mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
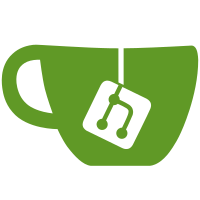
Added inline helper functions to check authsize and assoclen for gcm, rfc4106 and rfc4543. These are used in the generic implementation of gcm, rfc4106 and rfc4543. Signed-off-by: Iuliana Prodan <iuliana.prodan@nxp.com> Signed-off-by: Herbert Xu <herbert@gondor.apana.org.au>
63 lines
867 B
C
63 lines
867 B
C
#ifndef _CRYPTO_GCM_H
|
|
#define _CRYPTO_GCM_H
|
|
|
|
#include <linux/errno.h>
|
|
|
|
#define GCM_AES_IV_SIZE 12
|
|
#define GCM_RFC4106_IV_SIZE 8
|
|
#define GCM_RFC4543_IV_SIZE 8
|
|
|
|
/*
|
|
* validate authentication tag for GCM
|
|
*/
|
|
static inline int crypto_gcm_check_authsize(unsigned int authsize)
|
|
{
|
|
switch (authsize) {
|
|
case 4:
|
|
case 8:
|
|
case 12:
|
|
case 13:
|
|
case 14:
|
|
case 15:
|
|
case 16:
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* validate authentication tag for RFC4106
|
|
*/
|
|
static inline int crypto_rfc4106_check_authsize(unsigned int authsize)
|
|
{
|
|
switch (authsize) {
|
|
case 8:
|
|
case 12:
|
|
case 16:
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* validate assoclen for RFC4106/RFC4543
|
|
*/
|
|
static inline int crypto_ipsec_check_assoclen(unsigned int assoclen)
|
|
{
|
|
switch (assoclen) {
|
|
case 16:
|
|
case 20:
|
|
break;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
#endif
|