mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 01:41:39 +00:00
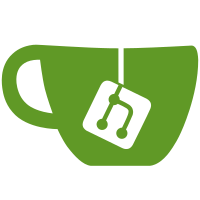
We recorded the dependencies for WAIT_FOR_SUBMIT in order that we could correctly perform priority inheritance from the parallel branches to the common trunk. However, for the purpose of timeslicing and reset handling, the dependency is weak -- as we the pair of requests are allowed to run in parallel and not in strict succession. The real significance though is that this allows us to rearrange groups of WAIT_FOR_SUBMIT linked requests along the single engine, and so can resolve user level inter-batch scheduling dependencies from user semaphores. Fixes:c81471f5e9
("drm/i915: Copy across scheduler behaviour flags across submit fences") Testcase: igt/gem_exec_fence/submit Signed-off-by: Chris Wilson <chris@chris-wilson.co.uk> Cc: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Cc: <stable@vger.kernel.org> # v5.6+ Reviewed-by: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Link: https://patchwork.freedesktop.org/patch/msgid/20200507155109.8892-1-chris@chris-wilson.co.uk (cherry picked from commit6b6cd2ebd8
) Signed-off-by: Rodrigo Vivi <rodrigo.vivi@intel.com>
57 lines
1.6 KiB
C
57 lines
1.6 KiB
C
/*
|
|
* SPDX-License-Identifier: MIT
|
|
*
|
|
* Copyright © 2018 Intel Corporation
|
|
*/
|
|
|
|
#ifndef _I915_SCHEDULER_H_
|
|
#define _I915_SCHEDULER_H_
|
|
|
|
#include <linux/bitops.h>
|
|
#include <linux/list.h>
|
|
#include <linux/kernel.h>
|
|
|
|
#include "i915_scheduler_types.h"
|
|
|
|
#define priolist_for_each_request(it, plist, idx) \
|
|
for (idx = 0; idx < ARRAY_SIZE((plist)->requests); idx++) \
|
|
list_for_each_entry(it, &(plist)->requests[idx], sched.link)
|
|
|
|
#define priolist_for_each_request_consume(it, n, plist, idx) \
|
|
for (; \
|
|
(plist)->used ? (idx = __ffs((plist)->used)), 1 : 0; \
|
|
(plist)->used &= ~BIT(idx)) \
|
|
list_for_each_entry_safe(it, n, \
|
|
&(plist)->requests[idx], \
|
|
sched.link)
|
|
|
|
void i915_sched_node_init(struct i915_sched_node *node);
|
|
void i915_sched_node_reinit(struct i915_sched_node *node);
|
|
|
|
bool __i915_sched_node_add_dependency(struct i915_sched_node *node,
|
|
struct i915_sched_node *signal,
|
|
struct i915_dependency *dep,
|
|
unsigned long flags);
|
|
|
|
int i915_sched_node_add_dependency(struct i915_sched_node *node,
|
|
struct i915_sched_node *signal,
|
|
unsigned long flags);
|
|
|
|
void i915_sched_node_fini(struct i915_sched_node *node);
|
|
|
|
void i915_schedule(struct i915_request *request,
|
|
const struct i915_sched_attr *attr);
|
|
|
|
void i915_schedule_bump_priority(struct i915_request *rq, unsigned int bump);
|
|
|
|
struct list_head *
|
|
i915_sched_lookup_priolist(struct intel_engine_cs *engine, int prio);
|
|
|
|
void __i915_priolist_free(struct i915_priolist *p);
|
|
static inline void i915_priolist_free(struct i915_priolist *p)
|
|
{
|
|
if (p->priority != I915_PRIORITY_NORMAL)
|
|
__i915_priolist_free(p);
|
|
}
|
|
|
|
#endif /* _I915_SCHEDULER_H_ */
|