mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 00:40:03 +00:00
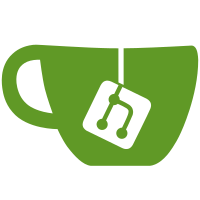
The MDS tries to enforce a limit on the total key/values in extended attributes. However, this limit is enforced only if doing a synchronous operation (MDS_OP_SETXATTR) -- if we're buffering the xattrs, the MDS doesn't have a chance to enforce these limits. This patch adds support for decoding the xattrs maximum size setting that is distributed in the mdsmap. Then, when setting an xattr, the kernel client will revert to do a synchronous operation if that maximum size is exceeded. While there, fix a dout() that would trigger a printk warning: [ 98.718078] ------------[ cut here ]------------ [ 98.719012] precision 65536 too large [ 98.719039] WARNING: CPU: 1 PID: 3755 at lib/vsprintf.c:2703 vsnprintf+0x5e3/0x600 ... Link: https://tracker.ceph.com/issues/55725 Signed-off-by: Luís Henriques <lhenriques@suse.de> Reviewed-by: Xiubo Li <xiubli@redhat.com> Signed-off-by: Ilya Dryomov <idryomov@gmail.com>
72 lines
1.8 KiB
C
72 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _FS_CEPH_MDSMAP_H
|
|
#define _FS_CEPH_MDSMAP_H
|
|
|
|
#include <linux/bug.h>
|
|
#include <linux/ceph/types.h>
|
|
|
|
/*
|
|
* mds map - describe servers in the mds cluster.
|
|
*
|
|
* we limit fields to those the client actually xcares about
|
|
*/
|
|
struct ceph_mds_info {
|
|
u64 global_id;
|
|
struct ceph_entity_addr addr;
|
|
s32 state;
|
|
int num_export_targets;
|
|
bool laggy;
|
|
u32 *export_targets;
|
|
};
|
|
|
|
struct ceph_mdsmap {
|
|
u32 m_epoch, m_client_epoch, m_last_failure;
|
|
u32 m_root;
|
|
u32 m_session_timeout; /* seconds */
|
|
u32 m_session_autoclose; /* seconds */
|
|
u64 m_max_file_size;
|
|
u64 m_max_xattr_size; /* maximum size for xattrs blob */
|
|
u32 m_max_mds; /* expected up:active mds number */
|
|
u32 m_num_active_mds; /* actual up:active mds number */
|
|
u32 possible_max_rank; /* possible max rank index */
|
|
struct ceph_mds_info *m_info;
|
|
|
|
/* which object pools file data can be stored in */
|
|
int m_num_data_pg_pools;
|
|
u64 *m_data_pg_pools;
|
|
u64 m_cas_pg_pool;
|
|
|
|
bool m_enabled;
|
|
bool m_damaged;
|
|
int m_num_laggy;
|
|
};
|
|
|
|
static inline struct ceph_entity_addr *
|
|
ceph_mdsmap_get_addr(struct ceph_mdsmap *m, int w)
|
|
{
|
|
if (w >= m->possible_max_rank)
|
|
return NULL;
|
|
return &m->m_info[w].addr;
|
|
}
|
|
|
|
static inline int ceph_mdsmap_get_state(struct ceph_mdsmap *m, int w)
|
|
{
|
|
BUG_ON(w < 0);
|
|
if (w >= m->possible_max_rank)
|
|
return CEPH_MDS_STATE_DNE;
|
|
return m->m_info[w].state;
|
|
}
|
|
|
|
static inline bool ceph_mdsmap_is_laggy(struct ceph_mdsmap *m, int w)
|
|
{
|
|
if (w >= 0 && w < m->possible_max_rank)
|
|
return m->m_info[w].laggy;
|
|
return false;
|
|
}
|
|
|
|
extern int ceph_mdsmap_get_random_mds(struct ceph_mdsmap *m);
|
|
struct ceph_mdsmap *ceph_mdsmap_decode(void **p, void *end, bool msgr2);
|
|
extern void ceph_mdsmap_destroy(struct ceph_mdsmap *m);
|
|
extern bool ceph_mdsmap_is_cluster_available(struct ceph_mdsmap *m);
|
|
|
|
#endif
|