mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 21:57:43 +00:00
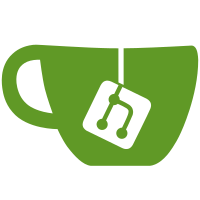
Add ECAM-related constants to provide a set of standard constants defining memory address shift values to the byte-level address that can be used to access the PCI Express Configuration Space, and then move native PCI Express controller drivers to use the newly introduced definitions retiring driver-specific ones. Refactor pci_ecam_map_bus() function to use newly added constants so that limits to the bus, device function and offset (now limited to 4K as per the specification) are in place to prevent the defective or malicious caller from supplying incorrect configuration offset and thus targeting the wrong device when accessing extended configuration space. This refactor also allows for the ".bus_shift" initialisers to be dropped when the user is not using a custom value as a default value will be used as per the PCI Express Specification. Thanks to Qian Cai <qcai@redhat.com>, Michael Walle <michael@walle.cc>, and Vladimir Oltean <olteanv@gmail.com> for reporting a pci_ecam_create() issue with .bus_shift and to Vladimir for proposing the fix. [bhelgaas: incorporate Vladimir's fix, update commit log] Suggested-by: Bjorn Helgaas <bhelgaas@google.com> Link: https://lore.kernel.org/r/20201129230743.3006978-2-kw@linux.com Tested-by: Michael Walle <michael@walle.cc> Signed-off-by: Krzysztof Wilczyński <kw@linux.com> Signed-off-by: Lorenzo Pieralisi <lorenzo.pieralisi@arm.com> Signed-off-by: Bjorn Helgaas <bhelgaas@google.com> Reviewed-by: Jon Derrick <jonathan.derrick@intel.com> Reviewed-by: Bjorn Helgaas <bhelgaas@google.com>
89 lines
2.2 KiB
C
89 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Simple, generic PCI host controller driver targeting firmware-initialised
|
|
* systems and virtual machines (e.g. the PCI emulation provided by kvmtool).
|
|
*
|
|
* Copyright (C) 2014 ARM Limited
|
|
*
|
|
* Author: Will Deacon <will.deacon@arm.com>
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/init.h>
|
|
#include <linux/module.h>
|
|
#include <linux/pci-ecam.h>
|
|
#include <linux/platform_device.h>
|
|
|
|
static const struct pci_ecam_ops gen_pci_cfg_cam_bus_ops = {
|
|
.bus_shift = 16,
|
|
.pci_ops = {
|
|
.map_bus = pci_ecam_map_bus,
|
|
.read = pci_generic_config_read,
|
|
.write = pci_generic_config_write,
|
|
}
|
|
};
|
|
|
|
static bool pci_dw_valid_device(struct pci_bus *bus, unsigned int devfn)
|
|
{
|
|
struct pci_config_window *cfg = bus->sysdata;
|
|
|
|
/*
|
|
* The Synopsys DesignWare PCIe controller in ECAM mode will not filter
|
|
* type 0 config TLPs sent to devices 1 and up on its downstream port,
|
|
* resulting in devices appearing multiple times on bus 0 unless we
|
|
* filter out those accesses here.
|
|
*/
|
|
if (bus->number == cfg->busr.start && PCI_SLOT(devfn) > 0)
|
|
return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
static void __iomem *pci_dw_ecam_map_bus(struct pci_bus *bus,
|
|
unsigned int devfn, int where)
|
|
{
|
|
if (!pci_dw_valid_device(bus, devfn))
|
|
return NULL;
|
|
|
|
return pci_ecam_map_bus(bus, devfn, where);
|
|
}
|
|
|
|
static const struct pci_ecam_ops pci_dw_ecam_bus_ops = {
|
|
.pci_ops = {
|
|
.map_bus = pci_dw_ecam_map_bus,
|
|
.read = pci_generic_config_read,
|
|
.write = pci_generic_config_write,
|
|
}
|
|
};
|
|
|
|
static const struct of_device_id gen_pci_of_match[] = {
|
|
{ .compatible = "pci-host-cam-generic",
|
|
.data = &gen_pci_cfg_cam_bus_ops },
|
|
|
|
{ .compatible = "pci-host-ecam-generic",
|
|
.data = &pci_generic_ecam_ops },
|
|
|
|
{ .compatible = "marvell,armada8k-pcie-ecam",
|
|
.data = &pci_dw_ecam_bus_ops },
|
|
|
|
{ .compatible = "socionext,synquacer-pcie-ecam",
|
|
.data = &pci_dw_ecam_bus_ops },
|
|
|
|
{ .compatible = "snps,dw-pcie-ecam",
|
|
.data = &pci_dw_ecam_bus_ops },
|
|
|
|
{ },
|
|
};
|
|
MODULE_DEVICE_TABLE(of, gen_pci_of_match);
|
|
|
|
static struct platform_driver gen_pci_driver = {
|
|
.driver = {
|
|
.name = "pci-host-generic",
|
|
.of_match_table = gen_pci_of_match,
|
|
},
|
|
.probe = pci_host_common_probe,
|
|
.remove = pci_host_common_remove,
|
|
};
|
|
module_platform_driver(gen_pci_driver);
|
|
|
|
MODULE_LICENSE("GPL v2");
|