mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
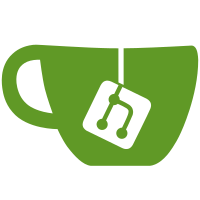
The authors of this tool were more familiar with a different type-checker, https://github.com/google/pytype. That's open source, but mypy seems more prevalent (and runs faster). And unlike pytype, mypy doesn't try to infer types so it doesn't check unanotated functions. So annotate ~all functions in kunit tool to increase type-checking coverage. Note: per https://www.python.org/dev/peps/pep-0484/, `__init__()` should be annotated as `-> None`. Doing so makes mypy discover a number of new violations. Exclude main() since we reuse `request` for the different types of requests, which mypy isn't happy about. This commit fixes all but one error, where `TestSuite.status` might be None. Signed-off-by: Daniel Latypov <dlatypov@google.com> Reviewed-by: David Gow <davidgow@google.com> Tested-by: Brendan Higgins <brendanhiggins@google.com> Acked-by: Brendan Higgins <brendanhiggins@google.com> Signed-off-by: Shuah Khan <skhan@linuxfoundation.org>
63 lines
1.8 KiB
Python
63 lines
1.8 KiB
Python
# SPDX-License-Identifier: GPL-2.0
|
|
#
|
|
# Generates JSON from KUnit results according to
|
|
# KernelCI spec: https://github.com/kernelci/kernelci-doc/wiki/Test-API
|
|
#
|
|
# Copyright (C) 2020, Google LLC.
|
|
# Author: Heidi Fahim <heidifahim@google.com>
|
|
|
|
import json
|
|
import os
|
|
|
|
import kunit_parser
|
|
|
|
from kunit_parser import TestStatus
|
|
|
|
def get_json_result(test_result, def_config, build_dir, json_path) -> str:
|
|
sub_groups = []
|
|
|
|
# Each test suite is mapped to a KernelCI sub_group
|
|
for test_suite in test_result.suites:
|
|
sub_group = {
|
|
"name": test_suite.name,
|
|
"arch": "UM",
|
|
"defconfig": def_config,
|
|
"build_environment": build_dir,
|
|
"test_cases": [],
|
|
"lab_name": None,
|
|
"kernel": None,
|
|
"job": None,
|
|
"git_branch": "kselftest",
|
|
}
|
|
test_cases = []
|
|
# TODO: Add attachments attribute in test_case with detailed
|
|
# failure message, see https://api.kernelci.org/schema-test-case.html#get
|
|
for case in test_suite.cases:
|
|
test_case = {"name": case.name, "status": "FAIL"}
|
|
if case.status == TestStatus.SUCCESS:
|
|
test_case["status"] = "PASS"
|
|
elif case.status == TestStatus.TEST_CRASHED:
|
|
test_case["status"] = "ERROR"
|
|
test_cases.append(test_case)
|
|
sub_group["test_cases"] = test_cases
|
|
sub_groups.append(sub_group)
|
|
test_group = {
|
|
"name": "KUnit Test Group",
|
|
"arch": "UM",
|
|
"defconfig": def_config,
|
|
"build_environment": build_dir,
|
|
"sub_groups": sub_groups,
|
|
"lab_name": None,
|
|
"kernel": None,
|
|
"job": None,
|
|
"git_branch": "kselftest",
|
|
}
|
|
json_obj = json.dumps(test_group, indent=4)
|
|
if json_path != 'stdout':
|
|
with open(json_path, 'w') as result_path:
|
|
result_path.write(json_obj)
|
|
root = __file__.split('tools/testing/kunit/')[0]
|
|
kunit_parser.print_with_timestamp(
|
|
"Test results stored in %s" %
|
|
os.path.join(root, result_path.name))
|
|
return json_obj
|