mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 09:50:04 +00:00
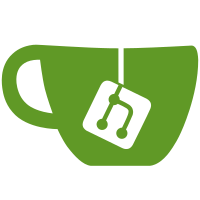
Before CXL 2.0 HDM Decoder Capability mechanisms can be utilized in a device the driver must determine that the device is ready for CXL.mem operation and that platform firmware, or some other agent, has established an active decode via the legacy CXL 1.1 decoder mechanism. This legacy mechanism is defined in the CXL DVSEC as a set of range registers and status bits that take time to settle after a reset. Validate the CXL memory decode setup via the DVSEC and cache it for later consideration by the cxl_mem driver (to be added). Failure to validate is not fatal to the cxl_pci driver since that is only providing CXL command support over PCI.mmio, and might be needed to rectify CXL DVSEC validation problems. Any potential ranges that the device is already claiming via DVSEC need to be reconciled with the dynamic provisioning ranges provided by platform firmware (like ACPI CEDT.CFMWS). Leave that reconciliation to the cxl_mem driver. [djbw: shorten defines] [djbw: change precise spin wait to generous msleep] Reported-by: kernel test robot <lkp@intel.com> Signed-off-by: Ben Widawsky <ben.widawsky@intel.com> [djbw: clarify changelog] Reviewed-by: Jonathan Cameron <Jonathan.Cameron@huawei.com> Link: https://lore.kernel.org/r/164375911821.559935.7375160041663453400.stgit@dwillia2-desk3.amr.corp.intel.com Signed-off-by: Dan Williams <dan.j.williams@intel.com>
75 lines
2.5 KiB
C
75 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/* Copyright(c) 2020 Intel Corporation. All rights reserved. */
|
|
#ifndef __CXL_PCI_H__
|
|
#define __CXL_PCI_H__
|
|
#include <linux/pci.h>
|
|
#include "cxl.h"
|
|
|
|
#define CXL_MEMORY_PROGIF 0x10
|
|
|
|
/*
|
|
* See section 8.1 Configuration Space Registers in the CXL 2.0
|
|
* Specification. Names are taken straight from the specification with "CXL" and
|
|
* "DVSEC" redundancies removed. When obvious, abbreviations may be used.
|
|
*/
|
|
#define PCI_DVSEC_HEADER1_LENGTH_MASK GENMASK(31, 20)
|
|
#define PCI_DVSEC_VENDOR_ID_CXL 0x1E98
|
|
|
|
/* CXL 2.0 8.1.3: PCIe DVSEC for CXL Device */
|
|
#define CXL_DVSEC_PCIE_DEVICE 0
|
|
#define CXL_DVSEC_CAP_OFFSET 0xA
|
|
#define CXL_DVSEC_MEM_CAPABLE BIT(2)
|
|
#define CXL_DVSEC_HDM_COUNT_MASK GENMASK(5, 4)
|
|
#define CXL_DVSEC_CTRL_OFFSET 0xC
|
|
#define CXL_DVSEC_MEM_ENABLE BIT(2)
|
|
#define CXL_DVSEC_RANGE_SIZE_HIGH(i) (0x18 + (i * 0x10))
|
|
#define CXL_DVSEC_RANGE_SIZE_LOW(i) (0x1C + (i * 0x10))
|
|
#define CXL_DVSEC_MEM_INFO_VALID BIT(0)
|
|
#define CXL_DVSEC_MEM_ACTIVE BIT(1)
|
|
#define CXL_DVSEC_MEM_SIZE_LOW_MASK GENMASK(31, 28)
|
|
#define CXL_DVSEC_RANGE_BASE_HIGH(i) (0x20 + (i * 0x10))
|
|
#define CXL_DVSEC_RANGE_BASE_LOW(i) (0x24 + (i * 0x10))
|
|
#define CXL_DVSEC_MEM_BASE_LOW_MASK GENMASK(31, 28)
|
|
|
|
/* CXL 2.0 8.1.4: Non-CXL Function Map DVSEC */
|
|
#define CXL_DVSEC_FUNCTION_MAP 2
|
|
|
|
/* CXL 2.0 8.1.5: CXL 2.0 Extensions DVSEC for Ports */
|
|
#define CXL_DVSEC_PORT_EXTENSIONS 3
|
|
|
|
/* CXL 2.0 8.1.6: GPF DVSEC for CXL Port */
|
|
#define CXL_DVSEC_PORT_GPF 4
|
|
|
|
/* CXL 2.0 8.1.7: GPF DVSEC for CXL Device */
|
|
#define CXL_DVSEC_DEVICE_GPF 5
|
|
|
|
/* CXL 2.0 8.1.8: PCIe DVSEC for Flex Bus Port */
|
|
#define CXL_DVSEC_PCIE_FLEXBUS_PORT 7
|
|
|
|
/* CXL 2.0 8.1.9: Register Locator DVSEC */
|
|
#define CXL_DVSEC_REG_LOCATOR 8
|
|
#define CXL_DVSEC_REG_LOCATOR_BLOCK1_OFFSET 0xC
|
|
#define CXL_DVSEC_REG_LOCATOR_BIR_MASK GENMASK(2, 0)
|
|
#define CXL_DVSEC_REG_LOCATOR_BLOCK_ID_MASK GENMASK(15, 8)
|
|
#define CXL_DVSEC_REG_LOCATOR_BLOCK_OFF_LOW_MASK GENMASK(31, 16)
|
|
|
|
/* Register Block Identifier (RBI) */
|
|
enum cxl_regloc_type {
|
|
CXL_REGLOC_RBI_EMPTY = 0,
|
|
CXL_REGLOC_RBI_COMPONENT,
|
|
CXL_REGLOC_RBI_VIRT,
|
|
CXL_REGLOC_RBI_MEMDEV,
|
|
CXL_REGLOC_RBI_TYPES
|
|
};
|
|
|
|
static inline resource_size_t cxl_regmap_to_base(struct pci_dev *pdev,
|
|
struct cxl_register_map *map)
|
|
{
|
|
if (map->block_offset == U64_MAX)
|
|
return CXL_RESOURCE_NONE;
|
|
|
|
return pci_resource_start(pdev, map->barno) + map->block_offset;
|
|
}
|
|
|
|
int devm_cxl_port_enumerate_dports(struct cxl_port *port);
|
|
#endif /* __CXL_PCI_H__ */
|