mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
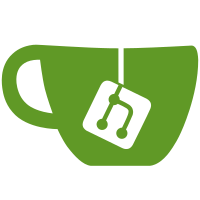
Instead of using shared global variables between userspace and BPF, use the ring buffer to send the IMA hash on the BPF ring buffer. This helps in validating both IMA and the usage of the ringbuffer in sleepable programs. Signed-off-by: KP Singh <kpsingh@kernel.org> Signed-off-by: Andrii Nakryiko <andrii@kernel.org> Link: https://lore.kernel.org/bpf/20210204193622.3367275-3-kpsingh@kernel.org
45 lines
830 B
C
45 lines
830 B
C
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
/*
|
|
* Copyright 2020 Google LLC.
|
|
*/
|
|
|
|
#include "vmlinux.h"
|
|
#include <errno.h>
|
|
#include <bpf/bpf_helpers.h>
|
|
#include <bpf/bpf_tracing.h>
|
|
|
|
u32 monitored_pid = 0;
|
|
|
|
struct {
|
|
__uint(type, BPF_MAP_TYPE_RINGBUF);
|
|
__uint(max_entries, 1 << 12);
|
|
} ringbuf SEC(".maps");
|
|
|
|
char _license[] SEC("license") = "GPL";
|
|
|
|
SEC("lsm.s/bprm_committed_creds")
|
|
void BPF_PROG(ima, struct linux_binprm *bprm)
|
|
{
|
|
u64 ima_hash = 0;
|
|
u64 *sample;
|
|
int ret;
|
|
u32 pid;
|
|
|
|
pid = bpf_get_current_pid_tgid() >> 32;
|
|
if (pid == monitored_pid) {
|
|
ret = bpf_ima_inode_hash(bprm->file->f_inode, &ima_hash,
|
|
sizeof(ima_hash));
|
|
if (ret < 0 || ima_hash == 0)
|
|
return;
|
|
|
|
sample = bpf_ringbuf_reserve(&ringbuf, sizeof(u64), 0);
|
|
if (!sample)
|
|
return;
|
|
|
|
*sample = ima_hash;
|
|
bpf_ringbuf_submit(sample, 0);
|
|
}
|
|
|
|
return;
|
|
}
|