mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 01:41:39 +00:00
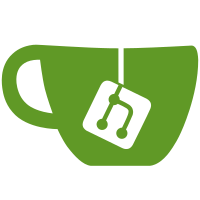
Commit670a5e356c
("thermal/core: Move the thermal zone lock out of the governors") moved thermal zone locking away from governors, but it forgot about the user space one which deadlocks now. Fix this by removing the thermal zone locking from the user space governor. Fixes:670a5e356c
("thermal/core: Move the thermal zone lock out of the governors") Tested-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com> Signed-off-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com>
56 lines
1.5 KiB
C
56 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* user_space.c - A simple user space Thermal events notifier
|
|
*
|
|
* Copyright (C) 2012 Intel Corp
|
|
* Copyright (C) 2012 Durgadoss R <durgadoss.r@intel.com>
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*/
|
|
|
|
#include <linux/slab.h>
|
|
#include <linux/thermal.h>
|
|
|
|
#include "thermal_core.h"
|
|
|
|
static int user_space_bind(struct thermal_zone_device *tz)
|
|
{
|
|
pr_info_once("Consider using thermal netlink events interface\n");
|
|
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* notify_user_space - Notifies user space about thermal events
|
|
* @tz: thermal_zone_device
|
|
* @trip: trip point index
|
|
*
|
|
* This function notifies the user space through UEvents.
|
|
*/
|
|
static int notify_user_space(struct thermal_zone_device *tz, int trip)
|
|
{
|
|
char *thermal_prop[5];
|
|
int i;
|
|
|
|
lockdep_assert_held(&tz->lock);
|
|
|
|
thermal_prop[0] = kasprintf(GFP_KERNEL, "NAME=%s", tz->type);
|
|
thermal_prop[1] = kasprintf(GFP_KERNEL, "TEMP=%d", tz->temperature);
|
|
thermal_prop[2] = kasprintf(GFP_KERNEL, "TRIP=%d", trip);
|
|
thermal_prop[3] = kasprintf(GFP_KERNEL, "EVENT=%d", tz->notify_event);
|
|
thermal_prop[4] = NULL;
|
|
kobject_uevent_env(&tz->device.kobj, KOBJ_CHANGE, thermal_prop);
|
|
for (i = 0; i < 4; ++i)
|
|
kfree(thermal_prop[i]);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct thermal_governor thermal_gov_user_space = {
|
|
.name = "user_space",
|
|
.throttle = notify_user_space,
|
|
.bind_to_tz = user_space_bind,
|
|
};
|
|
THERMAL_GOVERNOR_DECLARE(thermal_gov_user_space);
|