mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 02:39:48 +00:00
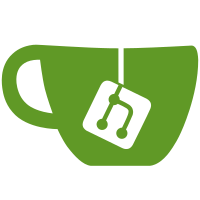
Remove the verbose license text from XFS files and replace them with SPDX tags. This does not change the license of any of the code, merely refers to the common, up-to-date license files in LICENSES/ This change was mostly scripted. fs/xfs/Makefile and fs/xfs/libxfs/xfs_fs.h were modified by hand, the rest were detected and modified by the following command: for f in `git grep -l "GNU General" fs/xfs/` ; do echo $f cat $f | awk -f hdr.awk > $f.new mv -f $f.new $f done And the hdr.awk script that did the modification (including detecting the difference between GPL-2.0 and GPL-2.0+ licenses) is as follows: $ cat hdr.awk BEGIN { hdr = 1.0 tag = "GPL-2.0" str = "" } /^ \* This program is free software/ { hdr = 2.0; next } /any later version./ { tag = "GPL-2.0+" next } /^ \*\// { if (hdr > 0.0) { print "// SPDX-License-Identifier: " tag print str print $0 str="" hdr = 0.0 next } print $0 next } /^ \* / { if (hdr > 1.0) next if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 next } /^ \*/ { if (hdr > 0.0) next print $0 next } // { if (hdr > 0.0) { if (str != "") str = str "\n" str = str $0 next } print $0 } END { } $ Signed-off-by: Dave Chinner <dchinner@redhat.com> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
97 lines
3.8 KiB
C
97 lines
3.8 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2002,2005 Silicon Graphics, Inc.
|
|
* All Rights Reserved.
|
|
*/
|
|
#ifndef __XFS_TRANS_RESV_H__
|
|
#define __XFS_TRANS_RESV_H__
|
|
|
|
struct xfs_mount;
|
|
|
|
/*
|
|
* structure for maintaining pre-calculated transaction reservations.
|
|
*/
|
|
struct xfs_trans_res {
|
|
uint tr_logres; /* log space unit in bytes per log ticket */
|
|
int tr_logcount; /* number of log operations per log ticket */
|
|
int tr_logflags; /* log flags, currently only used for indicating
|
|
* a reservation request is permanent or not */
|
|
};
|
|
|
|
struct xfs_trans_resv {
|
|
struct xfs_trans_res tr_write; /* extent alloc trans */
|
|
struct xfs_trans_res tr_itruncate; /* truncate trans */
|
|
struct xfs_trans_res tr_rename; /* rename trans */
|
|
struct xfs_trans_res tr_link; /* link trans */
|
|
struct xfs_trans_res tr_remove; /* unlink trans */
|
|
struct xfs_trans_res tr_symlink; /* symlink trans */
|
|
struct xfs_trans_res tr_create; /* create trans */
|
|
struct xfs_trans_res tr_create_tmpfile; /* create O_TMPFILE trans */
|
|
struct xfs_trans_res tr_mkdir; /* mkdir trans */
|
|
struct xfs_trans_res tr_ifree; /* inode free trans */
|
|
struct xfs_trans_res tr_ichange; /* inode update trans */
|
|
struct xfs_trans_res tr_growdata; /* fs data section grow trans */
|
|
struct xfs_trans_res tr_addafork; /* add inode attr fork trans */
|
|
struct xfs_trans_res tr_writeid; /* write setuid/setgid file */
|
|
struct xfs_trans_res tr_attrinval; /* attr fork buffer
|
|
* invalidation */
|
|
struct xfs_trans_res tr_attrsetm; /* set/create an attribute at
|
|
* mount time */
|
|
struct xfs_trans_res tr_attrsetrt; /* set/create an attribute at
|
|
* runtime */
|
|
struct xfs_trans_res tr_attrrm; /* remove an attribute */
|
|
struct xfs_trans_res tr_clearagi; /* clear agi unlinked bucket */
|
|
struct xfs_trans_res tr_growrtalloc; /* grow realtime allocations */
|
|
struct xfs_trans_res tr_growrtzero; /* grow realtime zeroing */
|
|
struct xfs_trans_res tr_growrtfree; /* grow realtime freeing */
|
|
struct xfs_trans_res tr_qm_setqlim; /* adjust quota limits */
|
|
struct xfs_trans_res tr_qm_dqalloc; /* allocate quota on disk */
|
|
struct xfs_trans_res tr_qm_quotaoff; /* turn quota off */
|
|
struct xfs_trans_res tr_qm_equotaoff;/* end of turn quota off */
|
|
struct xfs_trans_res tr_sb; /* modify superblock */
|
|
struct xfs_trans_res tr_fsyncts; /* update timestamps on fsync */
|
|
};
|
|
|
|
/* shorthand way of accessing reservation structure */
|
|
#define M_RES(mp) (&(mp)->m_resv)
|
|
|
|
/*
|
|
* Per-directory log reservation for any directory change.
|
|
* dir blocks: (1 btree block per level + data block + free block) * dblock size
|
|
* bmap btree: (levels + 2) * max depth * block size
|
|
* v2 directory blocks can be fragmented below the dirblksize down to the fsb
|
|
* size, so account for that in the DAENTER macros.
|
|
*/
|
|
#define XFS_DIROP_LOG_RES(mp) \
|
|
(XFS_FSB_TO_B(mp, XFS_DAENTER_BLOCKS(mp, XFS_DATA_FORK)) + \
|
|
(XFS_FSB_TO_B(mp, XFS_DAENTER_BMAPS(mp, XFS_DATA_FORK) + 1)))
|
|
#define XFS_DIROP_LOG_COUNT(mp) \
|
|
(XFS_DAENTER_BLOCKS(mp, XFS_DATA_FORK) + \
|
|
XFS_DAENTER_BMAPS(mp, XFS_DATA_FORK) + 1)
|
|
|
|
/*
|
|
* Various log count values.
|
|
*/
|
|
#define XFS_DEFAULT_LOG_COUNT 1
|
|
#define XFS_DEFAULT_PERM_LOG_COUNT 2
|
|
#define XFS_ITRUNCATE_LOG_COUNT 2
|
|
#define XFS_ITRUNCATE_LOG_COUNT_REFLINK 8
|
|
#define XFS_INACTIVE_LOG_COUNT 2
|
|
#define XFS_CREATE_LOG_COUNT 2
|
|
#define XFS_CREATE_TMPFILE_LOG_COUNT 2
|
|
#define XFS_MKDIR_LOG_COUNT 3
|
|
#define XFS_SYMLINK_LOG_COUNT 3
|
|
#define XFS_REMOVE_LOG_COUNT 2
|
|
#define XFS_LINK_LOG_COUNT 2
|
|
#define XFS_RENAME_LOG_COUNT 2
|
|
#define XFS_WRITE_LOG_COUNT 2
|
|
#define XFS_WRITE_LOG_COUNT_REFLINK 8
|
|
#define XFS_ADDAFORK_LOG_COUNT 2
|
|
#define XFS_ATTRINVAL_LOG_COUNT 1
|
|
#define XFS_ATTRSET_LOG_COUNT 3
|
|
#define XFS_ATTRRM_LOG_COUNT 3
|
|
|
|
void xfs_trans_resv_calc(struct xfs_mount *mp, struct xfs_trans_resv *resp);
|
|
uint xfs_allocfree_log_count(struct xfs_mount *mp, uint num_ops);
|
|
|
|
#endif /* __XFS_TRANS_RESV_H__ */
|