mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 23:25:07 +00:00
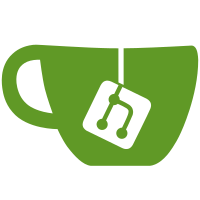
Recent Intel chipsets including Skylake and ApolloLake have a special ITSSPRC register which allows the 8254 PIT to be gated. When gated, the 8254 registers can still be programmed as normal, but there are no IRQ0 timer interrupts. Some products such as the Connex L1430 and exone go Rugged E11 use this register to ship with the PIT gated by default. This causes Linux to fail to boot: Kernel panic - not syncing: IO-APIC + timer doesn't work! Boot with apic=debug and send a report. The panic happens before the framebuffer is initialized, so to the user, it appears as an early boot hang on a black screen. Affected products typically have a BIOS option that can be used to enable the 8254 and make Linux work (Chipset -> South Cluster Configuration -> Miscellaneous Configuration -> 8254 Clock Gating), however it would be best to make Linux support the no-8254 case. Modern sytems allow to discover the TSC and local APIC timer frequencies, so the calibration against the PIT is not required. These systems have always running timers and the local APIC timer works also in deep power states. So the setup of the PIT including the IO-APIC timer interrupt delivery checks are a pointless exercise. Skip the PIT setup and the IO-APIC timer interrupt checks on these systems, which avoids the panic caused by non ticking PITs and also speeds up the boot process. Thanks to Daniel for providing the changelog, initial analysis of the problem and testing against a variety of machines. Reported-by: Daniel Drake <drake@endlessm.com> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Tested-by: Daniel Drake <drake@endlessm.com> Cc: bp@alien8.de Cc: hpa@zytor.com Cc: linux@endlessm.com Cc: rafael.j.wysocki@intel.com Cc: hdegoede@redhat.com Link: https://lkml.kernel.org/r/20190628072307.24678-1-drake@endlessm.com
132 lines
3.1 KiB
C
132 lines
3.1 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 1991,1992,1995 Linus Torvalds
|
|
* Copyright (c) 1994 Alan Modra
|
|
* Copyright (c) 1995 Markus Kuhn
|
|
* Copyright (c) 1996 Ingo Molnar
|
|
* Copyright (c) 1998 Andrea Arcangeli
|
|
* Copyright (c) 2002,2006 Vojtech Pavlik
|
|
* Copyright (c) 2003 Andi Kleen
|
|
*
|
|
*/
|
|
|
|
#include <linux/clocksource.h>
|
|
#include <linux/clockchips.h>
|
|
#include <linux/interrupt.h>
|
|
#include <linux/irq.h>
|
|
#include <linux/i8253.h>
|
|
#include <linux/time.h>
|
|
#include <linux/export.h>
|
|
|
|
#include <asm/vsyscall.h>
|
|
#include <asm/x86_init.h>
|
|
#include <asm/i8259.h>
|
|
#include <asm/timer.h>
|
|
#include <asm/hpet.h>
|
|
#include <asm/time.h>
|
|
|
|
#ifdef CONFIG_X86_64
|
|
__visible volatile unsigned long jiffies __cacheline_aligned_in_smp = INITIAL_JIFFIES;
|
|
#endif
|
|
|
|
unsigned long profile_pc(struct pt_regs *regs)
|
|
{
|
|
unsigned long pc = instruction_pointer(regs);
|
|
|
|
if (!user_mode(regs) && in_lock_functions(pc)) {
|
|
#ifdef CONFIG_FRAME_POINTER
|
|
return *(unsigned long *)(regs->bp + sizeof(long));
|
|
#else
|
|
unsigned long *sp =
|
|
(unsigned long *)kernel_stack_pointer(regs);
|
|
/*
|
|
* Return address is either directly at stack pointer
|
|
* or above a saved flags. Eflags has bits 22-31 zero,
|
|
* kernel addresses don't.
|
|
*/
|
|
if (sp[0] >> 22)
|
|
return sp[0];
|
|
if (sp[1] >> 22)
|
|
return sp[1];
|
|
#endif
|
|
}
|
|
return pc;
|
|
}
|
|
EXPORT_SYMBOL(profile_pc);
|
|
|
|
/*
|
|
* Default timer interrupt handler for PIT/HPET
|
|
*/
|
|
static irqreturn_t timer_interrupt(int irq, void *dev_id)
|
|
{
|
|
global_clock_event->event_handler(global_clock_event);
|
|
return IRQ_HANDLED;
|
|
}
|
|
|
|
static struct irqaction irq0 = {
|
|
.handler = timer_interrupt,
|
|
.flags = IRQF_NOBALANCING | IRQF_IRQPOLL | IRQF_TIMER,
|
|
.name = "timer"
|
|
};
|
|
|
|
static void __init setup_default_timer_irq(void)
|
|
{
|
|
/*
|
|
* Unconditionally register the legacy timer; even without legacy
|
|
* PIC/PIT we need this for the HPET0 in legacy replacement mode.
|
|
*/
|
|
if (setup_irq(0, &irq0))
|
|
pr_info("Failed to register legacy timer interrupt\n");
|
|
}
|
|
|
|
/* Default timer init function */
|
|
void __init hpet_time_init(void)
|
|
{
|
|
if (!hpet_enable()) {
|
|
if (!pit_timer_init())
|
|
return;
|
|
}
|
|
|
|
setup_default_timer_irq();
|
|
}
|
|
|
|
static __init void x86_late_time_init(void)
|
|
{
|
|
x86_init.timers.timer_init();
|
|
/*
|
|
* After PIT/HPET timers init, select and setup
|
|
* the final interrupt mode for delivering IRQs.
|
|
*/
|
|
x86_init.irqs.intr_mode_init();
|
|
tsc_init();
|
|
}
|
|
|
|
/*
|
|
* Initialize TSC and delay the periodic timer init to
|
|
* late x86_late_time_init() so ioremap works.
|
|
*/
|
|
void __init time_init(void)
|
|
{
|
|
late_time_init = x86_late_time_init;
|
|
}
|
|
|
|
/*
|
|
* Sanity check the vdso related archdata content.
|
|
*/
|
|
void clocksource_arch_init(struct clocksource *cs)
|
|
{
|
|
if (cs->archdata.vclock_mode == VCLOCK_NONE)
|
|
return;
|
|
|
|
if (cs->archdata.vclock_mode > VCLOCK_MAX) {
|
|
pr_warn("clocksource %s registered with invalid vclock_mode %d. Disabling vclock.\n",
|
|
cs->name, cs->archdata.vclock_mode);
|
|
cs->archdata.vclock_mode = VCLOCK_NONE;
|
|
}
|
|
|
|
if (cs->mask != CLOCKSOURCE_MASK(64)) {
|
|
pr_warn("clocksource %s registered with invalid mask %016llx. Disabling vclock.\n",
|
|
cs->name, cs->mask);
|
|
cs->archdata.vclock_mode = VCLOCK_NONE;
|
|
}
|
|
}
|