mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-13 14:14:37 +00:00
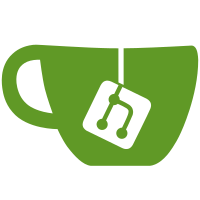
The results of "access_ok()" can be mis-speculated. The result is that you can end speculatively: if (access_ok(from, size)) // Right here even for bad from/size combinations. On first glance, it would be ideal to just add a speculation barrier to "access_ok()" so that its results can never be mis-speculated. But there are lots of system calls just doing access_ok() via "copy_to_user()" and friends (example: fstat() and friends). Those are generally not problematic because they do not _consume_ data from userspace other than the pointer. They are also very quick and common system calls that should not be needlessly slowed down. "copy_from_user()" on the other hand uses a user-controller pointer and is frequently followed up with code that might affect caches. Take something like this: if (!copy_from_user(&kernelvar, uptr, size)) do_something_with(kernelvar); If userspace passes in an evil 'uptr' that *actually* points to a kernel addresses, and then do_something_with() has cache (or other) side-effects, it could allow userspace to infer kernel data values. Add a barrier to the common copy_from_user() code to prevent mis-speculated values which happen after the copy. Also add a stub for architectures that do not define barrier_nospec(). This makes the macro usable in generic code. Since the barrier is now usable in generic code, the x86 #ifdef in the BPF code can also go away. Reported-by: Jordy Zomer <jordyzomer@google.com> Suggested-by: Linus Torvalds <torvalds@linuxfoundation.org> Signed-off-by: Dave Hansen <dave.hansen@linux.intel.com> Reviewed-by: Thomas Gleixner <tglx@linutronix.de> Acked-by: Daniel Borkmann <daniel@iogearbox.net> # BPF bits Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
74 lines
2.2 KiB
C
74 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
// Copyright(c) 2018 Linus Torvalds. All rights reserved.
|
|
// Copyright(c) 2018 Alexei Starovoitov. All rights reserved.
|
|
// Copyright(c) 2018 Intel Corporation. All rights reserved.
|
|
|
|
#ifndef _LINUX_NOSPEC_H
|
|
#define _LINUX_NOSPEC_H
|
|
|
|
#include <linux/compiler.h>
|
|
#include <asm/barrier.h>
|
|
|
|
struct task_struct;
|
|
|
|
#ifndef barrier_nospec
|
|
# define barrier_nospec() do { } while (0)
|
|
#endif
|
|
|
|
/**
|
|
* array_index_mask_nospec() - generate a ~0 mask when index < size, 0 otherwise
|
|
* @index: array element index
|
|
* @size: number of elements in array
|
|
*
|
|
* When @index is out of bounds (@index >= @size), the sign bit will be
|
|
* set. Extend the sign bit to all bits and invert, giving a result of
|
|
* zero for an out of bounds index, or ~0 if within bounds [0, @size).
|
|
*/
|
|
#ifndef array_index_mask_nospec
|
|
static inline unsigned long array_index_mask_nospec(unsigned long index,
|
|
unsigned long size)
|
|
{
|
|
/*
|
|
* Always calculate and emit the mask even if the compiler
|
|
* thinks the mask is not needed. The compiler does not take
|
|
* into account the value of @index under speculation.
|
|
*/
|
|
OPTIMIZER_HIDE_VAR(index);
|
|
return ~(long)(index | (size - 1UL - index)) >> (BITS_PER_LONG - 1);
|
|
}
|
|
#endif
|
|
|
|
/*
|
|
* array_index_nospec - sanitize an array index after a bounds check
|
|
*
|
|
* For a code sequence like:
|
|
*
|
|
* if (index < size) {
|
|
* index = array_index_nospec(index, size);
|
|
* val = array[index];
|
|
* }
|
|
*
|
|
* ...if the CPU speculates past the bounds check then
|
|
* array_index_nospec() will clamp the index within the range of [0,
|
|
* size).
|
|
*/
|
|
#define array_index_nospec(index, size) \
|
|
({ \
|
|
typeof(index) _i = (index); \
|
|
typeof(size) _s = (size); \
|
|
unsigned long _mask = array_index_mask_nospec(_i, _s); \
|
|
\
|
|
BUILD_BUG_ON(sizeof(_i) > sizeof(long)); \
|
|
BUILD_BUG_ON(sizeof(_s) > sizeof(long)); \
|
|
\
|
|
(typeof(_i)) (_i & _mask); \
|
|
})
|
|
|
|
/* Speculation control prctl */
|
|
int arch_prctl_spec_ctrl_get(struct task_struct *task, unsigned long which);
|
|
int arch_prctl_spec_ctrl_set(struct task_struct *task, unsigned long which,
|
|
unsigned long ctrl);
|
|
/* Speculation control for seccomp enforced mitigation */
|
|
void arch_seccomp_spec_mitigate(struct task_struct *task);
|
|
|
|
#endif /* _LINUX_NOSPEC_H */
|