struct intel_bw_state *bw_state);
|
|
void intel_sagv_pre_plane_update(struct intel_atomic_state *state);
|
|
void intel_sagv_post_plane_update(struct intel_atomic_state *state);
|
|
const struct skl_wm_level *skl_plane_wm_level(const struct skl_pipe_wm *pipe_wm,
|
|
enum plane_id plane_id,
|
|
int level);
|
|
const struct skl_wm_level *skl_plane_trans_wm(const struct skl_pipe_wm *pipe_wm,
|
|
enum plane_id plane_id);
|
|
bool skl_wm_level_equals(const struct skl_wm_level *l1,
|
|
const struct skl_wm_level *l2);
|
|
bool skl_ddb_allocation_overlaps(const struct skl_ddb_entry *ddb,
|
|
const struct skl_ddb_entry *entries,
|
|
int num_entries, int ignore_idx);
|
|
void skl_write_plane_wm(struct intel_plane *plane,
|
|
const struct intel_crtc_state *crtc_state);
|
|
void skl_write_cursor_wm(struct intel_plane *plane,
|
|
const struct intel_crtc_state *crtc_state);
|
|
bool ilk_disable_lp_wm(struct drm_i915_private *dev_priv);
|
|
void intel_init_ipc(struct drm_i915_private *dev_priv);
|
|
void intel_enable_ipc(struct drm_i915_private *dev_priv);
|
|
|
|
bool intel_set_memory_cxsr(struct drm_i915_private *dev_priv, bool enable);
|
|
|
|
struct intel_dbuf_state {
|
|
struct intel_global_state base;
|
|
|
|
struct skl_ddb_entry ddb[I915_MAX_PIPES];
|
|
unsigned int weight[I915_MAX_PIPES];
|
|
u8 slices[I915_MAX_PIPES];
|
|
u8 enabled_slices;
|
|
u8 active_pipes;
|
|
bool joined_mbus;
|
|
};
|
|
|
|
struct intel_dbuf_state *
|
|
intel_atomic_get_dbuf_state(struct intel_atomic_state *state);
|
|
|
|
#define to_intel_dbuf_state(x) container_of((x), struct intel_dbuf_state, base)
|
|
#define intel_atomic_get_old_dbuf_state(state) \
|
|
to_intel_dbuf_state(intel_atomic_get_old_global_obj_state(state, &to_i915(state->base.dev)->dbuf.obj))
|
|
#define intel_atomic_get_new_dbuf_state(state) \
|
|
to_intel_dbuf_state(intel_atomic_get_new_global_obj_state(state, &to_i915(state->base.dev)->dbuf.obj))
|
|
|
|
int intel_dbuf_init(struct drm_i915_private *dev_priv);
|
|
void intel_dbuf_pre_plane_update(struct intel_atomic_state *state);
|
|
void intel_dbuf_post_plane_update(struct intel_atomic_state *state);
|
|
|
|
#endif /* __INTEL_PM_H__ */
|