mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 01:41:39 +00:00
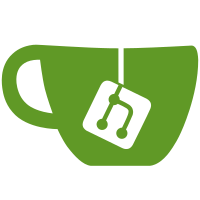
We have a I915_REQUEST_NOPREEMPT flag that we set when we must prevent the HW from preempting during the course of this request. We need to honour this flag and protect the HW even if we have a heartbeat request, or other maximum priority barrier, pending. As such, restrict the timeslicing check to avoid preempting into the topmost priority band, leaving the unpreemptable requests in blissful peace running uninterrupted on the HW. v2: Set the I915_PRIORITY_BARRIER to be less than I915_PRIORITY_UNPREEMPTABLE so that we never submit a request (heartbeat or barrier) that can legitimately preempt the current non-premptable request. Fixes:2a98f4e65b
("drm/i915: add infrastructure to hold off preemption on a request") Signed-off-by: Chris Wilson <chris@chris-wilson.co.uk> Cc: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Reviewed-by: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Link: https://patchwork.freedesktop.org/patch/msgid/20200527162418.24755-1-chris@chris-wilson.co.uk (cherry picked from commitb72f02d78e
) Signed-off-by: Joonas Lahtinen <joonas.lahtinen@linux.intel.com>
54 lines
1.5 KiB
C
54 lines
1.5 KiB
C
/*
|
|
* SPDX-License-Identifier: MIT
|
|
*
|
|
* Copyright © 2018 Intel Corporation
|
|
*/
|
|
|
|
#ifndef _I915_PRIOLIST_TYPES_H_
|
|
#define _I915_PRIOLIST_TYPES_H_
|
|
|
|
#include <linux/list.h>
|
|
#include <linux/rbtree.h>
|
|
|
|
#include <uapi/drm/i915_drm.h>
|
|
|
|
enum {
|
|
I915_PRIORITY_MIN = I915_CONTEXT_MIN_USER_PRIORITY - 1,
|
|
I915_PRIORITY_NORMAL = I915_CONTEXT_DEFAULT_PRIORITY,
|
|
I915_PRIORITY_MAX = I915_CONTEXT_MAX_USER_PRIORITY + 1,
|
|
|
|
/* A preemptive pulse used to monitor the health of each engine */
|
|
I915_PRIORITY_HEARTBEAT,
|
|
|
|
/* Interactive workload, scheduled for immediate pageflipping */
|
|
I915_PRIORITY_DISPLAY,
|
|
};
|
|
|
|
#define I915_USER_PRIORITY_SHIFT 0
|
|
#define I915_USER_PRIORITY(x) ((x) << I915_USER_PRIORITY_SHIFT)
|
|
|
|
#define I915_PRIORITY_COUNT BIT(I915_USER_PRIORITY_SHIFT)
|
|
#define I915_PRIORITY_MASK (I915_PRIORITY_COUNT - 1)
|
|
|
|
/* Smallest priority value that cannot be bumped. */
|
|
#define I915_PRIORITY_INVALID (INT_MIN | (u8)I915_PRIORITY_MASK)
|
|
|
|
/*
|
|
* Requests containing performance queries must not be preempted by
|
|
* another context. They get scheduled with their default priority and
|
|
* once they reach the execlist ports we ensure that they stick on the
|
|
* HW until finished by pretending that they have maximum priority,
|
|
* i.e. nothing can have higher priority and force us to usurp the
|
|
* active request.
|
|
*/
|
|
#define I915_PRIORITY_UNPREEMPTABLE INT_MAX
|
|
#define I915_PRIORITY_BARRIER (I915_PRIORITY_UNPREEMPTABLE - 1)
|
|
|
|
struct i915_priolist {
|
|
struct list_head requests[I915_PRIORITY_COUNT];
|
|
struct rb_node node;
|
|
unsigned long used;
|
|
int priority;
|
|
};
|
|
|
|
#endif /* _I915_PRIOLIST_TYPES_H_ */
|