mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
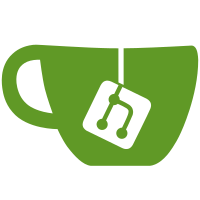
should_failslab() is a convenient function to hook into for directed error injection into kmalloc(). However, it is only available if a config flag is set. The following BCC script, for example, fails kmalloc() calls after a btrfs umount: from bcc import BPF prog = r""" BPF_HASH(flag); #include <linux/mm.h> int kprobe__btrfs_close_devices(void *ctx) { u64 key = 1; flag.update(&key, &key); return 0; } int kprobe__should_failslab(struct pt_regs *ctx) { u64 key = 1; u64 *res; res = flag.lookup(&key); if (res != 0) { bpf_override_return(ctx, -ENOMEM); } return 0; } """ b = BPF(text=prog) while 1: b.kprobe_poll() This patch refactors the should_failslab implementation so that the function is always available for error injection, independent of flags. This change would be similar in nature to commit f5490d3ec921 ("block: Add should_fail_bio() for bpf error injection"). Link: http://lkml.kernel.org/r/20180222020320.6944-1-hmclauchlan@fb.com Signed-off-by: Howard McLauchlan <hmclauchlan@fb.com> Reviewed-by: Andrew Morton <akpm@linux-foundation.org> Cc: Akinobu Mita <akinobu.mita@gmail.com> Cc: Christoph Lameter <cl@linux.com> Cc: Pekka Enberg <penberg@kernel.org> Cc: David Rientjes <rientjes@google.com> Cc: Joonsoo Kim <iamjoonsoo.kim@lge.com> Cc: Josef Bacik <jbacik@fb.com> Cc: Johannes Weiner <jweiner@fb.com> Cc: Alexei Starovoitov <ast@fb.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
67 lines
1.5 KiB
C
67 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/fault-inject.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/mm.h>
|
|
#include "slab.h"
|
|
|
|
static struct {
|
|
struct fault_attr attr;
|
|
bool ignore_gfp_reclaim;
|
|
bool cache_filter;
|
|
} failslab = {
|
|
.attr = FAULT_ATTR_INITIALIZER,
|
|
.ignore_gfp_reclaim = true,
|
|
.cache_filter = false,
|
|
};
|
|
|
|
bool __should_failslab(struct kmem_cache *s, gfp_t gfpflags)
|
|
{
|
|
/* No fault-injection for bootstrap cache */
|
|
if (unlikely(s == kmem_cache))
|
|
return false;
|
|
|
|
if (gfpflags & __GFP_NOFAIL)
|
|
return false;
|
|
|
|
if (failslab.ignore_gfp_reclaim && (gfpflags & __GFP_RECLAIM))
|
|
return false;
|
|
|
|
if (failslab.cache_filter && !(s->flags & SLAB_FAILSLAB))
|
|
return false;
|
|
|
|
return should_fail(&failslab.attr, s->object_size);
|
|
}
|
|
|
|
static int __init setup_failslab(char *str)
|
|
{
|
|
return setup_fault_attr(&failslab.attr, str);
|
|
}
|
|
__setup("failslab=", setup_failslab);
|
|
|
|
#ifdef CONFIG_FAULT_INJECTION_DEBUG_FS
|
|
static int __init failslab_debugfs_init(void)
|
|
{
|
|
struct dentry *dir;
|
|
umode_t mode = S_IFREG | S_IRUSR | S_IWUSR;
|
|
|
|
dir = fault_create_debugfs_attr("failslab", NULL, &failslab.attr);
|
|
if (IS_ERR(dir))
|
|
return PTR_ERR(dir);
|
|
|
|
if (!debugfs_create_bool("ignore-gfp-wait", mode, dir,
|
|
&failslab.ignore_gfp_reclaim))
|
|
goto fail;
|
|
if (!debugfs_create_bool("cache-filter", mode, dir,
|
|
&failslab.cache_filter))
|
|
goto fail;
|
|
|
|
return 0;
|
|
fail:
|
|
debugfs_remove_recursive(dir);
|
|
|
|
return -ENOMEM;
|
|
}
|
|
|
|
late_initcall(failslab_debugfs_init);
|
|
|
|
#endif /* CONFIG_FAULT_INJECTION_DEBUG_FS */
|