mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-03 07:38:10 +00:00
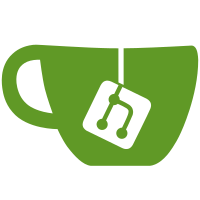
Add simple 1:1 wrappers of the C ioctl number manipulation functions. Since these are macros we cannot bindgen them directly, and since they should be usable in const context we cannot use helper wrappers, so we'll have to reimplement them in Rust. Thankfully, the C headers do declare defines for the relevant bitfield positions, so we don't need to duplicate that. Signed-off-by: Asahi Lina <lina@asahilina.net> Link: https://lore.kernel.org/r/20230329-rust-uapi-v2-2-bca5fb4d4a12@asahilina.net [ Moved the `#![allow(non_snake_case)]` to the usual place. ] Signed-off-by: Miguel Ojeda <ojeda@kernel.org>
103 lines
2.9 KiB
Rust
103 lines
2.9 KiB
Rust
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
//! The `kernel` crate.
|
|
//!
|
|
//! This crate contains the kernel APIs that have been ported or wrapped for
|
|
//! usage by Rust code in the kernel and is shared by all of them.
|
|
//!
|
|
//! In other words, all the rest of the Rust code in the kernel (e.g. kernel
|
|
//! modules written in Rust) depends on [`core`], [`alloc`] and this crate.
|
|
//!
|
|
//! If you need a kernel C API that is not ported or wrapped yet here, then
|
|
//! do so first instead of bypassing this crate.
|
|
|
|
#![no_std]
|
|
#![feature(allocator_api)]
|
|
#![feature(coerce_unsized)]
|
|
#![feature(core_ffi_c)]
|
|
#![feature(dispatch_from_dyn)]
|
|
#![feature(explicit_generic_args_with_impl_trait)]
|
|
#![feature(generic_associated_types)]
|
|
#![feature(new_uninit)]
|
|
#![feature(pin_macro)]
|
|
#![feature(receiver_trait)]
|
|
#![feature(unsize)]
|
|
|
|
// Ensure conditional compilation based on the kernel configuration works;
|
|
// otherwise we may silently break things like initcall handling.
|
|
#[cfg(not(CONFIG_RUST))]
|
|
compile_error!("Missing kernel configuration for conditional compilation");
|
|
|
|
// Allow proc-macros to refer to `::kernel` inside the `kernel` crate (this crate).
|
|
extern crate self as kernel;
|
|
|
|
#[cfg(not(test))]
|
|
#[cfg(not(testlib))]
|
|
mod allocator;
|
|
mod build_assert;
|
|
pub mod error;
|
|
pub mod init;
|
|
pub mod ioctl;
|
|
pub mod prelude;
|
|
pub mod print;
|
|
mod static_assert;
|
|
#[doc(hidden)]
|
|
pub mod std_vendor;
|
|
pub mod str;
|
|
pub mod sync;
|
|
pub mod task;
|
|
pub mod types;
|
|
|
|
#[doc(hidden)]
|
|
pub use bindings;
|
|
pub use macros;
|
|
pub use uapi;
|
|
|
|
#[doc(hidden)]
|
|
pub use build_error::build_error;
|
|
|
|
/// Prefix to appear before log messages printed from within the `kernel` crate.
|
|
const __LOG_PREFIX: &[u8] = b"rust_kernel\0";
|
|
|
|
/// The top level entrypoint to implementing a kernel module.
|
|
///
|
|
/// For any teardown or cleanup operations, your type may implement [`Drop`].
|
|
pub trait Module: Sized + Sync {
|
|
/// Called at module initialization time.
|
|
///
|
|
/// Use this method to perform whatever setup or registration your module
|
|
/// should do.
|
|
///
|
|
/// Equivalent to the `module_init` macro in the C API.
|
|
fn init(module: &'static ThisModule) -> error::Result<Self>;
|
|
}
|
|
|
|
/// Equivalent to `THIS_MODULE` in the C API.
|
|
///
|
|
/// C header: `include/linux/export.h`
|
|
pub struct ThisModule(*mut bindings::module);
|
|
|
|
// SAFETY: `THIS_MODULE` may be used from all threads within a module.
|
|
unsafe impl Sync for ThisModule {}
|
|
|
|
impl ThisModule {
|
|
/// Creates a [`ThisModule`] given the `THIS_MODULE` pointer.
|
|
///
|
|
/// # Safety
|
|
///
|
|
/// The pointer must be equal to the right `THIS_MODULE`.
|
|
pub const unsafe fn from_ptr(ptr: *mut bindings::module) -> ThisModule {
|
|
ThisModule(ptr)
|
|
}
|
|
}
|
|
|
|
#[cfg(not(any(testlib, test)))]
|
|
#[panic_handler]
|
|
fn panic(info: &core::panic::PanicInfo<'_>) -> ! {
|
|
pr_emerg!("{}\n", info);
|
|
// SAFETY: FFI call.
|
|
unsafe { bindings::BUG() };
|
|
// Bindgen currently does not recognize `__noreturn` so `BUG` returns `()`
|
|
// instead of `!`. See <https://github.com/rust-lang/rust-bindgen/issues/2094>.
|
|
loop {}
|
|
}
|