mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-30 06:10:56 +00:00
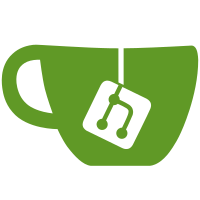
Prior to IPA v5.0, there could be no more than 32 endpoints. A filter table begins with a bitmap indicating which endpoints have a filter defined. That bitmap is currently assumed to fit in a 32-bit value. Starting with IPA v5.0, more than 32 endpoints are supported, so it's conceivable that a TX endpoint has an ID that exceeds 32. Increase the size of the field representing endpoints that support filtering to 64 bits. Rename the bitmap field "filtered". Unlike other similar fields, we do not use an (arbitrarily long) Linux bitmap for this purpose. The reason is that if a filter table ever *did* need to support more than 64 TX endpoints, its format would change in ways we can't anticipate. Have ipa_endpoint_init() return a negative errno rather than a mask that indicates which endpoints support filtering, and have that function assign the "filtered" field directly. Signed-off-by: Alex Elder <elder@linaro.org> Signed-off-by: David S. Miller <davem@davemloft.net>
79 lines
1.9 KiB
C
79 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
|
|
/* Copyright (c) 2012-2018, The Linux Foundation. All rights reserved.
|
|
* Copyright (C) 2019-2022 Linaro Ltd.
|
|
*/
|
|
#ifndef _IPA_TABLE_H_
|
|
#define _IPA_TABLE_H_
|
|
|
|
#include <linux/types.h>
|
|
|
|
struct ipa;
|
|
|
|
/**
|
|
* ipa_filtered_valid() - Validate a filter table endpoint bitmap
|
|
* @ipa: IPA pointer
|
|
* @filtered: Filter table endpoint bitmap to check
|
|
*
|
|
* Return: true if all regions are valid, false otherwise
|
|
*/
|
|
bool ipa_filtered_valid(struct ipa *ipa, u64 filtered);
|
|
|
|
/**
|
|
* ipa_table_hash_support() - Return true if hashed tables are supported
|
|
* @ipa: IPA pointer
|
|
*/
|
|
static inline bool ipa_table_hash_support(struct ipa *ipa)
|
|
{
|
|
return ipa->version != IPA_VERSION_4_2;
|
|
}
|
|
|
|
/**
|
|
* ipa_table_reset() - Reset filter and route tables entries to "none"
|
|
* @ipa: IPA pointer
|
|
* @modem: Whether to reset modem or AP entries
|
|
*/
|
|
void ipa_table_reset(struct ipa *ipa, bool modem);
|
|
|
|
/**
|
|
* ipa_table_hash_flush() - Synchronize hashed filter and route updates
|
|
* @ipa: IPA pointer
|
|
*/
|
|
int ipa_table_hash_flush(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_setup() - Set up filter and route tables
|
|
* @ipa: IPA pointer
|
|
*
|
|
* There is no need for a matching ipa_table_teardown() function.
|
|
*/
|
|
int ipa_table_setup(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_config() - Configure filter and route tables
|
|
* @ipa: IPA pointer
|
|
*
|
|
* There is no need for a matching ipa_table_deconfig() function.
|
|
*/
|
|
void ipa_table_config(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_init() - Do early initialization of filter and route tables
|
|
* @ipa: IPA pointer
|
|
*/
|
|
int ipa_table_init(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_exit() - Inverse of ipa_table_init()
|
|
* @ipa: IPA pointer
|
|
*/
|
|
void ipa_table_exit(struct ipa *ipa);
|
|
|
|
/**
|
|
* ipa_table_mem_valid() - Validate sizes of table memory regions
|
|
* @ipa: IPA pointer
|
|
* @filter: Whether to check filter or routing tables
|
|
*/
|
|
bool ipa_table_mem_valid(struct ipa *ipa, bool filter);
|
|
|
|
#endif /* _IPA_TABLE_H_ */
|