mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-20 14:16:02 +00:00
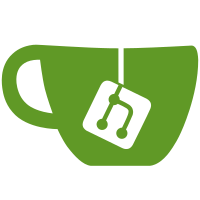
Before patchba92732e98
('perf kmaps: Check kmaps to make code more robust'), 'perf report' and 'perf annotate' will segfault if trace data contains kernel module information like this: # perf report -D -i ./perf.data ... 0 0 0x188 [0x50]: PERF_RECORD_MMAP -1/0: [0xffffffbff1018000(0xf068000) @ 0]: x [test_module] ... # perf report -i ./perf.data --objdump=/path/to/objdump --kallsyms=/path/to/kallsyms perf: Segmentation fault -------- backtrace -------- /path/to/perf[0x503478] /lib64/libc.so.6(+0x3545f)[0x7fb201f3745f] /path/to/perf[0x499b56] /path/to/perf(dso__load_kallsyms+0x13c)[0x49b56c] /path/to/perf(dso__load+0x72e)[0x49c21e] /path/to/perf(map__load+0x6e)[0x4ae9ee] /path/to/perf(thread__find_addr_map+0x24c)[0x47deec] /path/to/perf(perf_event__preprocess_sample+0x88)[0x47e238] /path/to/perf[0x43ad02] /path/to/perf[0x4b55bc] /path/to/perf(ordered_events__flush+0xca)[0x4b57ea] /path/to/perf[0x4b1a01] /path/to/perf(perf_session__process_events+0x3be)[0x4b428e] /path/to/perf(cmd_report+0xf11)[0x43bfc1] /path/to/perf[0x474702] /path/to/perf(main+0x5f5)[0x42de95] /lib64/libc.so.6(__libc_start_main+0xf4)[0x7fb201f23bd4] /path/to/perf[0x42dfc4] This is because __kmod_path__parse treats '[' leading names as kernel name instead of names of kernel module. If perf.data contains build information and the buildid of such modules can be found, the dso->kernel of it will be set to DSO_TYPE_KERNEL by __event_process_build_id(), not kernel module. It will then be passed to dso__load() -> dso__load_kernel_sym() -> dso__load_kcore() if --kallsyms is provided. The refered patch adds NULL pointer checker to avoid segfault. However, such kernel modules are still processed incorrectly. This patch fixes __kmod_path__parse, makes it treat names like '[test_module]' as kernel modules. kmod-path.c is also update to reflect the above changes. Signed-off-by: Wang Nan <wangnan0@huawei.com> Acked-by: Jiri Olsa <jolsa@kernel.org> Cc: Namhyung Kim <namhyung@kernel.org> Cc: Zefan Li <lizefan@huawei.com> Link: http://lkml.kernel.org/r/1433321541-170245-1-git-send-email-wangnan0@huawei.com [ Fixed the merged with0443f36b0d
("perf machine: Fix the search for the kernel DSO on the unified list" ] Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
145 lines
6.6 KiB
C
145 lines
6.6 KiB
C
#include <stdbool.h>
|
|
#include "tests.h"
|
|
#include "dso.h"
|
|
#include "debug.h"
|
|
|
|
static int test(const char *path, bool alloc_name, bool alloc_ext,
|
|
bool kmod, bool comp, const char *name, const char *ext)
|
|
{
|
|
struct kmod_path m;
|
|
|
|
memset(&m, 0x0, sizeof(m));
|
|
|
|
TEST_ASSERT_VAL("kmod_path__parse",
|
|
!__kmod_path__parse(&m, path, alloc_name, alloc_ext));
|
|
|
|
pr_debug("%s - alloc name %d, alloc ext %d, kmod %d, comp %d, name '%s', ext '%s'\n",
|
|
path, alloc_name, alloc_ext, m.kmod, m.comp, m.name, m.ext);
|
|
|
|
TEST_ASSERT_VAL("wrong kmod", m.kmod == kmod);
|
|
TEST_ASSERT_VAL("wrong comp", m.comp == comp);
|
|
|
|
if (ext)
|
|
TEST_ASSERT_VAL("wrong ext", m.ext && !strcmp(ext, m.ext));
|
|
else
|
|
TEST_ASSERT_VAL("wrong ext", !m.ext);
|
|
|
|
if (name)
|
|
TEST_ASSERT_VAL("wrong name", m.name && !strcmp(name, m.name));
|
|
else
|
|
TEST_ASSERT_VAL("wrong name", !m.name);
|
|
|
|
free(m.name);
|
|
free(m.ext);
|
|
return 0;
|
|
}
|
|
|
|
static int test_is_kernel_module(const char *path, int cpumode, bool expect)
|
|
{
|
|
TEST_ASSERT_VAL("is_kernel_module",
|
|
(!!is_kernel_module(path, cpumode)) == (!!expect));
|
|
pr_debug("%s (cpumode: %d) - is_kernel_module: %s\n",
|
|
path, cpumode, expect ? "true" : "false");
|
|
return 0;
|
|
}
|
|
|
|
#define T(path, an, ae, k, c, n, e) \
|
|
TEST_ASSERT_VAL("failed", !test(path, an, ae, k, c, n, e))
|
|
|
|
#define M(path, c, e) \
|
|
TEST_ASSERT_VAL("failed", !test_is_kernel_module(path, c, e))
|
|
|
|
int test__kmod_path__parse(void)
|
|
{
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("/xxxx/xxxx/x-x.ko", true , true , true, false, "[x_x]", NULL);
|
|
T("/xxxx/xxxx/x-x.ko", false , true , true, false, NULL , NULL);
|
|
T("/xxxx/xxxx/x-x.ko", true , false , true, false, "[x_x]", NULL);
|
|
T("/xxxx/xxxx/x-x.ko", false , false , true, false, NULL , NULL);
|
|
M("/xxxx/xxxx/x-x.ko", PERF_RECORD_MISC_CPUMODE_UNKNOWN, true);
|
|
M("/xxxx/xxxx/x-x.ko", PERF_RECORD_MISC_KERNEL, true);
|
|
M("/xxxx/xxxx/x-x.ko", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("/xxxx/xxxx/x.ko.gz", true , true , true, true, "[x]", "gz");
|
|
T("/xxxx/xxxx/x.ko.gz", false , true , true, true, NULL , "gz");
|
|
T("/xxxx/xxxx/x.ko.gz", true , false , true, true, "[x]", NULL);
|
|
T("/xxxx/xxxx/x.ko.gz", false , false , true, true, NULL , NULL);
|
|
M("/xxxx/xxxx/x.ko.gz", PERF_RECORD_MISC_CPUMODE_UNKNOWN, true);
|
|
M("/xxxx/xxxx/x.ko.gz", PERF_RECORD_MISC_KERNEL, true);
|
|
M("/xxxx/xxxx/x.ko.gz", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("/xxxx/xxxx/x.gz", true , true , false, true, "x.gz" ,"gz");
|
|
T("/xxxx/xxxx/x.gz", false , true , false, true, NULL ,"gz");
|
|
T("/xxxx/xxxx/x.gz", true , false , false, true, "x.gz" , NULL);
|
|
T("/xxxx/xxxx/x.gz", false , false , false, true, NULL , NULL);
|
|
M("/xxxx/xxxx/x.gz", PERF_RECORD_MISC_CPUMODE_UNKNOWN, false);
|
|
M("/xxxx/xxxx/x.gz", PERF_RECORD_MISC_KERNEL, false);
|
|
M("/xxxx/xxxx/x.gz", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("x.gz", true , true , false, true, "x.gz", "gz");
|
|
T("x.gz", false , true , false, true, NULL , "gz");
|
|
T("x.gz", true , false , false, true, "x.gz", NULL);
|
|
T("x.gz", false , false , false, true, NULL , NULL);
|
|
M("x.gz", PERF_RECORD_MISC_CPUMODE_UNKNOWN, false);
|
|
M("x.gz", PERF_RECORD_MISC_KERNEL, false);
|
|
M("x.gz", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("x.ko.gz", true , true , true, true, "[x]", "gz");
|
|
T("x.ko.gz", false , true , true, true, NULL , "gz");
|
|
T("x.ko.gz", true , false , true, true, "[x]", NULL);
|
|
T("x.ko.gz", false , false , true, true, NULL , NULL);
|
|
M("x.ko.gz", PERF_RECORD_MISC_CPUMODE_UNKNOWN, true);
|
|
M("x.ko.gz", PERF_RECORD_MISC_KERNEL, true);
|
|
M("x.ko.gz", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("[test_module]", true , true , true, false, "[test_module]", NULL);
|
|
T("[test_module]", false , true , true, false, NULL , NULL);
|
|
T("[test_module]", true , false , true, false, "[test_module]", NULL);
|
|
T("[test_module]", false , false , true, false, NULL , NULL);
|
|
M("[test_module]", PERF_RECORD_MISC_CPUMODE_UNKNOWN, true);
|
|
M("[test_module]", PERF_RECORD_MISC_KERNEL, true);
|
|
M("[test_module]", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("[test.module]", true , true , true, false, "[test.module]", NULL);
|
|
T("[test.module]", false , true , true, false, NULL , NULL);
|
|
T("[test.module]", true , false , true, false, "[test.module]", NULL);
|
|
T("[test.module]", false , false , true, false, NULL , NULL);
|
|
M("[test.module]", PERF_RECORD_MISC_CPUMODE_UNKNOWN, true);
|
|
M("[test.module]", PERF_RECORD_MISC_KERNEL, true);
|
|
M("[test.module]", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("[vdso]", true , true , false, false, "[vdso]", NULL);
|
|
T("[vdso]", false , true , false, false, NULL , NULL);
|
|
T("[vdso]", true , false , false, false, "[vdso]", NULL);
|
|
T("[vdso]", false , false , false, false, NULL , NULL);
|
|
M("[vdso]", PERF_RECORD_MISC_CPUMODE_UNKNOWN, false);
|
|
M("[vdso]", PERF_RECORD_MISC_KERNEL, false);
|
|
M("[vdso]", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("[vsyscall]", true , true , false, false, "[vsyscall]", NULL);
|
|
T("[vsyscall]", false , true , false, false, NULL , NULL);
|
|
T("[vsyscall]", true , false , false, false, "[vsyscall]", NULL);
|
|
T("[vsyscall]", false , false , false, false, NULL , NULL);
|
|
M("[vsyscall]", PERF_RECORD_MISC_CPUMODE_UNKNOWN, false);
|
|
M("[vsyscall]", PERF_RECORD_MISC_KERNEL, false);
|
|
M("[vsyscall]", PERF_RECORD_MISC_USER, false);
|
|
|
|
/* path alloc_name alloc_ext kmod comp name ext */
|
|
T("[kernel.kallsyms]", true , true , false, false, "[kernel.kallsyms]", NULL);
|
|
T("[kernel.kallsyms]", false , true , false, false, NULL , NULL);
|
|
T("[kernel.kallsyms]", true , false , false, false, "[kernel.kallsyms]", NULL);
|
|
T("[kernel.kallsyms]", false , false , false, false, NULL , NULL);
|
|
M("[kernel.kallsyms]", PERF_RECORD_MISC_CPUMODE_UNKNOWN, false);
|
|
M("[kernel.kallsyms]", PERF_RECORD_MISC_KERNEL, false);
|
|
M("[kernel.kallsyms]", PERF_RECORD_MISC_USER, false);
|
|
|
|
return 0;
|
|
}
|